Flyweight pattern is used to reduce the number of objects to save memory and optimize resources.
This article demonstrates Flyweight pattern implementations in Java. Check the following examples.
Examples
Here are a few examples of the Flyweight Pattern implementation in Java-
Example #1: Table Cells
Let’s take a look at how we can use the Flyweight pattern to reduce memory usage while generating thousands of table cells.
Class Diagram
Take a look at the class diagram. Two classes are used for Flyweight pattern implementation, TableCell and TableCellFactory.
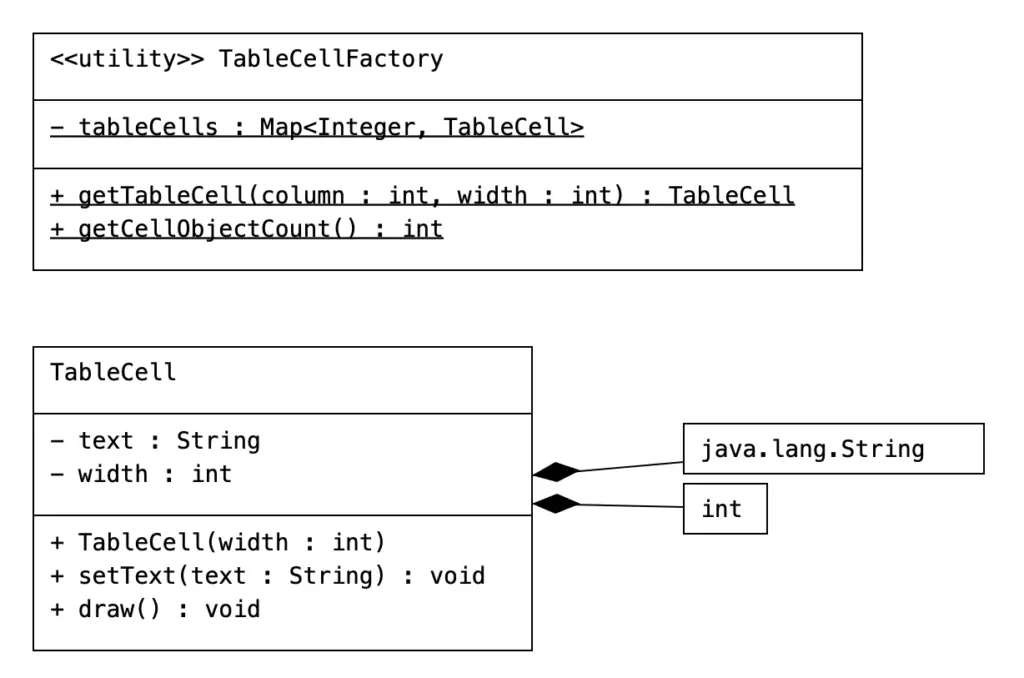
TableCell Class
Let’s create a class for printing a table cell. it has property width and text. For all cells of a column, the width will be the same, so we are setting the width in the constructor.
Text will be different for each cell, that’s why we are creating a separate method setText. This way the text can be set just before printing the cell. Finally, there is a method for drawing the cell.
This class will not be used directly. It will be used through a Factory, which is described next.
// TableCell.java
package com.bigboxcode.flyweight;
public class TableCell {
private int width;
private String text;
public TableCell(int width) {
this.width = width;
}
public void setText(String text) {
this.text = text;
}
public void draw() {
System.out.println("Drawing cell : width = " + width + " | text = " + text);
}
}
TableCellFactory Class
This factory class is used to generate the objects when required.
A hashmap is used to store the already generated objects. As all cells of a column will have the same width, so we are keeping the column number as the key of the HashMap. This way the factory will generate one object for all cells of a column.
The HashMap is made static so that it can hold the list of all generated objects. In the getTableCell method at first, we are checking if any object exists for a column. If an object exists then return it, else generate a new object for that column and return it.
// TableCellFactory.java
package com.bigboxcode.flyweight;
import java.util.HashMap;
import java.util.Map;
public class TableCellFactory {
private static Map<Integer, TableCell> tableCells = new HashMap<>();
public static TableCell getTableCell(int column, int width) {
TableCell tableCell = tableCells.get(column);
if (null != tableCell) return tableCell;
tableCell = new TableCell(width);
tableCells.put(column, tableCell);
return tableCell;
}
// For demo purpose only, not required in actual implementation
public static int getCellObjectCount() {
return tableCells.size();
}
}
Demo
Use the TableCellFactory where the functionality needs to be implemented.
First, we are using the factory to generate(or get existing) an object. Before drawing the cell we are setting the text for that specific cell.
For this example, we are printing 1000 rows and for each row, there will be 5 columns. So if we generate an object for each table cell then it will be 5000 objects in total.
But as we are using Flyweight pattern, and generating a single object for each column so here we will have only 5 objects of TableCell.
// Main.java
package com.bigboxcode.flyweight;
public class Main {
public static void main(String[] args) {
// There are 5 columns with width 3, 6, 2, 5, 10 of some standard unit
int[] columnWidths = {3, 6, 2, 5, 10};
// Print 1000 rows
for (int row = 0; row < 1000; row++) {
for (int column = 0; column < columnWidths.length; column++) {
TableCell tableCell = TableCellFactory.getTableCell(column, columnWidths[column]);
tableCell.setText(row + "-" + column); // For demo purpose, text can come from any other sources
tableCell.draw();
}
}
System.out.println("Total number of tree objects: " + TableCellFactory.getCellObjectCount());
}
}
Output
The output of the demo above will be like below. It will print 5000 cell data, and then finally we will see that the total number of the generated object is 5.
Drawing cell : width = 3 | text = 0-0
Drawing cell : width = 6 | text = 0-1
Drawing cell : width = 2 | text = 0-2
Drawing cell : width = 5 | text = 0-3
Drawing cell : width = 10 | text = 0-4
Drawing cell : width = 3 | text = 1-0
Drawing cell : width = 6 | text = 1-1
Drawing cell : width = 2 | text = 1-2
Drawing cell : width = 5 | text = 1-3
Drawing cell : width = 10 | text = 1-4
Drawing cell : width = 3 | text = 2-0
Drawing cell : width = 6 | text = 2-1
Drawing cell : width = 2 | text = 2-2
Drawing cell : width = 5 | text = 2-3
Drawing cell : width = 10 | text = 2-4
Drawing cell : width = 3 | text = 3-0
Drawing cell : width = 6 | text = 3-1
.
.
.
...// more output lines like this
.
.
.
.
Total number of tree objects: 5
Source Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: Table Cells | ![]() |
Other Code Implementations
Use the following links to check Flyweight pattern implementation in other programming languages.