Boolean represents a value that can be either true or false. Everything that is evaluated to a boolean can be one of 2 values-
- True
- False
If we convert any other data type to Boolean, it will be either True or False.
Create Boolean
To create a boolean value, assign True or False to create a boolean value-
big_box_bool = True
print(big_box_bool)
some_false_val = False
print(some_false_val)
print(type(big_box_bool))
PythonOutput:
True
False
<class 'bool'>
PlaintextOr, we can use the “bool()” function to create a boolean value-
some_bool_val = bool()
print(f"bool() with blank is: {some_bool_val}")
some_bool_val = bool("")
print(f"Bool of empty string is: {some_bool_val}")
some_bool_val = bool("BigBoxCode")
print(f"Bool of BigBoxCode is: {some_bool_val}")
some_bool_val = bool(0)
print(f"Bool of 0 is: {some_bool_val}")
some_bool_val = bool(1)
print(f"Bool of 1 is: {some_bool_val}")
some_bool_val = bool(True)
print(f"Bool of True is: {some_bool_val}")
some_bool_val = bool(False)
print(f"Bool of False is: {some_bool_val}")
PythonOutput:
bool() with blank is: False
Bool of empty string is: False
Bool of BigBoxCode is: True
Bool of 0 is: False
Bool of 1 is: True
Bool of True is: True
Bool of False is: False
PlaintextSize of Boolean
Let’s check the size of a boolean value in memory.
To get the size we have to use “getsizeof()” function from “sys” package. This function returns the size in bytes.
We can see the size of a boolean in memory is 28 bytes, in a 64-bit system.
NOTES
We are using a 64-bit system. So the result is for a 64-bit system.
import sys
print(sys.getsizeof(True))
print(sys.getsizeof(False))
PythonOutput:
28
28
PlaintextBoolean Reassignment Internals
Let’s create some boolean variables and check their internal ID.
first_bool = True
print("first_bool: ", id(first_bool))
second_bool = False
print("second_bool: ", id(second_bool))
third_bool = True
print("third_bool: ", id(third_bool))
fourth_bool = False
print("fourth_bool: ", id(fourth_bool))
fifth_bool = True
print("fifth_bool: ", id(fifth_bool))
PythonOutput:
All the True values have the same ID and all the false values have the same ID. Because all True variables refer to the same object in memory and the same for the False variables.
first_bool: 140727304839568
second_bool: 140727304839600
third_bool: 140727304839568
fourth_bool: 140727304839600
fifth_bool: 140727304839568
PlaintextHere is how it looks in memory-
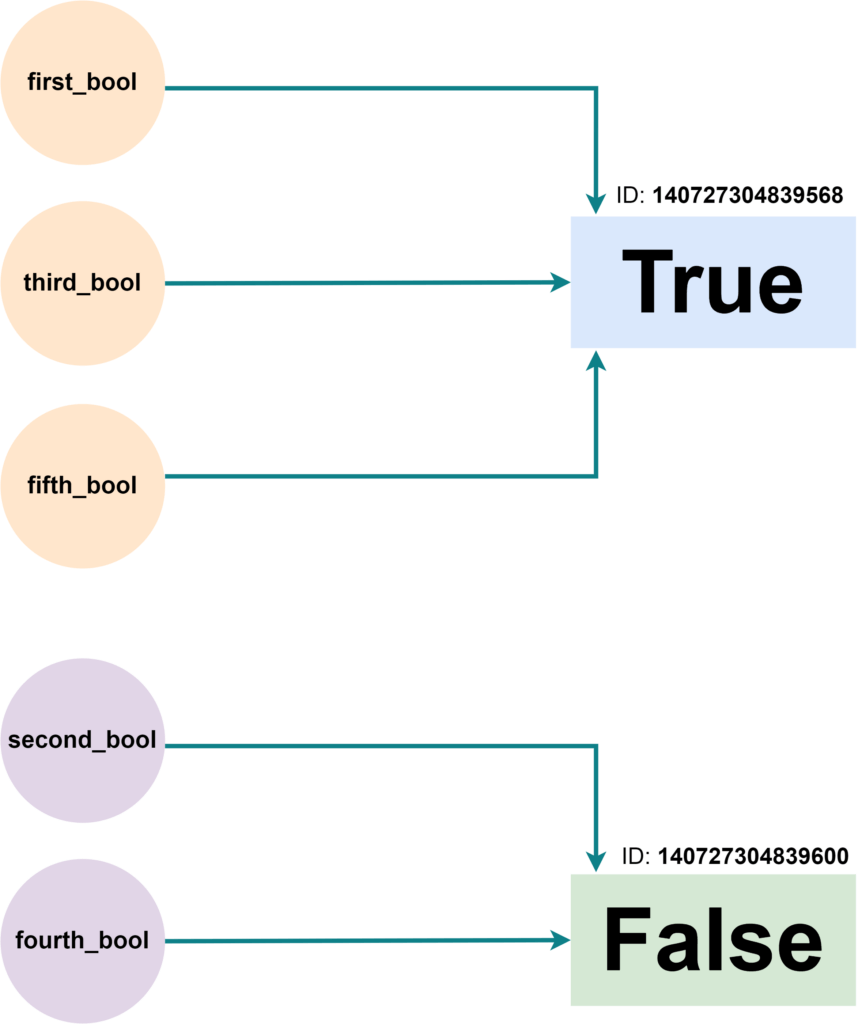
Now let’s see what happens when we change the value of a boolean variable-
import ctypes
big_box_bool = True
first_id = id(big_box_bool)
print("Initial id: ", first_id)
big_box_bool = False
print("ID after change: ", id(big_box_bool))
obj = ctypes.cast(first_id, ctypes.py_object)
print("Old boolean object: ", obj)
PythonOutput:
When reassigned, the variable will point to a new object in memory. And the previous boolean object remains the same in memory.
Initial id: 140727304839568
ID after change: 140727304839600
Old boolean object: py_object(True)
PlaintextHere is how the reassignment operation looks in memory-
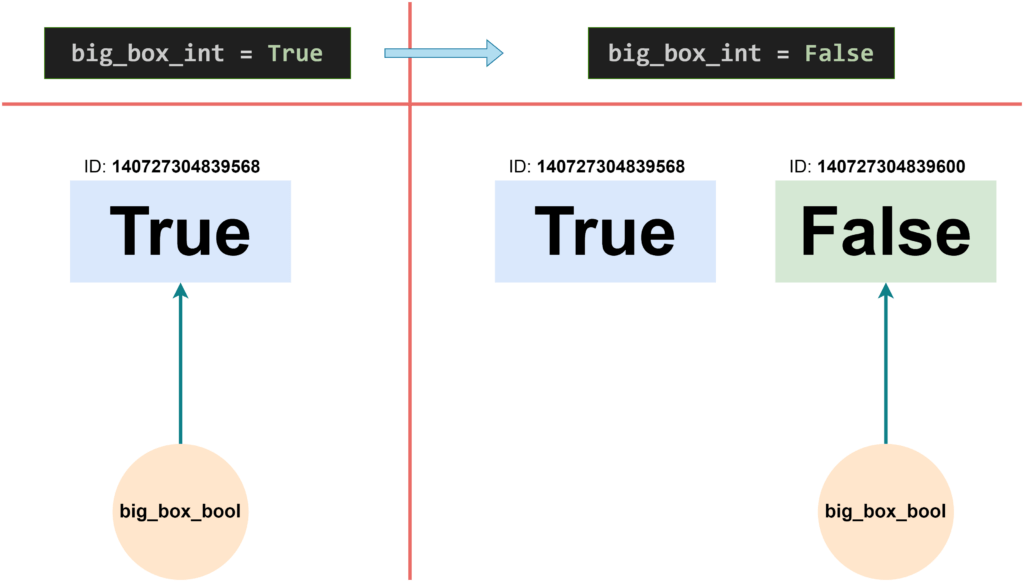
Value of Boolean
When a boolean is evaluated for its value, we get-
- True: is equivalent to integer value 1.
- False: is equivalent to integer value 0.
sum_one = True + 1
print("Value of True + 1 is: ", sum_one)
sum_two = False + 1
print("Value of False + 1 is: ", sum_two)
PythonOutput:
Value of True + 1 is: 2
Value of False + 1 is: 1
PlaintextLogical Operation
big_box_bool = True and False
print("Value of (True and False) is: ", big_box_bool)
big_box_bool = True or False
print("Value of (True or False) is: ", big_box_bool)
big_box_bool = not True
print("Value of (not True) is: ", big_box_bool)
big_box_bool = not False
print("Value of (not False) is: ", big_box_bool)
PythonOutput:
Value of (True and False) is: False
Value of (True or False) is: True
Value of (not True) is: False
Value of (not False) is: True
PlaintextComparison Operator
print("Value of 10 == 10 : ", 10 == 10)
print("Value of 10 == 3 : ", 10 == 3)
print("Value of 10 != 3 : ", 10 != 3)
print("Value of 10 != 10 : ", 10 != 10)
print("Value of 10 > 3 : ", 10 > 3)
print("Value of 10 < 3 : ", 10 < 3)
print("Value of 10 >= 3 : ", 10 >= 3)
print("Value of 10 >= 10 : ", 10 >= 10)
print("Value of 10 >= 100 : ", 10 >= 100)
print("Value of 10 <= 3 : ", 10 <= 3)
print("Value of 10 <= 10 : ", 10 <= 10)
print("Value of 10 <= 100 : ", 10 <= 100)
PythonOutput:
Value of 10 == 10 : True
Value of 10 == 3 : False
Value of 10 != 3 : True
Value of 10 != 10 : False
Value of 10 > 3 : True
Value of 10 < 3 : False
Value of 10 >= 3 : True
Value of 10 >= 10 : True
Value of 10 >= 100 : False
Value of 10 <= 3 : False
Value of 10 <= 10 : True
Value of 10 <= 100 : True
PlaintextConverting to Boolean
Here is what happens when we convert other data types to boolean-
Integer to Boolean
NOTES
Here is a summary of what happens when integers are converted to boolean-
Integer value(s) to convert | Boolean Value |
---|---|
0 | False |
All positive values | True |
All negative values | True |
print("Value of bool(1) is: ", bool(1))
print("Value of bool(-1) is: ", bool(-1))
print("Value of bool(100) is: ", bool(100))
print("Value of bool(-100) is: ", bool(-100))
print("Value of bool(0) is: ", bool(0))
print("Value of bool(-0) is: ", bool(-0))
PythonOutput:
Value of bool(1) is: True
Value of bool(-1) is: True
Value of bool(100) is: True
Value of bool(-100) is: True
Value of bool(0) is: False
Value of bool(-0) is: False
PlaintextFloat to Boolean
NOTES
Here is a summary of what happens when float are converted to boolean-
Float value(s) to convert | Boolean Value |
---|---|
0.0 | False |
All positive values | True |
All negative values | True |
print("Value of bool(1.0) is: ", bool(1.0))
print("Value of bool(-1.0) is: ", bool(-1.0))
print("Value of bool(0.1) is: ", bool(0.1))
print("Value of bool(-0.1) is: ", bool(-0.1))
print("Value of bool(0.0) is: ", bool(0.0))
print("Value of bool(-0.0) is: ", bool(-0.0))
print("Value of bool(1e100) is: ", bool(1e100))
print("Value of bool(-1e100) is: ", bool(-1e100))
print("Value of bool(float('inf')) is: ", bool(float('inf')))
print("Value of bool(float('-inf')) is: ", bool(float('-inf')))
print("Value of bool(float('nan')) is: ", bool(float('nan')))
PythonOutput:
Value of bool(1.0) is: True
Value of bool(-1.0) is: True
Value of bool(0.1) is: True
Value of bool(-0.1) is: True
Value of bool(0.0) is: False
Value of bool(-0.0) is: False
Value of bool(1e100) is: True
Value of bool(-1e100) is: True
Value of bool(float('inf')) is: True
Value of bool(float('-inf')) is: True
Value of bool(float('nan')) is: True
PlaintextString to Boolean
NOTES
Here is a summary of what happens when strings are converted to boolean-
String value(s) to convert | Boolean Value |
---|---|
Empty String | False |
Non-Empty String | True |
print("Value of bool('') is: ", bool(''))
print("Value of bool(' ') is: ", bool(' '))
print("Value of bool('\\n') is: ", bool('\n'))
print("Value of bool('true') is: ", bool('true'))
print("Value of bool('TRUE') is: ", bool('TRUE'))
print("Value of bool('1') is: ", bool("1"))
print("Value of bool('yes) is: ", bool("yes"))
print("Value of bool('no') is: ", bool("no"))
print("Value of bool('false') is: ", bool("false"))
print("Value of bool('FALSE') is: ", bool("FALSE"))
print("Value of bool('0') is: ", bool("0"))
print("Value of bool('on') is: ", bool("on"))
print("Value of bool('off') is: ", bool("off"))
print("Value of bool('maybe') is: ", bool("maybe"))
print("Value of bool('123') is: ", bool("123"))
print("Value of bool('null') is: ", bool("null"))
PythonOutput:
Value of bool('') is: False
Value of bool(' ') is: True
Value of bool('\n') is: True
Value of bool('true') is: True
Value of bool('TRUE') is: True
Value of bool('1') is: True
Value of bool('yes) is: True
Value of bool('no') is: True
Value of bool('false') is: True
Value of bool('FALSE') is: True
Value of bool('0') is: True
Value of bool('on') is: True
Value of bool('off') is: True
Value of bool('maybe') is: True
Value of bool('123') is: True
Value of bool('null') is: True
PlaintextTuple to Boolean
NOTES
Here is a summary of what happens when tuples are converted to boolean-
Tuple value(s) to convert | Boolean Value |
---|---|
Empty Tuple | False |
Non-Empty Tuple | True |
print("Value of bool(()) is: ", bool(()))
print("Value of bool((1, 2, 3)) is: ", bool((1, 2, 3)))
print("Value of bool((None,)) is: ", bool((None,)))
print("Value of bool((False,)) is: ", bool((False,)))
PythonOutput:
Value of bool(()) is: False
Value of bool((1, 2, 3)) is: True
Value of bool((None,)) is: True
Value of bool((False,)) is: True
PlaintextList to Boolean
NOTES
Here is a summary of what happens when lists are converted to boolean-
List value(s) to convert | Boolean Value |
---|---|
Empty List | False |
Non-Empty List | True |
print("Value of bool([]) is: ", bool([]))
print("Value of bool([1, 2, 3]) is: ", bool([1, 2, 3]))
print("Value of bool([0]) is: ", bool([0]))
print("Value of bool([""]) is: ", bool([""]))
print("Value of bool([None]) is: ", bool([None]))
print("Value of bool([0, None, "", [], False]) is: ", bool([0, None, "", [], False]))
print("Value of bool([1, "", None, 2]) is: ", bool([1, "", None, 2]))
print("Value of bool([[], [], []]) is: ", bool([[], [], []]))
print("Value of bool([1, 'Hello'', [1, 2]]) is: ", bool([1, "Hello", [1, 2]]))
PythonOutput:
Value of bool([]) is: False
Value of bool([1, 2, 3]) is: True
Value of bool([0]) is: True
Value of bool([]) is: True
Value of bool([None]) is: True
Value of bool([0, None, , [], False]) is: True
Value of bool([1, , None, 2]) is: True
Value of bool([[], [], []]) is: True
Value of bool([1, 'Hello'', [1, 2]]) is: True
PlaintextDictionary to Boolean
NOTES
Here is a summary of what happens when dictionaries are converted to boolean-
Dictionary value(s) to convert | Boolean Value |
---|---|
Empty Dictionary | False |
Non-Empty Dictionary | True |
print("Value of bool({}) is: ", bool({}))
print("Value of bool({\"key\": \"value\"}) is: ", bool({"key": "value"}))
print("Value of bool({\"key\": 0}) is: ", bool({"key": 0}))
print("Value of bool({\"key\": None}) is: ", bool({"key": None}))
print("Value of bool({\"key\": ""}) is: ", bool({"key": ""}))
print("Value of bool({\"key1\": 0, \"key2\": None}) is: ", bool({"key1": 0, "key2": None}))
print("Value of bool({\"key1\": [], \"key2\": {}}) is: ", bool({"key1": [], "key2": {}}))
print("Value of bool({\"key1\": True, \"key2\": \"value\"}) is: ", bool({"key1": True, "key2": "value"}))
PythonOutput:
Value of bool({}) is: False
Value of bool({"key": "value"}) is: True
Value of bool({"key": 0}) is: True
Value of bool({"key": None}) is: True
Value of bool({"key": }) is: True
Value of bool({"key1": 0, "key2": None}) is: True
Value of bool({"key1": [], "key2": {}}) is: True
Value of bool({"key1": True, "key2": "value"}) is: True
PlaintextSet to Boolean
NOTES
Here is a summary of what happens when sets are converted to boolean-
Set value(s) to convert | Boolean Value |
---|---|
Empty Set | False |
Non-Empty Set | True |
print("Value of bool(set()) is: ", bool(set()))
print("Value of bool({1, 2, 3}) is: ", bool({1, 2, 3}))
print("Value of bool({0}) is: ", bool({0}))
print("Value of bool({None}) is: ", bool({None}))
print("Value of bool({\"\", 0}) is: ", bool({"", 0}))
print("Value of bool({1, \"hello\", True}) is: ", bool({1, "hello", True}))
print("Value of bool({0, None, False}) is: ", bool({0, None, False}))
PythonOutput:
Value of bool(set()) is: False
Value of bool({1, 2, 3}) is: True
Value of bool({0}) is: True
Value of bool({None}) is: True
Value of bool({"", 0}) is: True
Value of bool({1, "hello", True}) is: True
Value of bool({0, None, False}) is: True
Plaintext