Summary
Pattern Name | Iterator Pattern |
Pattern Type | Behavioral Pattern |
Scope | Object |
Other Names | Cursor |
Tagline | Traverse through a list of items |
Use cases | 1. When we need to iterate through a list 2. When we need to hide the complex iteration process of a list |
Related Patterns | Factory Composite Memento |
Difficulty Level | Easy |
Implementations | ![]() ![]() ![]() ![]() |
Definition
Iterator pattern is used when we need to traverse through the items of an aggregate object (list or collection of items). Iterator is a very commonly used pattern.
Aggregate objects are lists of items, like collections, linked lists, hash tables, etc.
Iterator pattern hides the complexity of the iteration process and makes it easier to go through a list of items. Client doesn’t know about the underlying complexity of the pattern.
Use Cases
Here are a few use cases of the Iterator pattern-
- When we want to provide a standard way of iteration for a collection.
- When we want to hide the complexity of the iteration of an aggregate object, from a client.
Implementation
Iterator pattern has 4 elements involved in the implementation.
- Abstract List Interface: interface (or abstract class) to ensure the type of list class.
- Concrete List Class: class for the functionality of the list – add, remove, and modify items to the list. This class has a function (generally named ‘iterator’) to generate an iterator object and return that. This class implements the Abstract list Interface.
- Abstract Iterator Interface: interface to ensure the type of iterator.
- Concrete Iterator Class: class to implement all the required functionality for an iterator. Functions like hasNext(), getNextItem() are implemented in this class. This class implements the Abstract Iterator Interface.
Take a look at the implementation diagram.
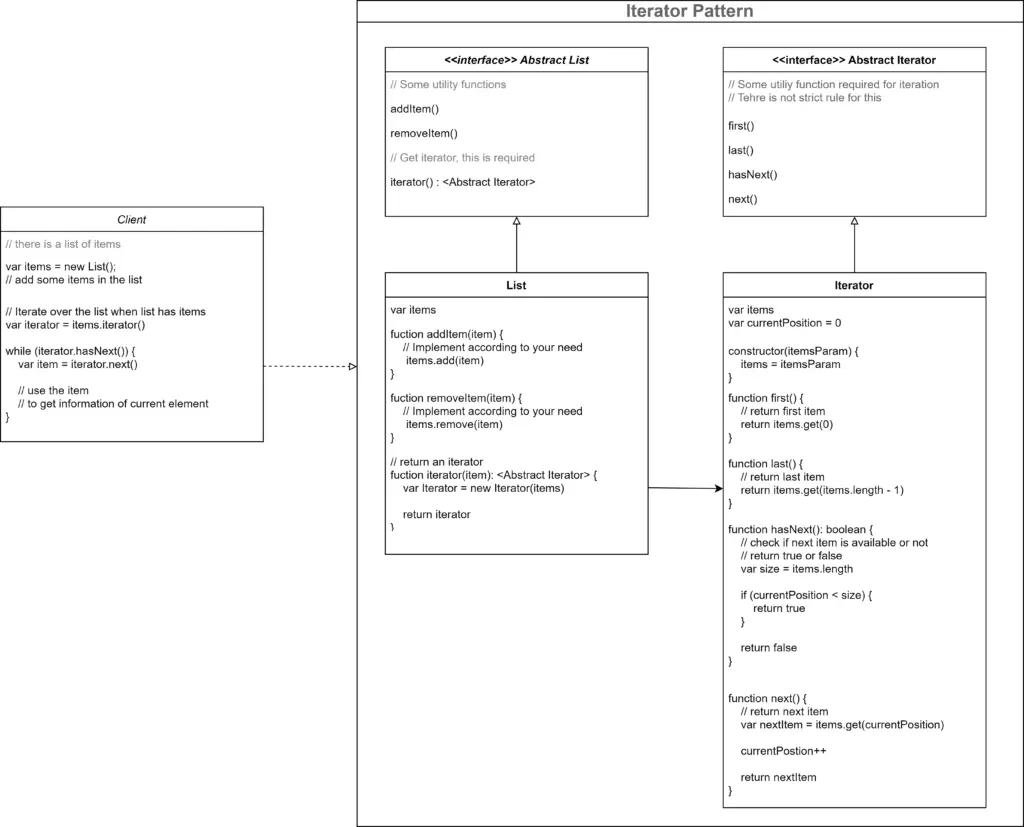
Follow the steps below to implement Iterator pattern:
- Create the interface for the list. Declare functions for adding, editing, delete items from the list. Also, add a method to generate an iterator and return that.
- Create a list class, and implement the list interface methods.
- Create an interface for the iterator. Declare functions for checking and getting the next items.
- Create the iterator class, and implement methods declared in the iterator interface.
- In the client create an instance of the list. Then get the iterator from the list.
- Then, in the client iterate over the items, using the methods for checking and getting the next items.
Examples
Example #1: Pagination Iterator
Let’s take the example of pagination.
List Interface and Class
// Abstract List Iterface
interface AbstractList
add(Page page)
remove(Page page)
iterator(): AbstractIterator
end interface
// List Class
class PageList implements AbstractList
var pages
// Utility function, implement according to your need
method add(page: Page)
pages.add(page)
end method
// Utility function, implement according to your need
method remove(page: Page)
pages.remove(page)
end method
// Generate and get iterator
method iterator(): AbstractIterator
return new Iterator(pages)
end method
end class
Iterator Interface and Class
// Abstract Iterator Interface
interface AbstractIterator
hasNext(): boolean
next()
end interface
// Iterator class
class Iterator implements AbstractIterator
var currentPosition = 0
var items
constructor(itemsParam)
items = itemsParam
end constructor
method hasNext(): boolean
// Check if there is any item available next
return currentPosition < items.length
end method
method next()
var item = items.get(currentPosition)
// Increate the current position for next time use
currentPosition++
return item
end method
end class
Demo
var pageList: PageList = new PageList()
// Add dummy data for testing
for (int i = 0; i < 10; i++) {
var page: Page = new Page()
page.setNumber(i)
pageList.add(page)
}
// Get iterator
AbstractIterator paginator = pageList.iterator();
// Iterate over the elements
while(paginator.hasNext()) {
var page: Page = paginator.next();
output "Page Number: " + page.getNumber()
output "Page Path: " + page.getPath()
}
Output
Page Number: 0
Page Path: /page/0
----------------------------------------
Page Number: 1
Page Path: /page/1
----------------------------------------
Page Number: 2
Page Path: /page/2
----------------------------------------
Page Number: 3
Page Path: /page/3
----------------------------------------
Page Number: 4
Page Path: /page/4
----------------------------------------
Page Number: 5
Page Path: /page/5
----------------------------------------
Page Number: 6
Page Path: /page/6
----------------------------------------
Page Number: 7
Page Path: /page/7
----------------------------------------
Page Number: 8
Page Path: /page/8
----------------------------------------
Page Number: 9
Page Path: /page/9
----------------------------------------
Code Implementations
Use the following links to check Iterator pattern implementation in specific programming languages.