Summary
Pattern Name | Prototype Pattern |
Pattern Type | Creational Pattern |
Scope | ![]() |
Tagline | Create a new object by copying/cloning an existing one |
Use cases | When we want to optimize the object creation performance |
Related Patterns | ![]() ![]() ![]() ![]() |
Difficulty Level | Easy |
Implementations | ![]() ![]() ![]() ![]() ![]() |
Definition
Prototype pattern defines the process of cloning an existing object and creating a new object from that. Prototype is the pattern for creating new objects, keeping performance in mind.
When we want to simplify the creation process of an object and optimize the performance of object creation, we can clone an existing object and create a new one from that.
The main focus of Prototype pattern is to reduce the resource usage of the creational process of new object instances.
Use Cases
Implementation
In Prototype pattern, the object that we are copying should contain the copying feature(clone) itself. The copying is not done using other classes.
Prototype pattern implementation in different languages will differ, as the implementation depends on some language feature. Here we are discussing the common implementation of Prototype pattern.
Language-specific implementation of Prototype pattern is discussed in separate individual articles. Check the language-specific implementations here at: Prototype Pattern Code Implementations
Cloning objects can be difficult if there are circular references.
Basic Prototype pattern implementation has 2 elements.
- Prototype Interface: an interface to have a common interface for all objects that supports the prototyping process. This interface will declare a method for cloning objects.
- Prototype Concrete Classes: classes that implement the Prototype Interface, and for the implementation these classes define a cloning method. Usually, that method is named as “clone“.
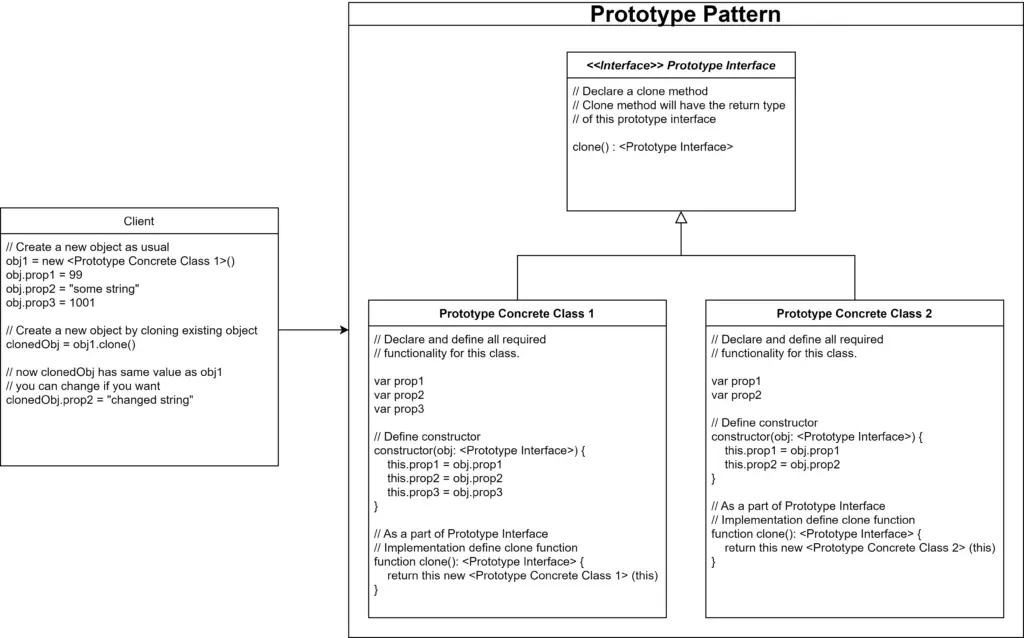
Here are the steps to follow for implementing Prototype pattern:
- Create an interface (or abstract class) and declare a function for cloning.
- Create concrete classes and implement the interface.
- Declare a constructor that accepts param.
- In the clone function implementation create a new object of the same class and return it.
Examples
Let’s take a look at some examples of Prototype pattern-
Example #1: Transport
Let’s take the example of a transport system. Take a look at the implementation.
Prototype Interface
interface Prototype
// Return type is of Prototype
clone(): Prototype
end interface
Prototype Implementations
class Car implements Prototype
var make: int
var model: String
var color: String
// Empty constuctor
constructor()
// Add any required operation
// or keep it empty
end constructor
// Constructor
constructor(carParam: Car)
this.make = carParam.make
this.model = carParam.model
this.color = carParam.color
end constructor
function clone(): Prototype
return new Car(this)
end function
function toString(): String
return "Make: " + make + " | Model: " + model + " | Color: " + color
end function
end class
class Plane implements Prototype
var model: String
var color: String
// Empty constructor
constructor()
// Add any required operation
// or keep it empty
end constructor
// Consctuctor
constructor(planeParam: Plane)
this.model = planeParam.model
this.color = planeParam.color
end constructor
function clone(): Prototype
return new Plane(this)
end function
function toString(): String
return "Model: " + model + " | Color: " + color
end function
end class
Demo
var car1: Car = new Car()
car1.make = 2014
car1.model = "ABCD"
car1.color = "Red"
print(car1.toString())
// Clone existing car object and create a new one
Car carClone = (Car) car1.clone() // Cast the returned object of type Prototype to Car to match behavior. This depends on the programming language.
carClone.model = "Some Different Model"
carClone.color = "White"
print(carClone.toString())
Output
Make: 2014 | Model: ABCD | Color: Red
---------------------------------------
Make: 2014 | Model: Some Different Model | Color: White
Code Implementations
Use the following links to check Prototype pattern implementation in specific programming languages.