Summary
Pattern Name | Mediator Pattern |
Pattern Type | Behavioral Pattern |
Scope | Object |
Tagline | Middleman between two objects |
Use cases | 1. When we want to stop objects from interacting directly with each other. |
Related Patterns | Facade Observer |
Difficulty Level | Medium |
Implementations | ![]() ![]() ![]() ![]() |
Definition
Mediator pattern prevents direct interaction between objects and works as a middleman. As objects can not refer to another object directly in this pattern, that’s why it ensures loose coupling.
In Mediator pattern, all communication between objects will be through the Mediator. Check the following diagram.
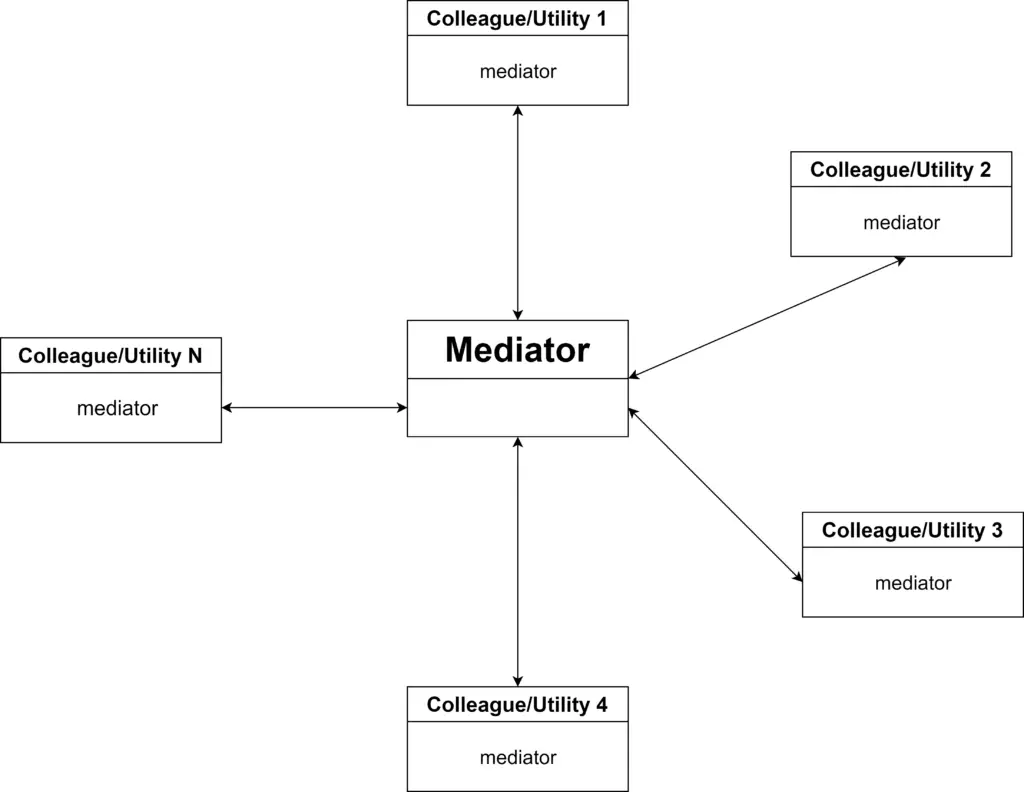
A mediator takes responsibility for communication between objects, and can keep track of the communication.
Other objects are aware of the mediator, then know that they need the help of the mediator for communication.
Use Cases
Here are the use cases of Mediator design pattern-
- When we want to centralize complex communication between objects.
- When we want to control the communication between objects.
- When we want the objects of a system to be free of communication responsibility among them.
Implementation
Mediator pattern has 4 elements.
- Mediator Interface: interface to ensure the type of mediator class.
- Concrete Mediator Class: implements the interface, and works as the medium of communication between the colleague/utility classes.
- Utility(Colleague) Interface [optional]: interface to ensure the type of colleague classes. This is optional though, you can implement the pattern without this interface.
- Concrete Utility Classes (colleagues): classes that have some use-case, and these classes need to communicate with each other. These classes use the mediator class to communicate.
Take a look at the implementation diagram.
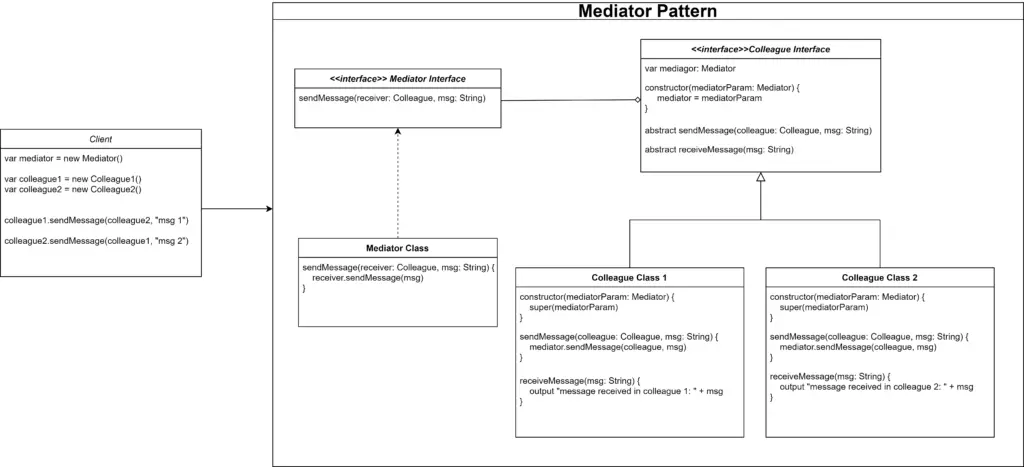
Follow the steps below to implement Mediator pattern:
- Create an interface for mediator class. Declare a function (sendMessage) that accepts messages.
- Create mediator class, and implement the interface. in the message sending function call message sending function of the colleague.
- Create an interface or abstract class for colleague/utility classes. Implement a way to receive mediator object and methods for sending and receiving messages.
- Create colleague/utility classes and implement the colleague interface.
- In the client create a mediator object. Create utility/colleague objects and pass mediator object.
- Then use the colleague objects’ message-sending function to send messages to the colleague object, which in turn uses the mediator object to send the message.
Examples
Example #1: General Mediator
Let’s take the example of a general mediator.
Mediator Interface and Class
// Mediator Interface
interface IMediator
method sendMessage(colleague: Colleague, msg: string)
end interface
// Mediator Class
class Mediator implements IMediator
method sendMessage(receiver: Colleague, msg: string)
receiver.receiveMessage(msg)
end method
end class
Colleague/Utility Interface and Class
// Interface
class Colleague
var mediator: Mediator
constructor (mediator: MediatorParam)
mediator = mediatorParam
end constructor
abstract method sendMessage(colleague: Colleague, message: string)
abstract method receiveMessage(message: string)
end class
// Colleague Classes
class Colleague1 extends Colleague
constructor(mediator: Mediator)
super(mediator)
end constructor
method sendMessage(colleague: Colleague, msg: string)
mediator.sendMessage(colleague, msg)
end method
method receiveMessage(msg: string)
output "Message received in Colleague1: " + msg
end method
end class
class Colleague2 extends Colleague
constructor(mediator: Mediator)
super(mediator)
end constructor
method sendMessage(colleague: Colleague, msg: string)
mediator.sendMessage(colleague, msg)
end method
method receiveMessage(msg: string)
output "Message received in Colleague2: " + msg
end method
end class
class Colleague3 extends Colleague
constructor(mediator: Mediator)
super(mediator)
end constructor
method sendMessage(colleague: Colleague, msg: string)
mediator.sendMessage(colleague, msg)
end method
method receiveMessage(msg: string)
output "Message received in Colleague3: " + msg
end method
end class
Demo
mediator = new Mediator()
colleague1 = new Colleague1(mediator)
colleague2 = new Colleague2(mediator)
colleague3 = new Colleague3(mediator)
colleague1.sendMessage(colleague2, "message from colleague1")
colleague1.sendMessage(colleague3, "message from colleague1")
colleague2.sendMessage(colleague3, "message from colleague2")
colleague3.sendMessage(colleague1, "message from colleague3")
Output
Page Number: 0
Page Path: /page/0
----------------------------------------
Page Number: 1
Page Path: /page/1
----------------------------------------
Page Number: 2
Page Path: /page/2
----------------------------------------
Page Number: 3
Page Path: /page/3
----------------------------------------
Page Number: 4
Page Path: /page/4
----------------------------------------
Page Number: 5
Page Path: /page/5
----------------------------------------
Page Number: 6
Page Path: /page/6
----------------------------------------
Page Number: 7
Page Path: /page/7
----------------------------------------
Page Number: 8
Page Path: /page/8
----------------------------------------
Page Number: 9
Page Path: /page/9
----------------------------------------
Code Implementations
Use the following links to check Mediator pattern implementation in specific programming languages.