Strategy pattern is used when there are multiple algorithms available for the same purpose, and we need to decide between those algorithms. Strategy pattern helps to decide the algorithm and return the generated object to be used.
This article demonstrates Strategy pattern implementations in Java. Check the following examples.
Examples
Here are some examples of Strategy pattern implementation-
Example #1: Transport
Let’s take the example of a transport system. Take a look at class diagram.
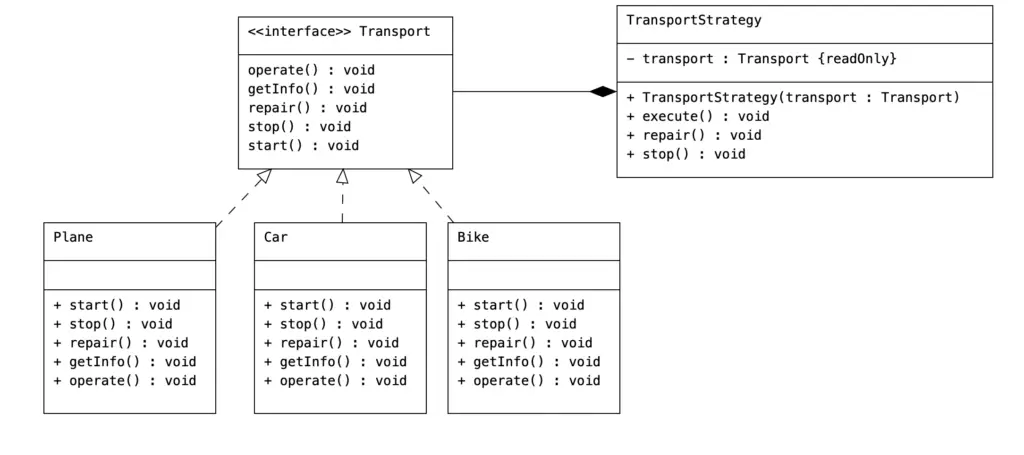
Transport Interface
// Transport.java
package com.bigboxcode;
public interface Transport {
void start();
void stop();
void repair();
void getInfo();
void operate();
}
JavaTransport Implementation for Bike
// Bike.java
package com.bigboxcode;
public class Bike implements Transport{
@Override
public void start() {
System.out.println("Bike started");
}
@Override
public void stop() {
System.out.println("Bike stopped");
}
@Override
public void repair() {
System.out.println("Bike repair");
}
@Override
public void getInfo() {
System.out.println("Transport type: Bike");
System.out.println("Number of wheels: 2");
System.out.println("Average Weight: 700 Pounds");
}
@Override
public void operate() {
System.out.println("Riding Bike ............");
}
}
JavaTransport Implementation for Car
// Car.java
package com.bigboxcode;
public class Car implements Transport{
@Override
public void start() {
System.out.println("Car started");
}
@Override
public void stop() {
System.out.println("Car stopped");
}
@Override
public void repair() {
System.out.println("Car repair");
}
@Override
public void getInfo() {
System.out.println("Transport type: Car");
System.out.println("Number of wheels: 4");
System.out.println("Average Weight: 4,000 Pounds");
}
@Override
public void operate() {
System.out.println("Driving car ............");
}
}
JavaTransport Implementation for Plane
// Plane.java
package com.bigboxcode;
public class Plane implements Transport{
@Override
public void start() {
System.out.println("Plane started");
}
@Override
public void stop() {
System.out.println("Plane stopped");
}
@Override
public void repair() {
System.out.println("Plane repair");
}
@Override
public void getInfo() {
System.out.println("Transport type: Plane");
System.out.println("Number of wheels: 3");
System.out.println("Average Weight: 50,000 Pounds");
}
@Override
public void operate() {
System.out.println("Flying plane ............");
}
}
JavaTransport Strategy Class
// TransportStrategy.java
package com.bigboxcode;
public class TransportStrategy {
private final Transport transport;
public TransportStrategy(Transport transport) {
this.transport = transport;
}
public void execute() {
this.transport.start();
this.transport.getInfo();
this.transport.operate();
}
public void repair() {
this.transport.repair();
}
public void stop() {
this.transport.stop();
}
}
JavaDemo
// Main.java
package com.bigboxcode;
public class Main {
public static void main(String[] args) {
// Use bike
System.out.println("Operating Bike:");
TransportStrategy myTransport = new TransportStrategy(new Bike());
myTransport.execute();
myTransport.stop();
// Use car
System.out.println("nnOperating Car:");
myTransport = new TransportStrategy(new Car());
myTransport.execute();
myTransport.stop();
myTransport.repair();
// Use plane
System.out.println("nnOperating plane:");
myTransport = new TransportStrategy(new Plane());
myTransport.execute();
myTransport.stop();
}
}
JavaOutput
Operating Bike:
Bike started
Transport type: Bike
Number of wheels: 2
Average Weight: 700 Pounds
Riding Bike ............
Bike stopped
Operating Car:
Car started
Transport type: Car
Number of wheels: 4
Average Weight: 4,000 Pounds
Driving car ............
Car stopped
Car repair
Operating plane:
Plane started
Transport type: Plane
Number of wheels: 3
Average Weight: 50,000 Pounds
Flying plane ............
Plane stopped
PlaintextExample #2: Mathematical Calculations
Let’s take a look at another example. In this example, we will demonstrate how Strategy pattern can be used while developing functionality for a calculator. we are just considering basic calculations here, the same steps can be used to implement more complex calculations.
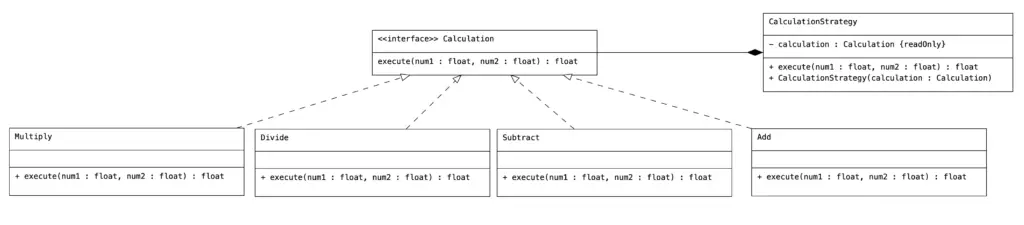
Calculation Interface
// Calculation.java
package com.BigBoxCode;
public interface Calculation {
float execute(float num1, float num2);
}
JavaCalculation Implementation for Add
// Add.java
package com.BigBoxCode;
public class Add implements Calculation {
@Override
public float execute(float num1, float num2) {
return num1 + num2;
}
}
JavaCalculation Implementation for Subtract
// Subtract.java
package com.BigBoxCode;
public class Subtract implements Calculation {
@Override
public float execute(float num1, float num2) {
return num1 - num2;
}
}
JavaCalculation Implementation for Multiply
// Multiply.java
package com.BigBoxCode;
public class Multiply implements Calculation {
@Override
public float execute(float num1, float num2) {
return num1 * num2;
}
}
JavaCalculation Implementation for Divide
// Divide.java
package com.BigBoxCode;
public class Divide implements Calculation {
@Override
public float execute(float num1, float num2) {
return num1 / num2;
}
}
JavaCalculation Strategy Class
// CalculationStrategy.java
package com.BigBoxCode;
public class CalculationStrategy {
private final Calculation calculation;
public CalculationStrategy(Calculation calculation) {
this.calculation = calculation;
}
public float execute(float num1, float num2) {
return this.calculation.execute(num1, num2);
}
}
JavaDemo
// Main.java
package com.BigBoxCode;
public class Main {
public static void main(String[] args) {
// Perform Addition
System.out.println("Performing operation: ADD");
CalculationStrategy myCalculation = new CalculationStrategy(new Add());
float result = myCalculation.execute(10, 5);
System.out.println("10 + 5 = " + result);
// Perform Subtraction
System.out.println("nnPerforming operation: SUBTRACT");
myCalculation = new CalculationStrategy(new Subtract());
result = myCalculation.execute(10, 5);
System.out.println("10 - 5 = " + result);
// Perform Multiplication
System.out.println("nnPerforming operation: MULTIPLY");
myCalculation = new CalculationStrategy(new Multiply());
result = myCalculation.execute(10, 5);
System.out.println("10 * 5 = " + result);
// Perform Division
System.out.println("nnPerforming operation: DIVIDE");
myCalculation = new CalculationStrategy(new Divide());
result = myCalculation.execute(10, 5);
System.out.println("10 / 5 = " + result);
}
}
JavaResult
Performing operation: ADD
10 + 5 = 15.0
Performing operation: SUBTRACT
10 - 5 = 5.0
Performing operation: MULTIPLY
10 * 5 = 50.0
Performing operation: DIVIDE
10 / 5 = 2.0
PlaintextExample #3: File Storage
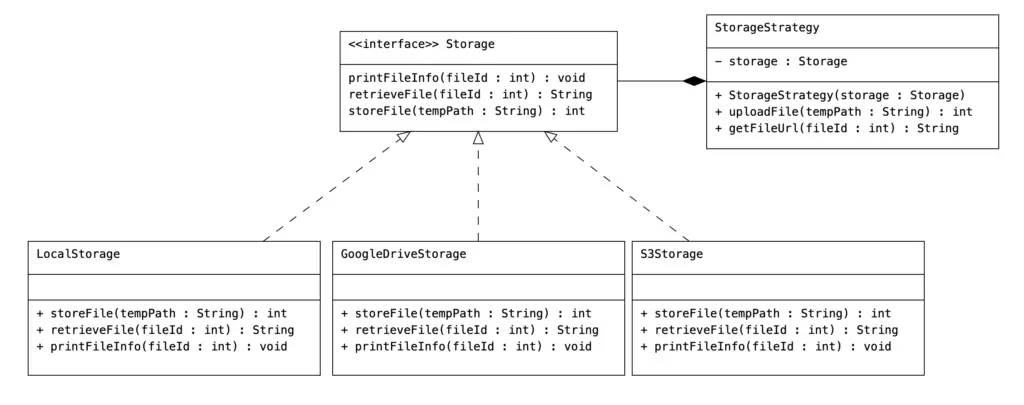
Define Storage Interface
// Storage.java
package com.bigboxcode;
public interface Storage {
int storeFile(String tempPath);
String retrieveFile(int fileId);
void printFileInfo(int fileId);
}
JavaImplement Storage Interface for Local Storage
// LocalStorage.java
package com.bigboxcode;
import java.util.Random;
public class LocalStorage implements Storage {
@Override
public int storeFile(String tempPath) {
// Code to store file here
// return the ID that is obtained from the database record or any other unique identifier.
// For demo purpose a random number is returned here
return (new Random()).nextInt(100);
}
@Override
public String retrieveFile(int fileId) {
// Retrieve the url and return back
// Some dummy url is returned for demo purpose
return "https://bigboxcode.com/files/local/" + fileId;
}
@Override
public void printFileInfo(int fileId) {
System.out.println("Storage type: Local Storage");
System.out.println("File ID: " + fileId);
System.out.println("File URL: " + this.retrieveFile(fileId));
}
}
JavaImplement Storage Interface for Google Drive
// GoogleDriveStorage.java
package com.bigboxcode;
import java.util.Random;
public class GoogleDriveStorage implements Storage {
@Override
public int storeFile(String tempPath) {
// Code to store file here
// return the ID that is obtained from the database record or any other unique identifier.
// For demo purpose a random number is returned here
return (new Random()).nextInt(1000);
}
@Override
public String retrieveFile(int fileId) {
// Retrieve the url and return back
// Some dummy url is returned for demo purpose
return "https://drive.google.com/file/d/1234_9K7654hu6RT_9j7JKY3fK/view";
}
@Override
public void printFileInfo(int fileId) {
System.out.println("Storage type: Google Drive");
System.out.println("File ID: " + fileId);
System.out.println("File URL: " + this.retrieveFile(fileId));
}
}
JavaImplement Storage Interface for AWS S3
// S3Storage.java
package com.bigboxcode;
import java.util.Random;
public class S3Storage implements Storage {
@Override
public int storeFile(String tempPath) {
// Code to store file here
// return the ID that is obtained from the database record or any other unique identifier.
// For demo purpose a random number is returned here
return (new Random()).nextInt(10000);
}
@Override
public String retrieveFile(int fileId) {
// Retrieve the url and return back
// Some dummy url is returned for demo purpose
return "https://bigboxcode.s3.amazonaws.com/pdf/UC-0e7654338-5697-4f99-b33-d89h87g5gf4gwfg.pdf";
}
@Override
public void printFileInfo(int fileId) {
System.out.println("Storage type: AWS S3");
System.out.println("File ID: " + fileId);
System.out.println("File URL: " + this.retrieveFile(fileId));
}
}
JavaStorage Strategy Class
// StorageStrategy.java
package com.bigboxcode;
public class StorageStrategy {
private Storage storage;
public StorageStrategy(Storage storage) {
this.storage = storage;
}
public int uploadFile(String tempPath) {
int fileId = this.storage.storeFile(tempPath);
this.storage.printFileInfo(fileId);
return fileId;
}
public String getFileUrl(int fileId) {
return this.storage.retrieveFile(fileId);
}
}
JavaDemo
// Main.java
package com.bigboxcode;
public class Main {
public static void main(String[] args) {
// Use Local storage
System.out.println("Using local storage:");
StorageStrategy fileStorage = new StorageStrategy(new LocalStorage());
fileStorage.uploadFile("/some-temp-path");
// Use car
System.out.println("nnUsing AWS S3:");
fileStorage = new StorageStrategy(new S3Storage());
fileStorage.uploadFile("/some-temp-path");
// Use plane
System.out.println("nnUsing Google Drive:");
fileStorage = new StorageStrategy(new GoogleDriveStorage());
fileStorage.uploadFile("/some-temp-path");
}
}
JavaOutput
Using local storage:
Storage type: Local Storage
File ID: 98
File URL: https://bigboxcode.com/files/local/98
Using AWS S3:
Storage type: AWS S3
File ID: 4905
File URL: https://bigboxcode.s3.amazonaws.com/pdf/UC-0e7654338-5697-4f99-b33-d89h87g5gf4gwfg.pdf
Using Google Drive:
Storage type: Google Drive
File ID: 736
File URL: https://drive.google.com/file/d/1234_9K7654hu6RT_9j7JKY3fK/view
PlaintextSource Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: Transport | ![]() |
Example #2: Mathematical Calculations | ![]() |
Example #3: File Storage | ![]() |
Other Code Implementations
Use the following links to check Strategy pattern implementation in other programming languages.