Summary
Pattern Name | Template Method Pattern |
Pattern Type | Behavioral Pattern |
Scope | Class |
Tagline | Let subclasses redefine certain steps of algorithm |
Use cases | When most of the steps will be the same for all subclasses and we want to rewrite a few steps in each subclass |
Related Patterns | Factory Method Strategy |
Difficulty Level | Easy |
Implementations | ![]() ![]() ![]() ![]() |
Definition
Template method pattern when we want the subclasses to define some steps of the processing steps (or algorithm). The parent class can define some steps and subclasses can define some steps(which are required).
So the parent(abstract) class works as an outline template for the algorithm or lifecycle of the process.
Functionalities/steps which are completely different for subclasses are left to the subclasses for definition. The parent (abstract) class works are a skeleton here.
Use Cases
Here are a few use cases of the Template Method design pattern-
- When we need to support algorithms that are similar but have different steps in the implementation.
- When we want to implement the variation of similar algorithms in separate subclasses.
- When we want to control at which step the implementation is changed for an algorithm.
- When we want subclasses to override some part of the implementation.
Implementation
Template Method pattern has 2 elements.
- Abstract Class: this defines some functions, which are common for all subclasses, and declares other methods which are different for subclasses. Then subclasses can define those methods for the functionality specific to that subclass.
- Concrete Classes: extends the abstract class. Defines the functions for the abstract functions.
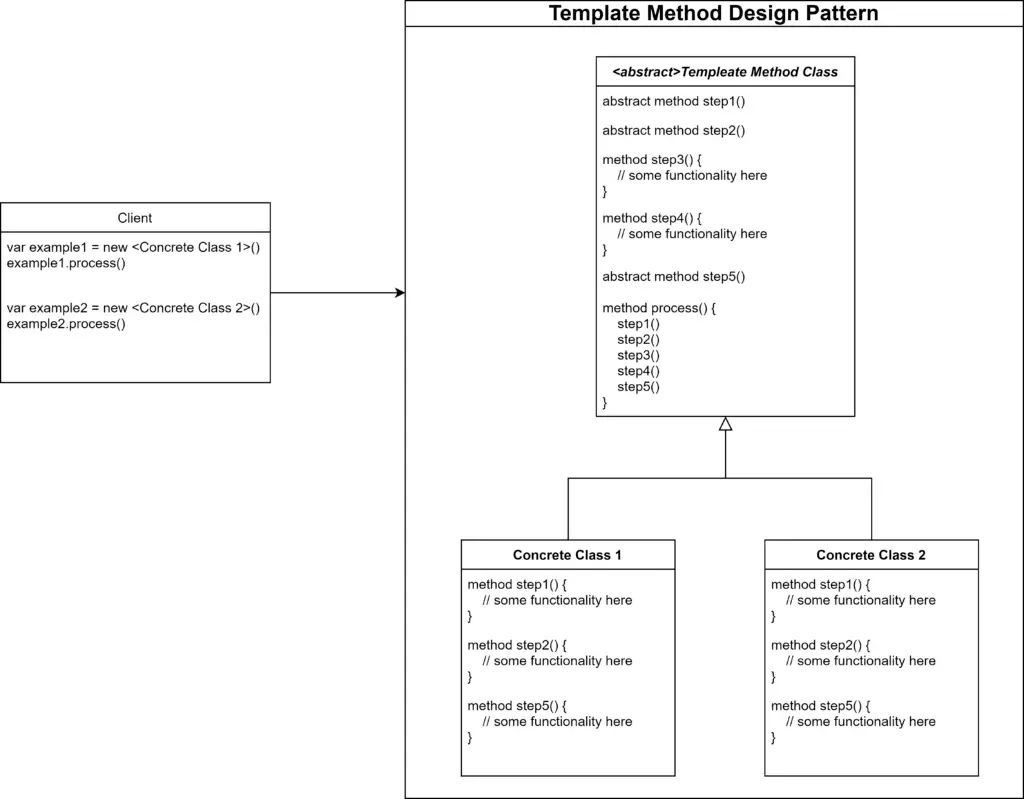
Follow the steps below to implement Template Method pattern:
- Create an abstract class.
- In the abstract class, define functions that will be common for all the sub-classes.
- In the abstract class, declare functions as abstract, for which functionalities are different for each subclass.
- In the abstract class, create a function that uses all the functions and performs the processing.
- Create concrete classes and extend the abstract class.
- Implement the required abstract methods in the concrete class.
Examples
Example #1: Transport Builder
Let’s implement Template Method for a transport builder.
Transport Template Method
abstract class Transport
abstract createBody()
abstract addEngine()
abstract addWheel()
// Required only for Plane
abstract addWing()
method addSeat()
// Add seats to the vehicle
// Adding seats are same for all transports so same functions for all
output "Adding seats"
end method
method build()
createBody()
addEngine()
addWheel()
addWing()
addSeat()
end method
end method
Transport Classes
// Car class
class Car extends Transport
method createBody()
output "Creating Car Body"
end method
method addEngine()
output "Adding Engine to Car"
end method
method addWheel()
output "Adding 4 Wheels to Car"
end method
method addWing()
// Not required for Car
end method
end class
// Bike class
class Bike extends Transport {
method createBody()
output "Creating Bike Body"
end method
method addEngine()
output "Adding Engine to Bike"
end method
method addWheel()
output "Adding 2 Wheels to Bike"
end method
method addWing()
// Not required for Bike
end method
end class
// Plane class
class Plane extends Transport
method createBody()
output "Creating Plane Body"
end method
method addEngine()
output "Adding Engine to Plane"
end method
method addWheel()
output "Adding 3 Wheels to Plane"
end method
method addWing()
output "Adding Wings Plane"
end method
end class
// Train class
class Train extends Transport
method createBody()
output "Creating Train Body"
end method
method addEngine()
output "Adding Engine to Train"
end method
method addWheel()
output "Adding Wheels to Train"
end method
method addWing()
// Not required for Train
end method
end class
Context
// Context
class Context
var state: State
method setState(stateParam: State)
state = stateParam
end method
method getState(): State
return state
end method
method performActionOne()
state.actionOne()
end method
method performActionTwo()
state.actionTwo()
end method
end class
Demo
var car: Transport = new Car()
car.build()
var plane: Transport = new Plane()
plane.build()
Output
Creating Car Body
Adding Engine to Car
Adding 4 Wheels to Car
Adding seats
Creating Plane Body
Adding Engine to Plane
Adding 3 Wheels to Plane
Adding Wings Plane
Adding seats
Code Implementations
Use the following links to check State pattern implementation in specific programming languages.