Shell is like a container, that accepts commands from the user/other programs and sends them to the kernel after parsing and processing.
Shell
In simple words, Shell is an application that takes input from the keyboard, and passes that to the kernel for processing.
If you want to check available shells on your Linux OS, check the “/etc/shells” file.
$ cat /etc/shells
# /etc/shells: valid login shells
/bin/sh
/bin/bash
/usr/bin/bash
/bin/rbash
/usr/bin/rbash
/usr/bin/sh
/bin/dash
/usr/bin/dash
/usr/bin/tmux
/usr/bin/screen
BashHere we can see a list of all available shells.
To check which shell you are using right now, use the following command-
# Check shell that is currently running
$ echo $0
-bash
# Check the default shell assigned to this user
$ echo $SHELL
/bin/bash
# Check the process the currently running shell
$ ps -p $$
PID TTY TIME CMD
55782 pts/10 00:00:00 bash
BashYou can also check the “/etc/passwd” file. In the file, you can check which shell is defined by default for your user name.
$ cat /etc/passwd
root:x:0:0:root:/root:/bin/bash
bigboxcode:x:1000:1000:,,,:/home/bigboxcode:/bin/bash
redis:x:108:118::/var/lib/redis:/usr/sbin/nologin
BashIf you want to change the default shell by making changes in this file.
Bash
The original Linux shell was written(developed) by Steve Bourne.
Bash is an acronym of- bourne-again shell
Bash is an enhanced version of shell(sh).
Kernel
Kernel is the interface between hardware and software. Kernel stays on top of the hardware as a layer, takes every command provided by Shell, and executes that on the hardware.
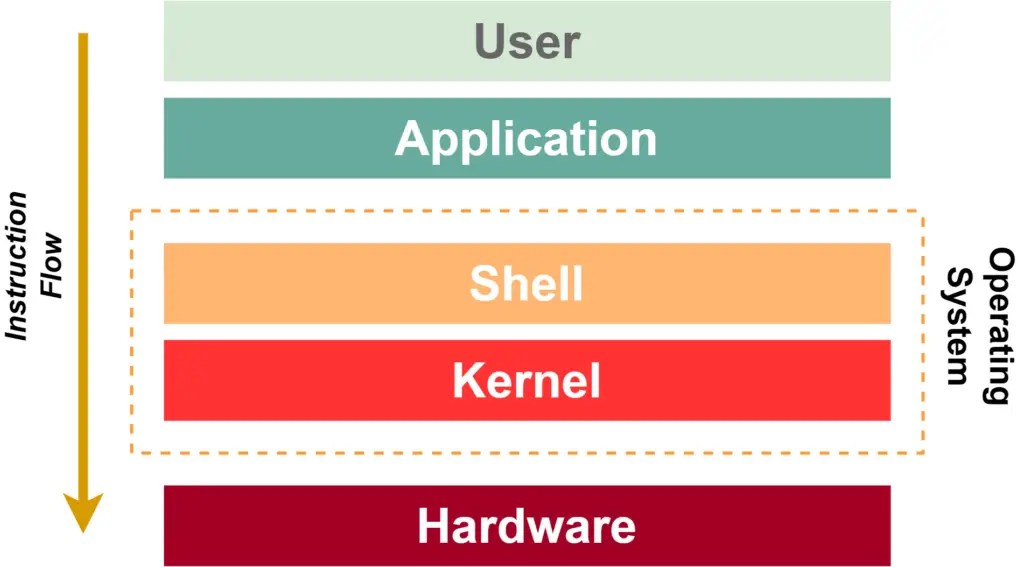
Shell Script Permission
Script files should have proper permission for execution. Give them the script execution permission by using the following command-
$ chmod a+x name_of_the_script
BashDenote Shell
At the beginning of the script, we have to denote the shell, for which this script is written for.
For all our scripts we will write for “sh”. So we will write the following line at the beging of every script-
#!/bin/sh
ShellScriptHello World
Let’s start with a simple example of print “hello world”. We can use “echo” to output the “Hello world” on the script.
#!/bin/sh
echo Hello World
ShellScriptSave the file as “script1.sh” and then run it, you will get output as below-
$ ./script1.sh
Hello World
Bashecho
Use the echo command to print something on the screen. We can also use “echo” without any param/string to print empty line-
#!/bin/sh
echo
echo Hello World
echo
ShellScriptRun the script and you will see the output like below-
$ ./script1.sh
Hello World
BashVariable
Declaring a variable is very simple. Just write the name of the variable, then the equal sign and then the value.
name=bigboxcode
url="https://bigboxcode.com"
description="A long description of the site"
show_list="ls -la"
ShellScriptTo use the variable use a dollar sign($) before the variable name.
Like, if we want to print the values then we can do it like below-
name=bigboxcode
url="https://bigboxcode.com"
description="A long description of the site"
show_list="ls -la"
echo Name: $name
echo $url
echo $description
echo $show_list
ShellScriptTo use the variable value as a command, like we have “show_list” here, just add the dollar sign($) before the variable name and put it in a new line-
show_list="ls -la"
$show_list
ShellScriptPrint Command Output
If we want to print the output of a command then we have to apply a specific format for that.
First, let’s see what happens if we just put the command directly in the “echo” command-
hn1=hostname
echo Host name: $hn1
echo Host name: hostname
ShellScriptOutput:
Host name: hostname
Host name: hostname
PlaintextTo execute the command “hostname” we have to wrap it in backtick(`).
hn2=`hostname`
echo Host name: $hn2
echo Host name: `hostname`
ShellScriptOutput:
Host name: bigboxcode
Host name: bigboxcode
PlaintextWe can do the same thing with other commands also-
echo List of files: `ls`
echo List of files: `ls -l`
ShellScriptOutput:
List of files: script1.sh script2.sh script3.sh
List of files:
total 12
-rwxr-xr-x 1 bigboxcode bigboxcode 38 Jul 22 12:38 script1.sh
-rwxr-xr-x 1 bigboxcode bigboxcode 193 Jul 22 13:41 script2.sh
-rwxr-xr-x 1 bigboxcode bigboxcode 192 Jul 22 14:03 script3.sh
PlaintextRead Input
Use the command “read” to get input from the user and save it in a variable. Later we can use that variable as a normal variable-
echo Enter your name here:
read name
echo Enter age:
read age
echo Hello, $name
echo Your age is: $age
BashOutput:
Enter your name here:
Big Box Code
Enter age:
18
Hello, Big Box Code
Your age is: 18
Plaintextif-else Condition
We can write an if-else condition in the script.
Compare Values
If you want to check if something is equal to some value use “-eq”.
mark=100
if [ $mark -eq 100 ]
then
echo In Then
echo Mark is 100
else
echo In Else
echo Mark is not 100
fi
BashOutput:
In Then
Mark is 100
PlaintextWe can also take mark as input from the user and compare it-
echo Enter Mark:
read mark
if [ $mark -eq 100 ]
then
echo In Then
echo Mark is 100
else
echo In Else
echo Mark is not 100
fi
BashOutput:
Enter Mark:
30
In Else
Mark is not 100
PlaintextHere are some more comparison examples. Here we are checking the quality of the mark. We are checking if the mark is greater than(-gt) and greater than or equal(-ge).
echo Enter mark:
read mark
if [ $mark -gt 100 ]; then
echo Invlaid number
elif [ $mark -ge 90 ]; then
echo Excellent
elif [ $mark -ge 80 ]; then
echo Good
elif [ $mark -ge 60 ]; then
echo OK
else
echo Not Good
fi
BashOutput:
Run the script and enter some values. Here we have entered 80.
Enter mark:
80
Good
PlaintextCheck File Existence
Let’s see how we can check if a file exists. Write a script to check if the file “crash.log” exists or not-
if [ -e ./crash.log ]
then
echo file exists
else
echo file does not exist
fi
BashOutput:
file does not exist
PlaintextNow create file named “crash.log”.
touch crash.log
BashAnd run the script again-
Output:
file exists
Plaintextcase Statement
Start a case statement with “case” and end with “esac”. To ensure default option(when none of the options match) use asterisk(*). Use pipe(|) to use multiple options as “or”.
echo Enter option:
read option
case $option in
a) echo You pressed a;;
b|c|d) echo you pressed one of b or c or d;;
*) echo none of the option matches;;
esac
BashOutput:
Enter option:
c
you pressed one of b or c or d
Enter option:
sdfd
none of the option matches
Plaintextfor Loop
for superhero in bat super spider iron snow
do
echo Name of a superhero: ${superhero}man
done
BashSpecify the end of the for loop with “done”.
Output:
Name of a superhero: batman
Name of a superhero: superman
Name of a superhero: spiderman
Name of a superhero: ironman
Name of a superhero: snowman
Plaintextwhile Loop
count=0
while [ $count -lt 10 ]
do
echo $count
sleep 1
count=`expr $count + 1`
done
BashOutput:
0
1
2
3
4
5
6
7
8
9
PlaintextExist Status
0 = OK or successful
1 = Minor problem
2 = Serious trouble
3-255 = Everything else
#!/bin/sh
ls -l ./crash.log
# Check status code of the last command execution by $?
if [ $? -eq 0 ]; then
echo File found in the system
else
echo File not found
fi
BashOutput:
-rw-r--r-- 1 bigboxcode bigboxcode 0 Jul 22 16:26 ./crash.log
File found in the system
Plaintext