List in Python is a data type that stores a collection of data, of zero or more types. Python list is mutable. Items in the list store the order of each item.
A Python list is mutable ordered collection of Zero or more Python object references.
A list can be sliced, iterated, or nested.
List Capabilities
Here is what a List in Python can and can’t do-
List can do
List can not do
WARNING
Python Lists might use more memory than the array implementation in other languages(like c, Java, etc.). We can use ‘numpy‘ arrays if needed, for better memory efficiency.
Indexing in a List is a constant time operation, but functions like ‘insert‘ and ‘remove‘ do not have const time performance.
Define a List
Use the function “list” to create a new list. We can items to the list after that-
big_box_list = list()
print("Type: ", type(big_box_list))
print("Length: ", len(big_box_list))
print(dir(big_box_list))
# Add items using append()
# big_box_list.append("one")
# big_box_list.append("two")
# big_box_list.append("three")
PythonOutput:
Type: <class 'list'>
Length: 0
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
PlaintextOr we can define a list by using square brackets, and putting the items separated by command-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
print("Type: ", type(big_box_list))
print("Length: ", len(big_box_list))
print(dir(big_box_list))
PythonOutput:
Type: <class 'list'>
Length: 7
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
PlaintextObject Referencing
Internally, the values are not saved in a List. When a value is added/appended to a list, the value is stored in memory separately with initialization, and the reference of the object is saved in the List.
Here is how the referencing looks under the hood-
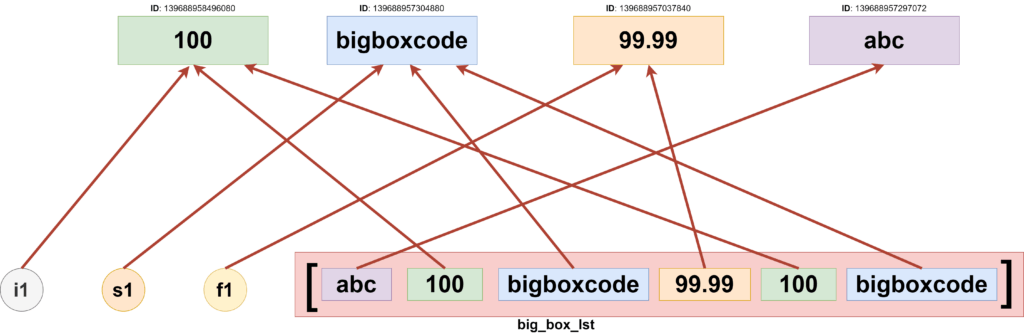
Check the example below-
i1 = 100
s1 = 'bigboxcode'
f1 = 99.99
print(f"i1 => value: {i1} || type: {type(i1)} || id: {id(i1)}")
print(f"s1 => value: {s1} || type: {type(s1)} || id: {id(s1)}")
print(f"f1 => value: {f1} || type: {type(f1)} || id: {id(f1)}")
big_box_lst = ["abc", 100, "bigboxcode", 99.99, 100, "bigboxcode"]
print(f"big_box_lst[0] => value: {big_box_lst[0]} || type: {type(big_box_lst[0])} || id: {id(big_box_lst[0])}")
print(f"big_box_lst[1] => value: {big_box_lst[1]} || type: {type(big_box_lst[1])} || id: {id(big_box_lst[1])}")
print(f"big_box_lst[2] => value: {big_box_lst[2]} || type: {type(big_box_lst[2])} || id: {id(big_box_lst[2])}")
print(f"big_box_lst[3] => value: {big_box_lst[3]} || type: {type(big_box_lst[3])} || id: {id(big_box_lst[3])}")
print(f"big_box_lst[3] => value: {big_box_lst[4]} || type: {type(big_box_lst[4])} || id: {id(big_box_lst[4])}")
print(f"big_box_lst[3] => value: {big_box_lst[5]} || type: {type(big_box_lst[5])} || id: {id(big_box_lst[5])}")
PythonOutput:
List items refer to the same object, as those individually defined object-
i1 => value: 100 || type: <class 'int'> || id: 139688958496080
s1 => value: bigboxcode || type: <class 'str'> || id: 139688957304880
f1 => value: 99.99 || type: <class 'float'> || id: 139688957037840
big_box_lst[0] => value: abc || type: <class 'str'> || id: 139688957297072
big_box_lst[1] => value: 100 || type: <class 'int'> || id: 139688958496080
big_box_lst[2] => value: bigboxcode || type: <class 'str'> || id: 139688957304880
big_box_lst[3] => value: 99.99 || type: <class 'float'> || id: 139688957037840
big_box_lst[3] => value: 100 || type: <class 'int'> || id: 139688958496080
big_box_lst[3] => value: bigboxcode || type: <class 'str'> || id: 139688957304880
PlaintextItem Index in List
Index in a list starts from zero(0). And item at a certain index can be accessed by using “list_name[index]”.
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
print(big_box_list[0])
print(big_box_list[4])
print(big_box_list[6])
print(big_box_list[-1])
PythonOutput:
First item
5
Last Item here
Last Item here
PlaintextChange List Item
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
big_box_list[0] = 11
big_box_list[3] = "Changed"
print(big_box_list)
PythonOutput:
[11, 'Second item', 'Third item', 'Changed', 5, 100, 'Last Item here']
PlaintextAdd Item to List
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
big_box_list.append("New item here")
big_box_list.append("Second new item")
print(big_box_list)
PythonOutput:
['First item', 'Second item', 'Third item', 4, 5, 100, 'Last Item here', 'New item here', 'Second new item']
PlaintextCheck Length of List
Use the “len()” function to get the length of a list-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
list_length = len(big_box_list)
print(list_length)
PythonOutput:
7
PlaintextCheck Membership
We can check the membership of an item in a list using “in”. Both “in” and “not in” can be used, like below-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
print("4 in big_box_list: ", 4 in big_box_list)
print("\"First item\" in big_box_list: ", "First item" in big_box_list)
print("\"Unknown Item\" in big_box_list: ", "Unknown Item" in big_box_list)
print("4 not in big_box_list: ", 4 in big_box_list)
print("\"First item\" not in big_box_list: ", "First item" not in big_box_list)
print("\"Unknown Item\" not in big_box_list: ", "Unknown Item" not in big_box_list)
PythonOutput:
4 in big_box_list: True
"First item" in big_box_list: True
"Unknown Item" in big_box_list: False
4 not in big_box_list: True
"First item" not in big_box_list: False
"Unknown Item" not in big_box_list: True
PlaintextConcat Lists
We can concat 2 or more lists, using the “+” operator-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
second_list = [99, 999, 99.99, 9090]
print(big_box_list + second_list)
PythonOutput:
['First item', 'Second item', 'Third item', 4, 5, 100, 'Last Item here', 99, 999, 99.99, 9090]
PlaintextSlicing a List
We can get a certain portion of a list by slicing it-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
print(big_box_list[0:3])
print(big_box_list[:3])
print(big_box_list[2:7])
print(big_box_list[:-1])
PythonOutput:
['First item', 'Second item', 'Third item']
['First item', 'Second item', 'Third item']
['Third item', 4, 5, 100, 'Last Item here']
['First item', 'Second item', 'Third item', 4, 5, 100]
PlaintextCheck Length of List
Use the “len()” function to get the length of a list-
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
list_length = len(big_box_list)
print(list_length)
PythonOutput:
7
PlaintextLoop through a List
Case #1:
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
for item in big_box_list:
print(item)
PythonOutput:
First item
Second item
Third item
4
5
100
Last Item here
PlaintextCase #2:
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
for index in range(len(big_box_list)):
print(index, big_box_list[index])
PythonOutput:
0 First item
1 Second item
2 Third item
3 4
4 5
5 100
6 Last Item here
PlaintextCase #3: Using while Loop
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
# Start from index 0
item_index = 0
while item_index < len(big_box_list):
print(f"Index: {item_index} || Item value: {big_box_list[item_index]}")
# Increase the index by 1
item_index += 1
PythonOutput:
Index: 0 || Item value: First item
Index: 1 || Item value: Second item
Index: 2 || Item value: Third item
Index: 3 || Item value: 4
Index: 4 || Item value: 5
Index: 5 || Item value: 100
Index: 6 || Item value: Last Item here
PlaintextCase #4: Using enumerate With for Loop
big_box_list = ["First item", "Second item", "Third item", 4, 5, 100, "Last Item here"]
for key, value in enumerate(big_box_list):
print(f"Key: {key} || Value: {value}")
PythonOutput:
Key: 0 || Value: First item
Key: 1 || Value: Second item
Key: 2 || Value: Third item
Key: 3 || Value: 4
Key: 4 || Value: 5
Key: 5 || Value: 100
Key: 6 || Value: Last Item here
Plaintext