Mediator pattern is used to reduce the complexity of communication between objects. As all the communication between objects goes through a mediator/middleman, that’s why the objects are not aware of the underlying complexity.
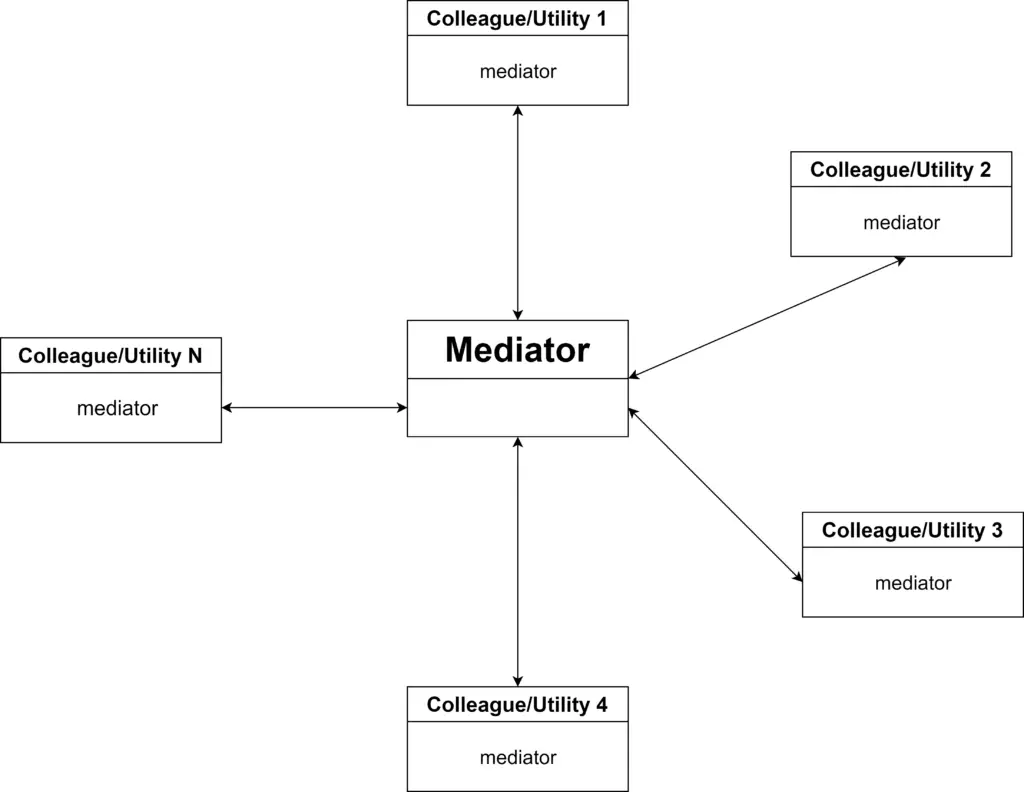
This article demonstrates Mediator pattern implementations in TypeScript. Check the following examples.
Implementation
Use the following steps to implement Mediator pattern in TypeScript.
- Create an interface for mediator.
- Create a mediator class and implement the interface.
- Create an abstract for the colleagues. Define a property for mediator. In the constructor set the mediator. Declare a few abstract methods for the communication between colleague objects.
- Define colleague classes which will communicate through the mediator. Extend the abstract mediator class. In the abstract method implementation accept another colleague object, and use the mediator when required.
Examples
Let’s take a look at a few examples of the Mediator pattern implementation in TypeScript.
Example #1: General Mediator
Let’s consider a general example.
Mediator [Interface and Class]
- Create file “imediator.ts”.
- Define a mediator interface named “IMediator”. Declare method named “sendMessage”, this accepts a colleague and a message as parameter.
- Define class “Mediator”.
- Implement the “IMediator” interface for “Mediator” class.
// mediator.ts
import Colleague from "./colleague";
interface IMediator {
sendMessage(colleague: Colleague, msg: string): void;
}
class Mediator implements IMediator {
sendMessage(receiver: Colleague, msg: string): void {
receiver.receiveMessage(msg);
}
}
export default Mediator;
Colleague Abstract Class
- Create file “colleague.ts”.
- Create abstract class “Colleague”.
- Define protected property “mediator” of type “Mediator”.
- In the constructor set the mediator.
- Declare abstract methods – “sendMessage” and “receiveMessage”.
// colleague.ts
import Mediator from "./mediator";
abstract class Colleague {
protected mediator: Mediator;
constructor(mediator: Mediator) {
this.mediator = mediator;
}
abstract sendMessage(colleague: Colleague, message: string): void;
abstract receiveMessage(message: string): void;
}
export default Colleague;
Colleague1 Class
- Create file “colleague1.ts”.
- Define class “Colleague1”.
- Extend the abstract class “Colleague” for class “Colleague1”.
- In the constructor accept a mediator and call the parent constructor.
- Define method “sendMessage”. Calll the “sendMessage” method from the mediator.
- Define method “receiveMessage” for the class.
// colleague1.ts
import Colleague from "./colleague";
import Mediator from "./mediator";
class Colleague1 extends Colleague {
constructor(mediator: Mediator) {
super(mediator);
}
sendMessage(colleague: Colleague, msg: string): void {
this.mediator.sendMessage(colleague, msg);
}
receiveMessage(msg: string): void {
console.log("Message received in Colleague1: " + msg);
}
}
export default Colleague1;
Colleague2 Class
- Create file “colleague2.ts”.
- Define class “Colleague2”.
- Extend the abstract class “Colleague”.
- Define method “sendMessage” and “receiveMessage”.
// colleague2.ts
import Colleague from "./colleague";
import Mediator from "./mediator";
class Colleague2 extends Colleague {
constructor(mediator: Mediator) {
super(mediator);
}
sendMessage(colleague: Colleague, msg: string): void {
this.mediator.sendMessage(colleague, msg);
}
receiveMessage(msg: string): void {
console.log("Message received in Colleague2: " + msg);
}
}
export default Colleague2;
Colleague3 Class
- Create file “colleague3.ts”.
- Define class “Colleague3” and extend the “Colleague” class for this. Define method “sendMessage” and “receiveMessage”.
// colleague3.ts
import Colleague from "./colleague";
import Mediator from "./mediator";
class Colleague3 extends Colleague {
constructor(mediator: Mediator) {
super(mediator);
}
sendMessage(colleague: Colleague, msg: string): void {
this.mediator.sendMessage(colleague, msg);
}
receiveMessage(msg: string): void {
console.log("Message received in Colleague3: " + msg);
}
}
export default Colleague3;
Demo
To use the implementation-
- Create a mediator object.
- Create colleague objects.
- Call the “sendMessage” of the colleague. Pass another colleague to the method so that message can be sent to that colleague.
// demo.ts
import Colleague1 from "./colleague1";
import Colleague2 from "./colleague2";
import Colleague3 from "./colleague3";
import Mediator from "./mediator";
const mediator = new Mediator();
const colleague1 = new Colleague1(mediator);
const colleague2 = new Colleague2(mediator);
const colleague3 = new Colleague3(mediator);
colleague1.sendMessage(colleague2, "message from colleague1");
colleague1.sendMessage(colleague3, "message from colleague1");
colleague2.sendMessage(colleague3, "message from colleague2");
colleague3.sendMessage(colleague1, "message from colleague3");
Output
Output of the above demo code will be as below-
Message received in Colleague2: message from colleague1
Message received in Colleague3: message from colleague1
Message received in Colleague3: message from colleague2
Message received in Colleague1: message from colleague3
Source Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: General Mediator | ![]() |
Other Code Implementations
Use the following links to check Mediator pattern implementation in other programming languages.