We can use a floating point number to represent a decimal number. In Python we can represent 3 types of floating values-
- Floating-point values
- Decimal values(using decimal.Decimal)
- Complex numbers
NOTES
All these 3 types are immutable.
That means when any of the above types of data is created in memory, it is never changed. Reassigning a new value to any of the above types, will create a new object and rebind the variable to the new object.
The old object remains as it is.
Float
Floating point numbers are represented by class “float” in python. As numbers are stored in binary format internally, that might cause some issue in accuracy for the floating point numbers.
Define Float
Use the function “float” to define a floating point number. The “float” function converts a string or number to a floating point number.
big_box_float = float(99.99)
print("big_box_float: ", big_box_float)
print("type of big_box_float: ", type(big_box_float))
second_float = float(500)
print("second_float: ", second_float)
print("type of second_float: ", type(second_float))
third_float = float(-33.5)
print("third_float: ", third_float)
print("type of third_float: ", type(third_float))
PythonOutput:
big_box_float: 99.99
type of big_box_float: <class 'float'>
second_float: 500.0
type of second_float: <class 'float'>
third_float: -33.5
type of third_float: <class 'float'>
PlaintextOr you can just assign a float value to a varialbe directly, and that will create a float object in memory.
big_box_float = 99.99
print("big_box_float: ", big_box_float)
print("type of big_box_float: ", type(big_box_float))
second_float = 500.58
print("second_float: ", second_float)
print("type of second_float: ", type(second_float))
PythonOutput:
big_box_float: 99.99
type of big_box_float: <class 'float'>
second_float: 500.58
type of second_float: <class 'float'>
PlaintextFloat min and max
The max and min values of float number, depends on the system(or the Python interpreter). Let’s check the system infomration related to float-
import sys
print(sys.float_info)
PythonOutput:
sys.float_info(max=1.7976931348623157e+308, max_exp=1024, max_10_exp=308, min=2.2250738585072014e-308, min_exp=-1021, min_10_exp=-307, dig=15, mant_dig=53, epsilon=2.220446049250313e-16, radix=2, rounds=1)
PlaintextFloat Reassignment
If reassign a new value to a float variable, then a new float object is created in memory and the new object is assigned to the variable.
import ctypes
big_box_float = 1000.50
first_id = id(big_box_float)
print("Initial id: ", first_id)
big_box_float = 2000.89
print("ID after change: ", id(big_box_float))
obj = ctypes.cast(first_id, ctypes.py_object)
print("Old float object: ", obj)
PythonOutput:
As we can see from the result, then old object stays in memory with the original value.
Initial id: 1513665479408
ID after change: 1513661831472
Old float object: py_object(1000.5)
PlaintextHere is how it looks like after reassging a variable-
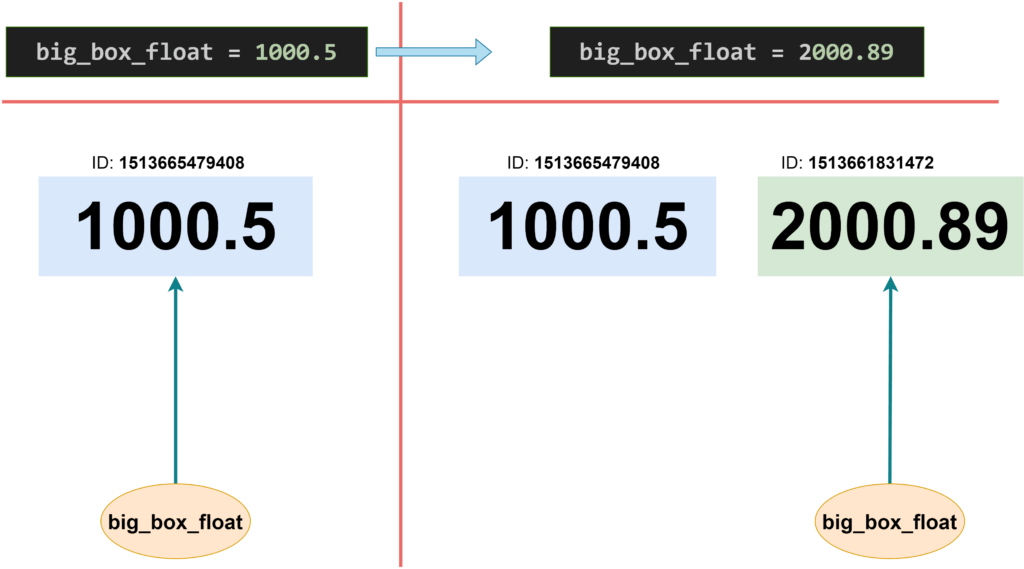
Float Utility
Here are some usage of float number, with some utility fuctions-
import math
big_box_float = 9.87
print("round(50.46) = ", round(50.56))
print("int(big_box_float) = ", int(big_box_float))
print("math.floor(big_box_float) = ", math.floor(big_box_float))
print("math.ceil(big_box_float) = ", math.ceil(big_box_float))
print("big_box_float.as_integer_ratio() = ", big_box_float.as_integer_ratio())
print("big_box_float.hex() = ", big_box_float.hex())
PythonOutput:
round(50.46) = 51
int(big_box_float) = 9
math.floor(big_box_float) = 9
math.ceil(big_box_float) = 10
big_box_float.as_integer_ratio() = (5556316040268349, 562949953421312)
big_box_float.hex() = 0x1.3bd70a3d70a3dp+3
PlaintextDecimal
Decimals also represent floating point numbers, but provided better accuracy compared to the float data type.
Using decimal affect the performance of the execution, but when we need better accuracy with the numbers, we should use Decimal.
Define Decimal
Use the “decimal” module to define a decimal-
import decimal
big_box_decimal = decimal.Decimal(1234)
print(big_box_decimal)
print(type(big_box_decimal))
big_box_decimal = decimal.Decimal(123456789.123456789)
print(big_box_decimal)
print(type(big_box_decimal))
PythonOutput:
1234
<class 'decimal.Decimal'>
123456789.12345679104328155517578125
<class 'decimal.Decimal'>
PlaintextDecimal Calculation
All mathematical calculations are supported. The result of the calcualtion will be also a decimal-
import decimal
first_num = decimal.Decimal(123.45)
second_num = decimal.Decimal(6789.11)
multiply_result = first_num * second_num
print("Multiplication result: ", multiply_result)
print("Type of multiplication result: ", type(multiply_result))
div_result = second_num / first_num
print("Division result: ", div_result)
print("Type of division result: ", type(div_result))
PythonOutput:
Multiplication result: 838115.6294999999788760476349
Type of multiplication result: <class 'decimal.Decimal'>
Division result: 54.99481571486431361909469517
Type of division result: <class 'decimal.Decimal'>
PlaintextWe can not peroform operation with mixed type of data. Python throws an error if we try to perform calcuation using a decimal and float-
import decimal
result = decimal.Decimal(45.33) + 3.5
print("Result: ", result)
print("Type of result: ", type(result))
PythonOutput:
Traceback (most recent call last):
File "dec_ex.py", line 3, in <module>
result = decimal.Decimal(45.33) + 3.5
TypeError: unsupported operand type(s) for +: 'decimal.Decimal' and 'float'
PlaintextComplex Numbers
Complex numbers have 2 parts – real and imaginary. These numbers are represented with combining the 2 parts with a Plus(+) or a Minus(-) sign. The imaginary part is denoted by adding a “j” with it, i.e. 9.87+.5j
Complex numbers in python are consist of a pair of floating point numbers. First part is for the real part, and the second part is for the iaginary part.
Define Complex Numbers
Use the complex function to create a a complex number in Python. The real and imaginary parts are provided as separate part-
big_box_complex = complex(99)
print(big_box_complex)
print(type(big_box_complex))
big_box_complex = complex(10.4, 2.3)
print(big_box_complex)
print(type(big_box_complex))
PythonOutput:
(99+0j)
<class 'complex'>
(10.4+2.3j)
<class 'complex'>
PlaintextWe can define a complex number by just assigin a number, with has “j” added for the imaginary part. Check the code below-
z = 9.87 + .5j
print(z)
print(type(z))
print("z real part: ", z.real)
print("z imaginary part: ", z.imag)
PythonOutput:
After definition we can separate the real and imaginary part and represent those –
(9.87+0.5j)
<class 'complex'>
z real part: 9.87
z imaginary part: 0.5
Plaintext