Summary
Data Structure Name | Stack |
Type | Linear Data Structure |
Tagline | LIFO – Last In First Out |
Operations | Push Pop |
Use cases | |
Implementations | ![]() ![]() ![]() ![]() ![]() |
Definition
Stack is a data structure that has only one end for performing operations. All operations can be performed at the head, be it adding(pushing) element or removing(popping) element.
Stack follows the LIFO – Last In First Out principle, so the item which is inserted at the last, is removed(popped) first.
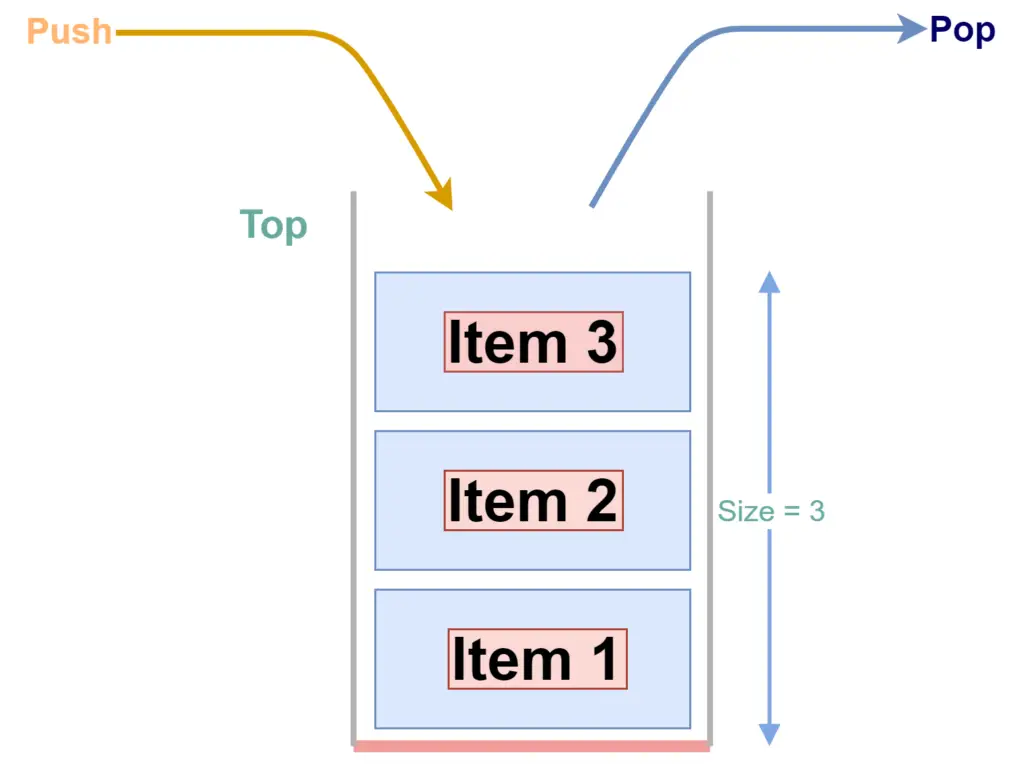
Use Cases
Characteristics
Elements
A Stack data structure has the following info-
- Head
- Tail
- Length
NOTES
Types
Functionality
Code Implementations
Use the following links to check Stack data structure implementation in specific programming languages.