Tuple is an immutable and ordered collection of items. A tuple is similar to a list, but the items can not be changed, once the tuple is created.
Tuple Capabilities
Here is what a Tuple in Python can and can’t do-
Tuple can do
Tuple can not do
Define a Tuple
Let’s see how we can define a tuple-
Method #1: Standard Tuple Definition
Separate the items with a comma(,) and enclose the whole thing with parentheses, to define a Tuple. Check the example below-
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
print(big_box_tpl)
print(type(big_box_tpl))
print(len(big_box_tpl))
print(dir(big_box_tpl))
PythonOutput:
('First', 'Second', 'test', 123, 456, 789, 'last')
<class 'tuple'>
7
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__getstate__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'count', 'index']
Plaintext NOTE
We can define an empty tuple by using just empty parentheses-
big_box_tpl = ()
print(big_box_tpl)
print(type(big_box_tpl))
print(len(big_box_tpl))
PythonOutput:
()
<class 'tuple'>
0
Plaintext WARNING
If we try to change any item of a tuple, it will throw an error-
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
big_box_tpl[1] = "changed"
PythonOutput:
Traceback (most recent call last):
File "tuple.py", line 3, in <module>
big_box_tpl[1] = "changed"
~~~~~~~~~~~^^^
TypeError: 'tuple' object does not support item assignment
PlaintextMethod #2: Just Comma Separating Items
We can define a tuple by just separating items with commas.
big_box_tpl = "Big", "Box", "Code"
print(big_box_tpl)
print(type(big_box_tpl))
print(len(big_box_tpl))
PythonOutput:
Note that, the tuple is printed as enclosed in parentheses. Though we defined it without parentheses, but it is internally represented with parentheses.
('Big', 'Box', 'Code')
<class 'tuple'>
3
Plaintext NOTE
If we want to define a tuple with only one item, without using parenthesis- then we have to add a comma at the end.
big_box_tpl = "Big",
print(big_box_tpl)
print(type(big_box_tpl))
print(len(big_box_tpl))
PythonOutput:
('Big',)
<class 'tuple'>
1
PlaintextObject Referencing
Tuples do not store the items, but store the reference object. When a tuple is created, it stores the references of the items, not the value of the items.
Case #1
The items in tuple, store the reference of individual item objects. The same item in multiple tuples has the same object reference, as those refer to the same object in memory.
i1 = 100
s1 = 'bigboxcode'
f1 = 99.99
print(f"i1 => value: {i1} || type: {type(i1)} || id: {id(i1)}")
print(f"s1 => value: {s1} || type: {type(s1)} || id: {id(s1)}")
print(f"f1 => value: {f1} || type: {type(f1)} || id: {id(f1)}")
big_box_tpl = ("abc", 100, "bigboxcode", 99.99)
print(f"big_box_tpl[0] => value: {big_box_tpl[0]} || type: {type(big_box_tpl[0])} || id: {id(big_box_tpl[0])}")
print(f"big_box_tpl[1] => value: {big_box_tpl[1]} || type: {type(big_box_tpl[1])} || id: {id(big_box_tpl[1])}")
print(f"big_box_tpl[2] => value: {big_box_tpl[2]} || type: {type(big_box_tpl[2])} || id: {id(big_box_tpl[2])}")
print(f"big_box_tpl[3] => value: {big_box_tpl[3]} || type: {type(big_box_tpl[3])} || id: {id(big_box_tpl[3])}")
PythonOutput:
Value from tuple has the same object reference as the previously defined individual values.
i1 => value: 100 || type: <class 'int'> || id: 140479784373584
s1 => value: bigboxcode || type: <class 'str'> || id: 140479783246128
f1 => value: 99.99|| type: <class 'float'> || id: 140479782931728
big_box_tpl[0] => value: abc || type: <class 'str'> || id: 140479783191088
big_box_tpl[1] => value: 100 || type: <class 'int'> || id: 140479784373584
big_box_tpl[2] => value: bigboxcode || type: <class 'str'> || id: 140479783246128
big_box_tpl[3] => value: 99.99 || type: <class 'float'> || id: 140479782931728
PlaintextHere is how the referencing looks under the hood-
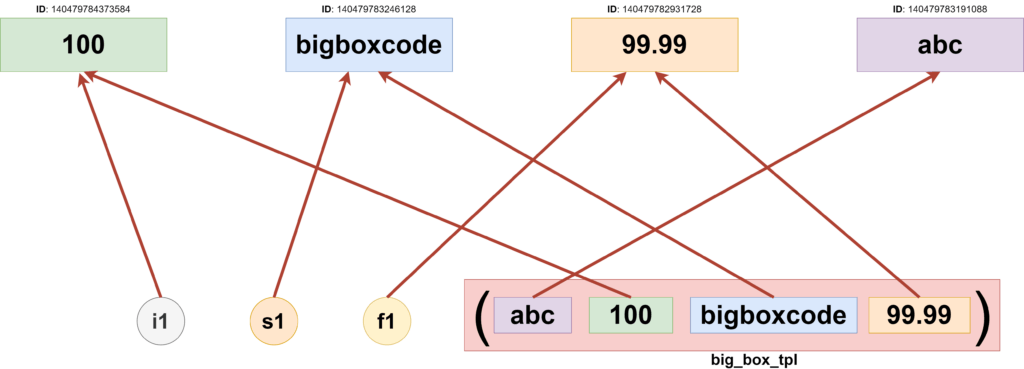
Case #2
Multiple Tuples defined with the same values, in the same sequence refer to the same object.
big_box_tpl = ("abc", 100, "bigboxcode", 99.99)
print(f"big_box_tpl => value: {big_box_tpl} || type: {type(big_box_tpl)} || id: {id(big_box_tpl)}")
# Define another tuple with the same values
big_box_tpl1 = ("abc", 100, "bigboxcode", 99.99)
print(f"big_box_tpl1 => value: {big_box_tpl1} || type: {type(big_box_tpl1)} || id: {id(big_box_tpl1)}")
# Assign big_box_tpl1 to big_box_tpl2
big_box_tpl2 = big_box_tpl1
print(f"big_box_tpl2 => value: {big_box_tpl2} || type: {type(big_box_tpl2)} || id: {id(big_box_tpl2)}")
# Create tuple with same values, but in different sequence
big_box_tpl3 = (100, "abc", 99.99, "bigboxcode")
print(f"big_box_tpl3 => value: {big_box_tpl3} || type: {type(big_box_tpl3)} || id: {id(big_box_tpl3)}")
PythonOutput:
big_box_tpl => value: ('abc', 100, 'bigboxcode', 99.99) || type: <class 'tuple'> || id: 139660434219280
big_box_tpl1 => value: ('abc', 100, 'bigboxcode', 99.99) || type: <class 'tuple'> || id: 139660434219280
big_box_tpl2 => value: ('abc', 100, 'bigboxcode', 99.99) || type: <class 'tuple'> || id: 139660434219280
big_box_tpl3 => value: (100, 'abc', 99.99, 'bigboxcode') || type: <class 'tuple'> || id: 139660434224560
PlaintextItem Indexing in Tuple
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
print(big_box_tpl[0])
print(big_box_tpl[2])
print(big_box_tpl[6])
print(big_box_tpl[-1])
PythonOutput:
First
test
last
last
PlaintextNesting in Tuple
We can add other tuples, lists, dictionaries, etc. to a Tuple. The behavior of those lists, dictionaries and other data types will remain the same.
Define Nested Items
Add other data types directly into the Tuple.
big_box_tpl = (
1,
2,
3,
("abc", "def"),
["big", "box", "code"],
{
"site": "BigBoxCode",
},
{
"first item",
"second ite",
},
)
print(big_box_tpl)
PythonOutput:
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code'], {'site': 'BigBoxCode'}, {'first item', 'second ite'})
PlaintextWe can also separately define the lists, dictionaries, sets and then add it to the tuple-
tpl1 = ("abc", "def")
list1 = ["big", "box", "code"]
dict1 = {
"site": "BigBoxCode",
}
set1 = {
"first item",
"second ite",
}
big_box_tpl = (1, 2, 3, tpl1, list1, dict1, set1)
print(big_box_tpl)
PythonOutput:
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code'], {'site': 'BigBoxCode'}, {'first item', 'second ite'})
PlaintextChange Items in Nested Tuple
The behavior of a list, or dictionary, or other data type remains the same even when it is nested inside a Tuple. We can still change those items.
tpl1 = ("abc", "def")
list1 = ["big", "box", "code"]
dict1 = {
"site": "BigBoxCode",
}
set1 = {
"first item",
"second item",
}
big_box_tpl = (1, 2, 3, tpl1, list1, dict1, set1)
print(big_box_tpl)
# Change the list
list1.append("New item 1")
# Change dictioary
dict1["url"] = "https://bigboxcode.com"
dict1["github"] = "github.com/webhkp/bigboxcode"
# Change the set
set1.add("Another item")
set1.add(999.99)
print(big_box_tpl)
PythonOutput:
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code'], {'site': 'BigBoxCode'}, {'first item', 'second item'})
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code', 100], {'site': 'BigBoxCode', 'github': 'github.com/webhkp/bigboxcode'}, {'first item', 'second item', 999.99})
PlaintextThe same thing happens when we define those lists, dictionaries and sets separately-
tpl1 = ("abc", "def")
list1 = ["big", "box", "code"]
dict1 = {
"site": "BigBoxCode",
}
set1 = {
"first item",
"second item",
}
big_box_tpl = (1, 2, 3, tpl1, list1, dict1, set1)
print(big_box_tpl)
# Change the list
list1.append("New item 1")
# Change dictioary
dict1["url"] = "https://bigboxcode.com"
dict1["github"] = "github.com/webhkp/bigboxcode"
# Change the set
set1.add("Another item")
set1.add(999.99)
print(big_box_tpl)
PythonOutput:
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code'], {'site': 'BigBoxCode'}, {'first item', 'second item'})
(1, 2, 3, ('abc', 'def'), ['big', 'box', 'code', 'New item 1'], {'site': 'BigBoxCode', 'url': 'https://bigboxcode.com', 'github': 'github.com/webhkp/bigboxcode'}, {'Another item', 'first item', 999.99, 'second item'})
PlaintextLooping through Tuple
Case #1
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
for item in big_box_tpl:
print(item)
PythonOutput:
First
Second
test
123
456
789
last
PlaintextCase #2
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
# Start from index 0
item_index = 0
while item_index < len(big_box_tpl):
print(f"Index: {item_index} || Item value: {big_box_list[item_index]}")
# Increase the index by 1
item_index += 1
PythonOutput:
First
Second
test
123
456
789
last
PlaintextTuple Concatenation
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
tuple2 = ("new 1", "new 2", "new 3")
print(big_box_tpl + tuple2)
PythonOutput:
('First', 'Second', 'test', 123, 456, 789, 'last', 'new 1', 'new 2', 'new 3')
PlaintextCheck Item Existence
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
print("First" in big_box_tpl)
print(123 in big_box_tpl)
print("123" in big_box_tpl)
print("Unknown" in big_box_tpl)
PythonOutput:
True
True
False
False
PlaintextSlicing a Tuple
big_box_tpl = ("First", "Second", "test", 123, 456, 789, "last")
print(big_box_tpl[0:4])
print(big_box_tpl[:4])
print(big_box_tpl[4:])
print(big_box_tpl[0:-1])
PythonOutput:
('First', 'Second', 'test', 123)
('First', 'Second', 'test', 123)
(456, 789, 'last')
('First', 'Second', 'test', 123, 456, 789)
PlaintextNamed Tuple
A named tuple is similar to a tuple. But in a named tuple, we can access the items by name(also by index).
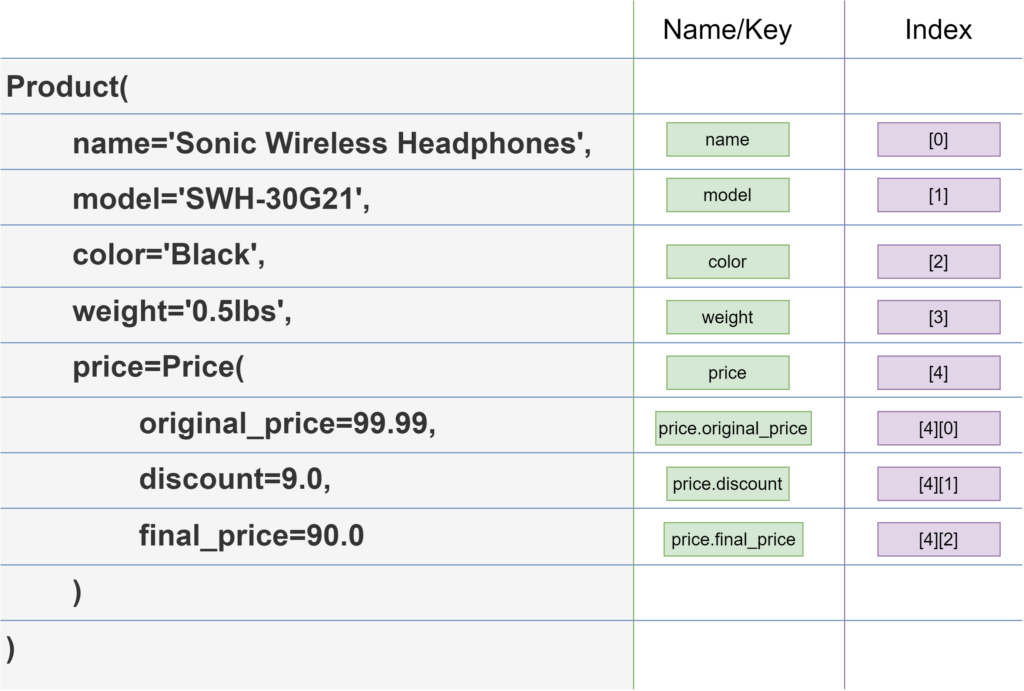
Use the “namedtuple” function from “collections” module, to create a named tuple. The following parameters are used to define the named tuple-
- First param – typename : name of the type
- Second param – field_names : list of field names separated by space
Here we are creating a named tuple with named “Product” which has fields – “name”, “mode”, “color”, “weight”.
import collections
Product = collections.namedtuple('Product', 'name model color weight')
product_one = Product("Sonic Wireless Headphones", "SWH-30G21", "Black", "0.5lbs")
print(Product)
print(product_one)
PythonOutput:
<class '__main__.Product'>
Product(name='Sonic Wireless Headphones', model='SWH-30G21', color='Black', weight='0.5lbs')
PlaintextWe can use any data type as a value for the fields. The field can be of another named tuple.
Here we have used “Price” as another named tuple, which defines product price with fields – “original_price”, “discount”, “final_price”.
import collections
Product = collections.namedtuple('Product', 'name model color weight price')
Price = collections.namedtuple('Price', 'original_price discount final_price')
product_one = Product("Sonic Wireless Headphones", "SWH-30G21", "Black", "0.5lbs", Price(99.99, 9.00, 90.00))
print(Product)
print(product_one)
PythonOutput:
<class '__main__.Product'>
Product(name='Sonic Wireless Headphones', model='SWH-30G21', color='Black', weight='0.5lbs', price=Price(original_price=99.99, discount=9.0, final_price=90.0))
PlaintextWe can access the fields from “Product” and from “Price” using the field names-
import collections
Product = collections.namedtuple('Product', 'name model color weight price')
Price = collections.namedtuple('Price', 'original_price discount final_price')
product_one = Product("Sonic Wireless Headphones", "SWH-30G21", "Black", "0.5lbs", Price(99.99, 9.00, 90.00))
print(product_one.name)
print(product_one.model)
print(product_one.price.final_price)
PythonOutput:
Sonic Wireless Headphones
SWH-30G21
90.0
PlaintextWe can also access those field using index of the field, like below-
import collections
Product = collections.namedtuple('Product', 'name model color weight price')
Price = collections.namedtuple('Price', 'original_price discount final_price')
product_one = Product("Sonic Wireless Headphones", "SWH-30G21", "Black", "0.5lbs", Price(99.99, 9.00, 90.00))
print(product_one[0])
print(product_one[1])
print(product_one[2])
print(product_one[3])
print(product_one[4])
print(product_one[4][0])
PythonOutput:
Sonic Wireless Headphones
SWH-30G21
Black
0.5lbs
Price(original_price=99.99, discount=9.0, final_price=90.0)
99.99
Plaintext