Integer in Python represents whole numbers, without any fractional part. An integer can be positive, negative, or zero.
NOTES
Max size of an integer in Python depends on the memory of the machine.
Integers can be very large in Python, though working with a very large integer will be very slow.
If the integer in Python fits the integer size of the processor, then the processing will be much faster (relative to a large-size integer).
Create Integer
Create an integer by using the “int” function. If no value is provided to the “int” function then the default value is 0(Zero).
We can provide a value to the “int” function, and that will be the value of the integer-
big_box_int = int()
print("big_box_int: ", big_box_int)
print("type of big_box_int: ", type(big_box_int))
second_val = int(100)
print("second_val: ", second_val)
print("type of second_val: ", type(second_val))
third_val = int(-50)
print("third_val: ", third_val)
print("type of third_val: ", type(third_val))
PythonOutput:
big_box_int: 0
type of big_box_int: <class 'int'>
second_val: 100
type of second_val: <class 'int'>
third_val: -50
type of third_val: <class 'int'>
PlaintextOr we can just assign the value directly to the variable-
big_box_int = 100
print("big_box_int: ", big_box_int)
print("type of big_box_int: ", type(big_box_int))
second_val = -50
print("second_val: ", second_val)
print("type of second_val: ", type(second_val))
PythonOutput:
big_box_int: 100
type of big_box_int: <class 'int'>
second_val: -50
type of second_val: <class 'int'>
PlaintextInteger Reassignment Internals
import ctypes
big_box_int = 1000
first_id = id(big_box_int)
print("Initial id: ", first_id)
big_box_int = 2000
print("ID after change: ", id(big_box_int))
obj = ctypes.cast(first_id, ctypes.py_object)
print("Old integer object: ", obj)
PythonOutput:
Initial id: 2268869335344
ID after change: 2268872852048
Old integer object: py_object(1000)
Plaintext NOTES
Integers in Python are immutable internally. The object which is created internally for the integer, is never changed.
But we don’t notice it because of the assignment operator. When a new value is assigned to the same integer variable, a new integer object is created and the variable is pointed to that new object.
In the meantime, the old integer object remains as it is.
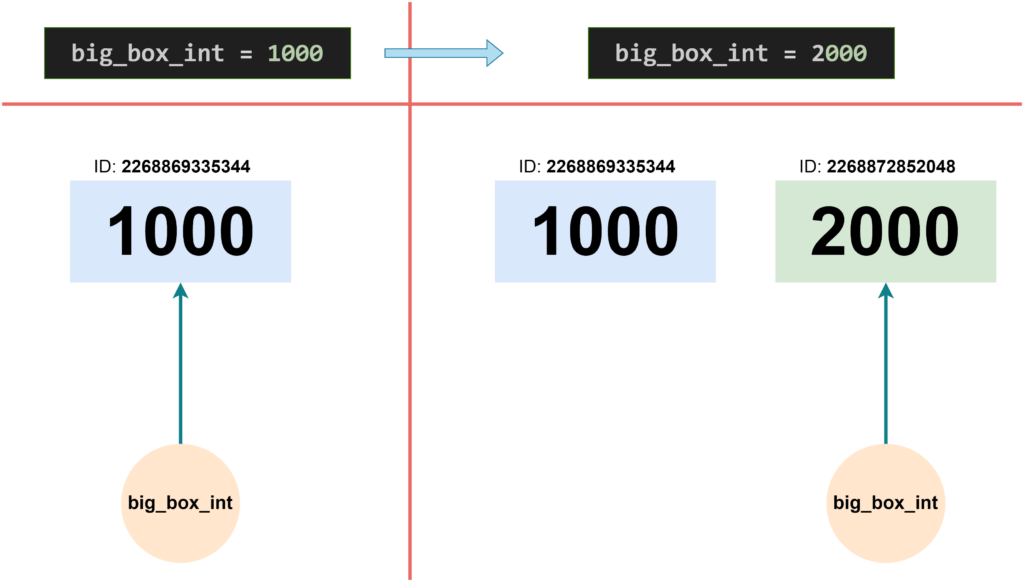
Integer with Different Base
By default, the integers are 10-based. We can write integers with different bases using different prefixes-
Name | Base | Starts With (Note: the followings start with 0) | Example (All represent 1000) |
---|---|---|---|
Decimal | 10 | 1000 | |
Binary | 2 | 0b | 0b1111101000 |
Octal | 8 | 0o | 0o1750 |
Hexadecimal | 16 | 0x | 0x3E8 |
Check the code below for representing numbers indifferent base-
big_box_int = 1000
big_box_binary = 0b1111101000
big_box_hex = 0x3E8
big_box_oct = 0o1750
print("Integer: ", big_box_int)
print("From Binary: ", big_box_binary)
print("From Hex: ", big_box_hex)
print("From Octal: ", big_box_oct)
PythonOutput:
When we print with the “print” function it prints the decimal value(no matter what format we provided to it)-
Integer: 1000
From Binary: 1000
From Hex: 1000
From Octal: 1000
PlaintextBase Conversion
We can convert an integer(10-based) to a binary, hex or octal by using the relevant functions. Check the example below.
print("Binary representation: ", bin(1000))
print("Hex representation: ", hex(1000))
print("Oct representation: ", oct(1000))
PythonOutput:
Binary representation: 0b1111101000
Hex representation: 0x3e8
Oct representation: 0o1750
PlaintextWe can also convert a string or any other type of data to an integer by using the “int” function. We can also provide the base for the “int” function.
print("Integer conversion: ", int("1000"))
print("Integer conversion with base 10: ", int("1000", 10))
print("Integer conversion with base 2: ", int("1000", 2))
print("Integer conversion with base 3: ", int("1000", 3))
print("Integer conversion with base 8: ", int("1000", 8))
PythonOutput:
Integer conversion: 1000
Integer conversion with base 10: 1000
Integer conversion with base 2: 8
Integer conversion with base 3: 27
Integer conversion with base 8: 512
Plaintext