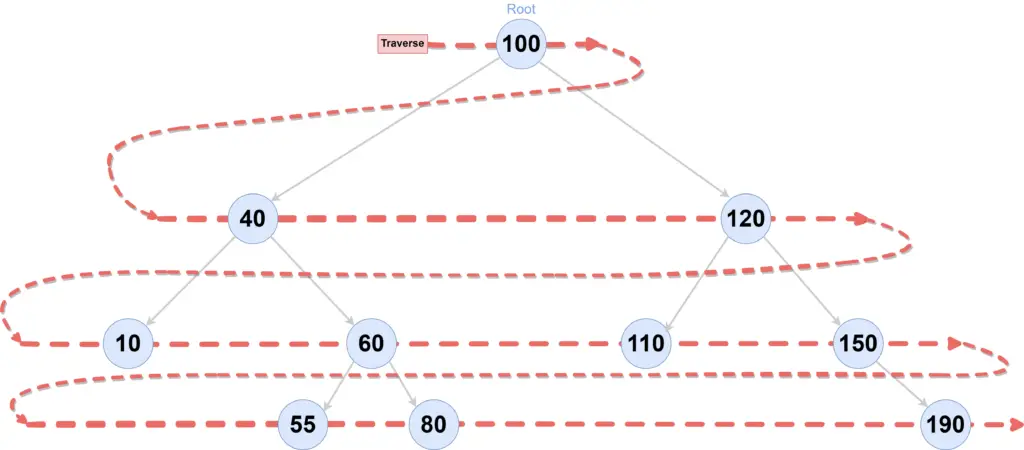
NOTE
In this article, we are discussing in detail, the implementation of Breadth First Search(BFS) JavaScript and TypeScript.
Step #1: Node Class
Step #2: BfsTree Class
Step #3: Insert Method
Step #2: bfs Method
Full Source Code
Demo
Output:
----------- BFS example -----------
[
100, 40, 120, 10,
60, 110, 150, 55,
80, 190
]
Testing
Time Complexity
Operation | Complexity |
---|---|
Insert | |
Search |
Source Code
Use the following links to get the source code used in this article-
Source Code of | Implementation Code | Demo Code | Test Code |
---|---|---|---|
JavaScript Implementation | ![]() | ![]() | ![]() |
TypeScript Implementation | ![]() | ![]() | ![]() |
Related Data Structures
Command | Details |
---|---|
Singly Linked List | ![]() |
Other Code Implementations
Use the following links to check the BFS implementation in other programming languages-