Operator | Description |
---|---|
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
// | Floor division |
** | Power |
% | Reminder |
Addition
a = 90
b = 23
x = a + 100
y = a + b
z = x + b
print(x)
print(y)
print(z)
PythonOutput:
190
113
213
PlaintextSubtraction
a = 90
b = 23
x = a - 100
y = a - b
z = x - b
print(x)
print(y)
print(z)
PythonOutput:
-10
67
-33
PlaintextMultiplication
a = 90
b = 23
x = a * 100
y = a * b
z = x * b
print(x)
print(y)
print(z)
PythonOutput:
9000
2070
207000
PlaintextDivision
a = 90
b = 23
x = a / 100
y = a / b
z = x / b
print(x)
print(y)
print(z)
PythonOutput:
0.9
3.9130434782608696
0.0391304347826087
PlaintextFloor Division
a = 90
b = 20
x = a // 10 # 90 / 10 = 9
y = a // b # 90 / 20 = 4.5
z = x // b # 9 / 20 = 0.45
print(x)
print(y)
print(z)
PythonOutput:
9
4
0
PlaintextReminder
a = 90
b = 23
x = a % 8
y = a % b
z = x % b
print(x)
print(y)
print(z)
PythonOutput:
2
21
2
PlaintextPower
a = 90
b = 23
x = a ** 3
y = a ** b
z = x ** b
print(x)
print(y)
print(z)
PythonOutput:
729000
886293811965250109592900000000000000000000000
696198609130885597695136021593547814689632716312296141651066450089000000000000000000000000000000000000000000000000000000000000000000000
PlaintextPrecedence of Mathematical Operators
Not all operators have the same precedence. Based on the operators’ priority/precedence, the evaluation sequence will change.
Let’s check the precedence of the operators and how that affects the evaluation of an expression-
Notes
Expressions are evaluated from Left to Right when they have the same precedence.
Let’s evaluate the following operation-
(3 + 7) * 24 / 2 ** 2 + 6
The operation will be performed as below-
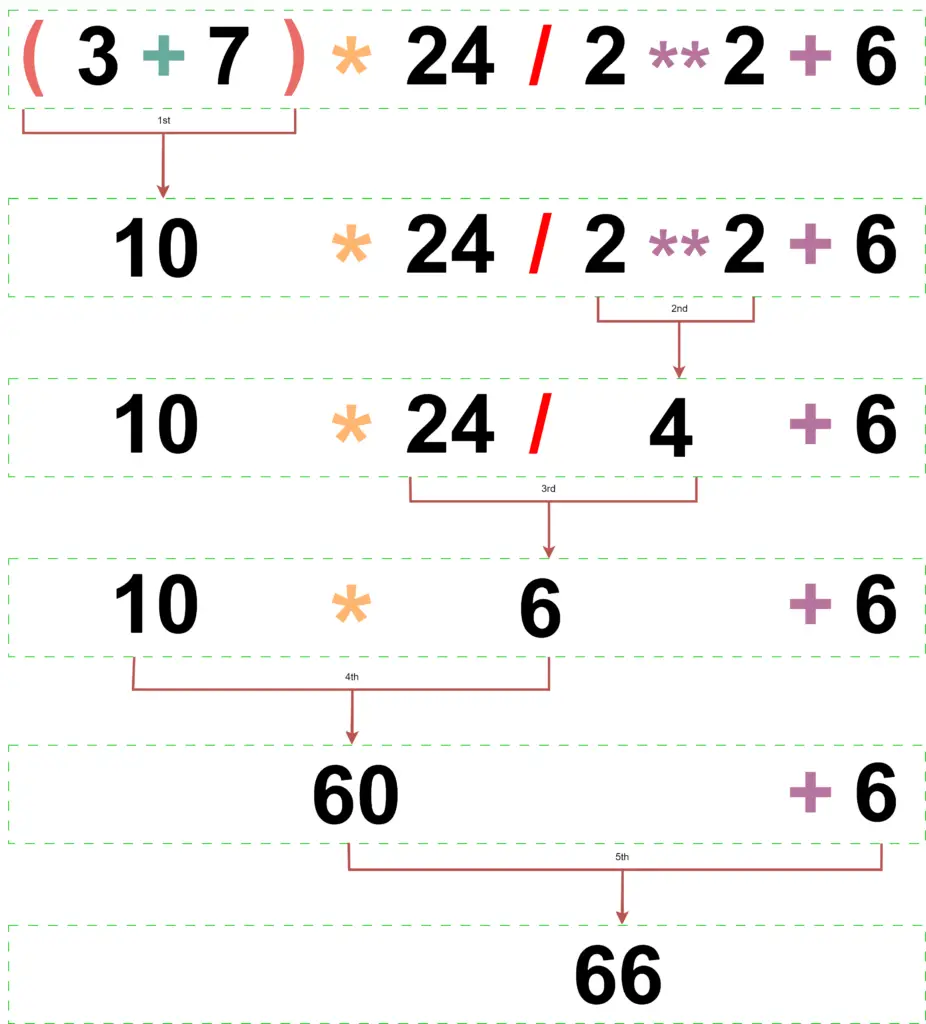
Let’s check the same thing in code-
calculated_value = (3 + 7) * 24 / 2 ** 2 + 6
# 10 * 24 / 2 ** 2 + 6
# 10 * 24 / 4 + 6
# 10 * 6 + 6
# 60 + 6
# 66
print(calculated_value)
PythonOutput:
66.0
PlaintextAugmented Assignment Operators
We can use the mathematical operators, perform the operation and assign at the same time, by using the following operator
Operator | Description | Example | Equivalent Normal Operation |
---|---|---|---|
+= | Add and assign | x += 10 | x = x + 10 |
-= | Subtract and assign | x -= 10 | x = x – 10 |
*= | Multiply and assign | x *= 10 | x = x * 10 |
/= | Divide and assign | x /= 10 | x = x / 10 |
//= | Floor divide and assign | x //= 10 | x = x // 10 |
**= | Exponenatiate and assign | x **= 10 | x = x ** 10 |
%= | Modulo and assign | x %= 10 | x = x % 10 |