Hash table data structure is used to store key-value data pairs. Main benefit of using a Hash Table is the performance improvements for set and get operation of data.
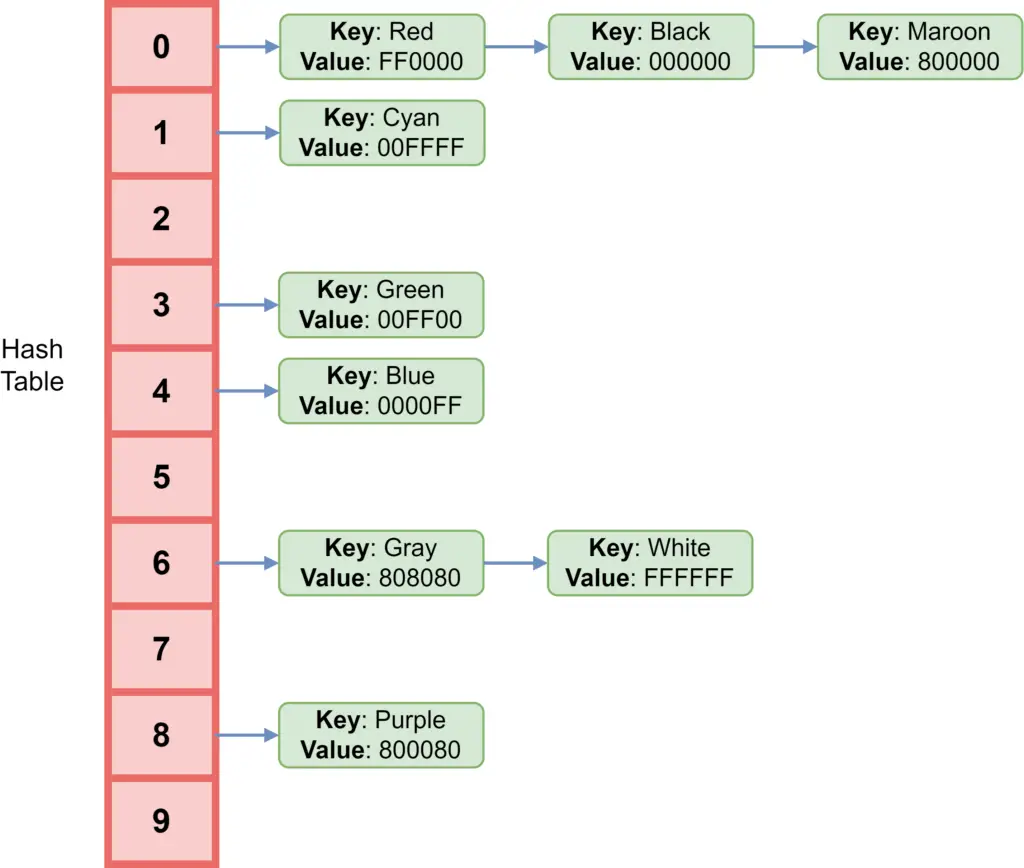
NOTE
In this article, we are discussing the implementation of Hash Table in JavaScript and TypeScript.
We are using the “Separate Chaining” method here, to store data if the hash function returns the same value for multiple dataset.
Step #1: Hash Table Class
Here is how the stored data would look like when stored in array-
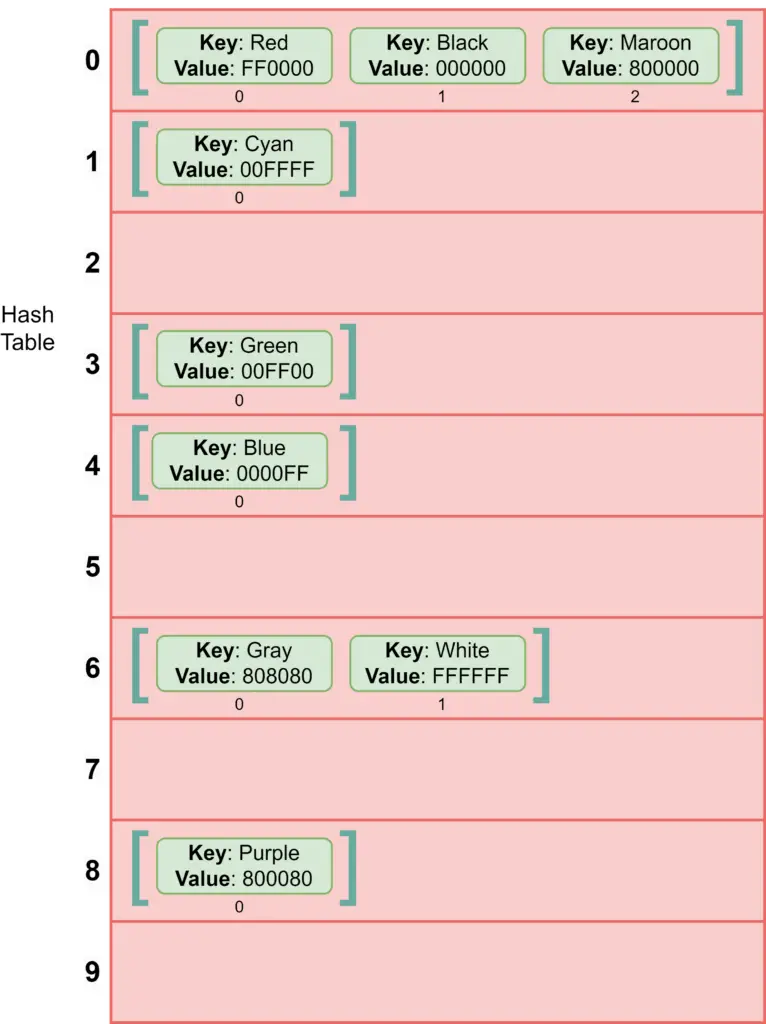
Step #2: Hashing function
Here we are using a hash function for demo purposes. This hash function can vary depending on your implementation.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step #3: Set data in the Hash Table
This function is used to set key-value pair in the dataset.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step #4: Get data from the Hash Table
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step #5: Get all Keys
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step #6: Get all Values
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Full Source Code
Here is the full source code of Hash Table implementation-
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Demo
Use the following code to check the implementation-
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Output:
Here is the output of above demo code-
---------- Hash Table - Set example -----------
[
[ { key: 'Yellow', value: 'FFFF00' } ],
<1 empty item>,
[ { key: 'Indigo', value: '4B0082' } ],
<1 empty item>,
[
{ key: 'Brown', value: 'A52A2A' },
{ key: 'Orange', value: 'FFA500' }
],
[
{ key: 'Green', value: '00FF00' },
{ key: 'Magenta', value: 'FF00FF' },
{ key: 'Turquoise', value: '40E0D0' }
],
[
{ key: 'Pink', value: 'FFC0CB' },
{ key: 'Teal', value: '008080' },
{ key: 'Navy', value: '000080' }
],
[
{ key: 'Red', value: 'FF0000' },
{ key: 'Gray', value: '808080' },
{ key: 'Lime', value: '00FF00' },
{ key: 'Olive', value: '808000' }
],
[
{ key: 'Blue', value: '0000FF' },
{ key: 'Purple', value: '800080' },
{ key: 'Maroon', value: '800000' }
],
[
{ key: 'Black', value: '000000' },
{ key: 'White', value: 'FFFFFF' }
],
<1 empty item>,
[ { key: 'Cyan', value: '00FFFF' } ]
]
---------- Hash Table - Get example -----------
Find 'Pink' in hash table: { key: 'Pink', value: 'FFC0CB' }
Find 'Black' in hash table: { key: 'Black', value: '000000' }
Find 'Blue' in hash table: { key: 'Blue', value: '0000FF' }
Find 'Green' in hash table: { key: 'Green', value: '00FF00' }
Find 'Maroon' in hash table: { key: 'Maroon', value: '800000' }
Find 'Unknown or wrong color name' in hash table: undefined
---------- Hash Table - Get all keys example -----------
All keys: [
'Yellow', 'Indigo',
'Brown', 'Orange',
'Green', 'Magenta',
'Turquoise', 'Pink',
'Teal', 'Navy',
'Red', 'Gray',
'Lime', 'Olive',
'Blue', 'Purple',
'Maroon', 'Black',
'White', 'Cyan'
]
---------- Hash Table - Get all values example -----------
All values: [
'FFFF00', '4B0082', 'A52A2A',
'FFA500', '00FF00', 'FF00FF',
'40E0D0', 'FFC0CB', '008080',
'000080', 'FF0000', '808080',
'00FF00', '808000', '0000FF',
'800080', '800000', '000000',
'FFFFFF', '00FFFF'
]
Testing
Here is the test cases for the Hash table implementation-
Tests are implemented with ‘vitest’.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Time Complexity
Operation | Complexity |
---|---|
Insert | O(1) |
Delete | O(1) |
Access/Get | O(1) |
Source Code
Use the following links to get the source code used in this article-
Source Code of | Implementation Code | Demo Code | Test Code |
---|---|---|---|
JavaScript Implementation | ![]() | ![]() | ![]() |
TypeScript Implementation | ![]() | ![]() | ![]() |
Related Data Structures
Command | Details |
---|---|
Binary Heap | ![]() |