MongoDB is a document-based database management system. It is schemaless by nature, so the same collection can hold data of different properties/schemas/structures.
The name is MongoDB is taken from the word “HUMONGOUS“, which means huge. This is because- MongoDB is built to store and handle a huge amount of data.
Hierarchy of data storing in a MongoDB database is in 3 areas-
- Database: Main/Largest entity where all the data of an application/group lives.
- Collection: Contains a certain type of data. Can hold multiple sets of data/documents and lives in a database. There can be multiple collections in a database. A collection can be compared to a table of a relational database management system.
- Document: Contains data of a single item. Can hold multiple fields/properties that are required for representing an item. A collection will contain multiple documents. A document can be referred to as a row of a RDBMS.
So the hierarchy is: Database > Collection > Document
A MongoDB server can have multiple databases in it.
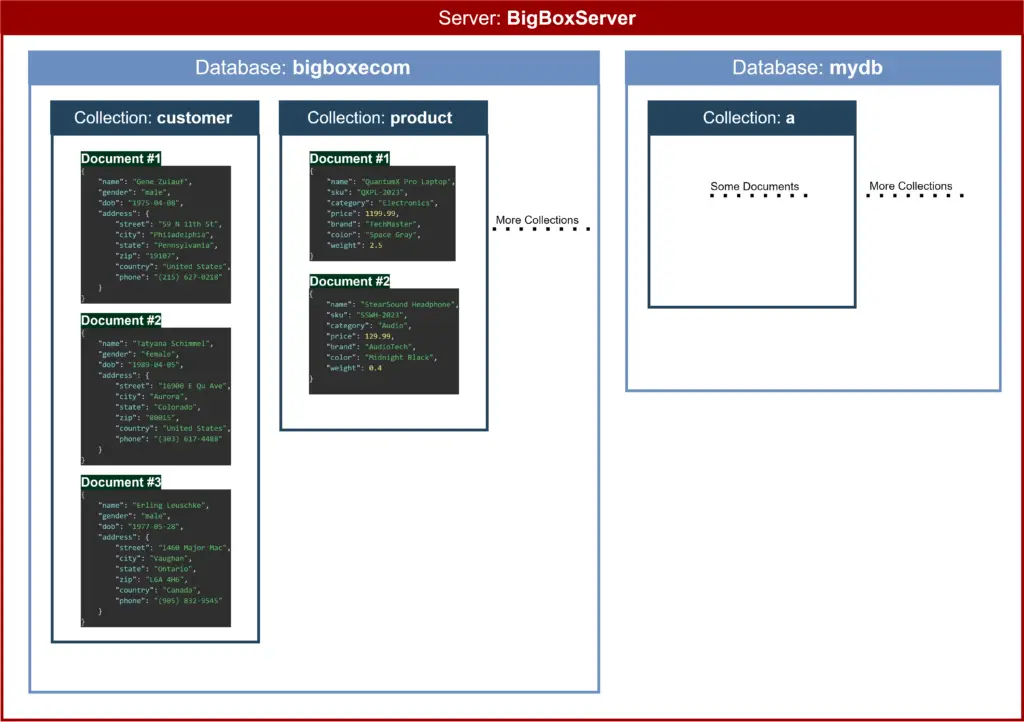
Key Features
- MongoDB is schemaless, which makes it flexible to adapt to the changing application needs. Though there are some advantages of following a schema, but when our application is evolving, if we use the flexibility of a scheme it will be very easy to use.
- Instead of distributing data into multiple tables, in MongoDB, we can embed related information into the same document. This way we can save some operations(like JOIN), as all data stays together.
- MongoDB is suitable for reading and writing heavy applications because of the performance it provides.
Basic Commands
Purpose | Command | Notes |
---|---|---|
Show the list of all databases on the server |
| Alternative command that serves the same purpose-
|
Select a specific database to start using |
| Here “bigboxecom” is the name of the database. We can switch to a database that does not exist yet. Switching to a database that does not exist, will not create the database right then. The database will be created when we start adding some data to it. |
Get the list of all collections of the database |
| This only give the list of collections of the database which is being used(with the use command). |
Get the name of the database that is being used currently |
| This will print the name of the database that is being used currently. |
Installation & Management
Data Types
Data Modeling
Create/Insert Operations
- db.collection.insertOne()
- db.collection.insertMany()
- db.collection.bulkWrite()
Read/Find Operations
- db.collection.find()
- db.collection.fineOne()
- db.collection.findAndModify()
- db.collection.findOneAndUpdate()
- db.collection.findOneAndReplace()
- db.collection.findOneAndDelete()
- db.collection.distinct()
- db.collection.count()
- db.collection.countDocuments()
- db.collection.estimatedDocumentCount()
Update Operations
- db.collection.updateOne()
- db.collection.updateMany()
- db.collection.replaceOne()
Delete Operations
- db.collection.deleteOne()
- db.collection.deleteMany()
Indexing
- db.collection.createIndex()
- db.collection.createIndexes()
- db.collection.getIndexes()
- db.collection.dropIndex()
- db.collection.dropIndexes()
- db.collection.hideIndex()
- db.collection.unhideIndex()
- db.collection.reIndex()
- db.collection.totalIndexSize()
Aggregation
Collection Management/Stat Operations
- db.collection.dataSize()
- db.collection.storageSize()
- db.collection.totalIndexSize()
- db.collection.totalSize()
- db.collection.drop()
- db.collection.explain()
- db.collection.isCapped()
- db.collection.latencyStats()
- db.collection.renameCollection()
- db.collection.stats()
- db.collection.validate()
Database Operations
- db.adminCommand()
- db.aggregate()
- db.commandHelp()
- db.createCollection()
- db.createView()
- db.currentOp()
- db.dropDatabase()
- db.fsyncLock()
- db.fsyncUnlock()
- db.getCollection()
- db.getCollectionInfos()
- db.getCollectionNames()
- db.getLogComponents()
- db.getMongo()
- db.getName()
- db.getProfilingStatus()
- db.getReplicationInfo()
- db.getSiblingDB()
- db.hello()
- db.help()
- db.hostInfo()
- db.killOp()
- db.listCommands()
- db.logout()
- db.printCollectionStats()
- db.printReplicationInfo()
- db.printSecondaryReplicationInfo()
- db.printShardingStatus()
- db.printSlaveReplicationInfo()
- db.resetError()
- db.rotateCertificates()
- db.runCommand()
- db.serverBuildInfo()
- db.serverCmdLineOpts()
- db.serverStatus()
- db.setLogLevel()
- db.setProfilingLevel()
- db.shutdownServer()
- db.stats()
- db.version()
- db.watch()
Bulk Operations
- db.collection.initializeOrderedBulkOp()
- db.collection.initializeUnorderedBulkOp()
- Bulk()
- Bulk.execute()
- Bulk.find()
- Bulk.find.arrayFilters()
- Bulk.find.collation()
- Bulk.find.hint()
- Bulk.find.remove()
- Bulk.find.removeOne()
- Bulk.find.replaceOne()
- Bulk.find.updateOne()
- Bulk.find.update()
- Bulk.find.upsert()
- Bulk.getOperations()
- Bulk.insert()
- Bulk.toJSON()
- Bulk.toString()
Query Plan Cache Operations
- db.collection.getPlanCache()
- PlanCache.clear()
- PlanCache.clearPlansByQuery()
- PlanCache.help()
- PlanCache.list()
Connection Operations
- connect()
- Mongo()
- Mongo.getDB()
- Mongo.getDBNames()
- Mongo.getDBs()
- Mongo.getReadPrefMode()
- Mongo.getReadPrefTagSet()
- Mongo.getWriteConcern()
- Mongo.setCausalConsistency()
- Mongo.setReadPref()
- Mongo.startSession()
- Mongo.setWriteConcern()
- Mongo.watch()
- Session.abortTransaction()
- Session.commitTransaction()
- Session.startTransaction()
- Session.withTransaction()
Security
- User Management Operations
- db.auth()
- db.changeUserPassword()
- db.createUser()
- db.dropUser()
- db.dropAllUsers()
- db.getUser()
- db.getUsers()
- db.grantRolesToUser()
- db.removeUser()
- db.revokeRolesFromUser()
- db.updateUser()
- passwordPrompt()
- Role Management Operations
- db.createRole()
- db.dropRole()
- db.dropAllRoles()
- db.getRole()
- db.getRoles()
- db.grantPrivilegesToRole()
- db.revokePrivilegesFromRole()
- db.grantRolesToRole()
- db.revokeRolesFromRole()
- db.updateRole()
- Client-Side Encryption Operations
- getKeyVault()
- KeyVault.createKey()
- KeyVault.deleteKey()
- KeyVault.getKey()
- KeyVault.getKeys()
- KeyVault.addKeyAlternateName()
- KeyVault.removeKeyAlternateName()
- KeyVault.getKeyByAltName()
- KeyVault.rewrapManyDataKey()
- getClientEncryption()
- ClientEncryption.createEncryptedCollection()
- ClientEncryption.encrypt()
- ClientEncryption.decrypt()
Cursor Operations
- cursor.addOption()
- cursor.allowDiskUse()
- cursor.allowPartialResults()
- cursor.batchSize()
- cursor.close()
- cursor.isClosed()
- cursor.collation()
- cursor.comment()
- cursor.count()
- cursor.explain()
- cursor.forEach()
- cursor.hasNext()
- cursor.hint()
- cursor.isExhausted()
- cursor.itcount()
- cursor.limit()
- cursor.map()
- cursor.max()
- cursor.maxAwaitTimeMS()
- cursor.maxTimeMS()
- cursor.min()
- cursor.next()
- cursor.noCursorTimeout()
- cursor.objsLeftInBatch()
- cursor.pretty()
- cursor.readConcern()
- cursor.readPref()
- cursor.returnKey()
- cursor.showRecordId()
- cursor.size()
- cursor.skip()
- cursor.sort()
- cursor.tailable()
- cursor.toArray()
- cursor.tryNext()
Object Constructor Operations
- Binary.createFromBase64()
- Binary.createFromHexString()
- BinData()
- BulkWriteResult()
- Date() and Datetime
- ObjectId()
- ObjectId.createFromBase64()
- ObjectId.createFromHexString()
- ObjectId.getTimestamp()
- ObjectId.toString()
- ObjectId.valueOf()
- UUID()
- WriteResult()
- WriteResult.hasWriteError()
- WriteResult.hasWriteConcernError()
Replication
- rs.add()
- rs.addArb()
- rs.conf()
- rs.freeze()
- rs.help()
- rs.initiate()
- rs.printReplicationInfo()
- rs.printSecondaryReplicationInfo()
- rs.reconfig()
- rs.reconfigForPSASet()
- rs.remove()
- rs.status()
- rs.stepDown()
- rs.syncFrom()
Sharding
- convertShardKeyToHashed
- db.checkMetadataConsistency()
- db.collection.checkMetadataConsistency()
- db.collection.analyzeShardKey()
- db.collection.configureQueryAnalyzer()
- db.collection.getShardDistribution()
- db.collection.getSharedVersion()
- db.collection.watch()
- sh.abortRehashedCollection()
- sh.addShard()
- sh.addShardTag()
- sh.addShardToZone()
- sh.addTagRange()
- sh.balancerCollectionStatus()
- sh.checkMetadataConsistency()
- sh.commitReshardCollection()
- sh.disableAutoMerger()
- sh.disableBalancing()
- sh.enableAutoMerger()
- sh.enableBalancing()
- sh.disableAutoSplit()
- sh.enableAutoSplit()
- sh.enableSharding()
- sh.getBalancerState()
- sh.help()
- sh.isBalancerRunning()
- sh.moveChunk()
- sh.removeRangeFromZone()
- sh.removeTagRange()
- sh.reshardCollection()
- sh.setBalancerState()
- sh.shardCollection()
- sh.splitAt()
- sh.splitFind()
- sh.startAutoMerger()
- sh.startBalancer()
- sh.status()
- sh.stopAutoMerger()
- sh.stopBalancer()
- sh.waitForBalancer()
- sh.waitForPingChange()
- sh.pdateZoneKeyRange()