Bridge pattern is used to decouple implementation and abstraction.
NOTES
In this article, we discuss the implementation of the Bridge Pattern in Java.
See the Bridge in other languages in the “Other Code Implementations” section. Or, use the link below to check the details of the Bridge Pattern-
Examples
Here are some examples of Bridge pattern implementation in Java-
Example #1: UI Elements
In the example below we are implementing the Bridge pattern for a drawing UI Elements
Take a look at the class diagram.
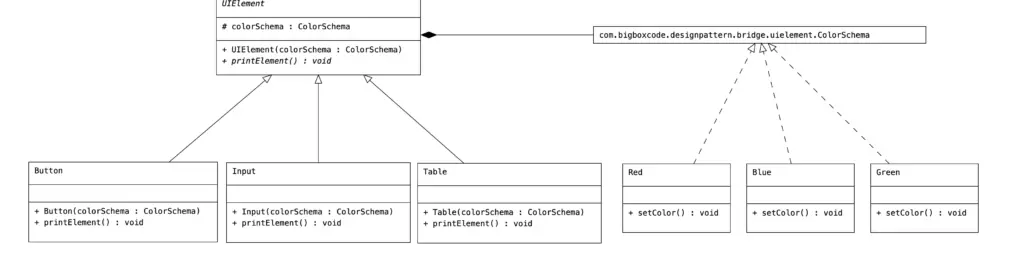
package com.bigboxcode.designpattern.bridge.uielement;
public interface ColorSchema {
void setColor();
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Red implements ColorSchema {
@Override
public void setColor() {
System.out.println("Setting proper color for Red color schema");
}
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Green implements ColorSchema {
@Override
public void setColor() {
System.out.println("Setting proper color for Green color schema");
}
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Blue implements ColorSchema {
@Override
public void setColor() {
System.out.println("Setting proper color for Blue color schema");
}
}
UI Element Abstract Class
package com.bigboxcode.designpattern.bridge.uielement;
public abstract class UIElement {
protected ColorSchema colorSchema;
public UIElement(ColorSchema colorSchema) {
this.colorSchema = colorSchema;
}
public abstract void printElement();
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Button extends UIElement{
public Button(ColorSchema colorSchema) {
super(colorSchema);
}
@Override
public void printElement() {
// Write code for printing element
System.out.println("Printing Button");
// Set color schema
colorSchema.setColor();
}
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Input extends UIElement{
public Input(ColorSchema colorSchema) {
super(colorSchema);
}
@Override
public void printElement() {
// Write code for printing element
System.out.println("Printing Input");
// Set color schema
colorSchema.setColor();
}
}
package com.bigboxcode.designpattern.bridge.uielement;
public class Table extends UIElement{
public Table(ColorSchema colorSchema) {
super(colorSchema);
}
@Override
public void printElement() {
// Write code for printing table element
System.out.println("Printing Table");
// Set color schema
colorSchema.setColor();
}
}
Demo
package com.bigboxcode.designpattern.bridge.uielement;
public class Demo {
public static void main(String args[]) {
// Print a Red table
UIElement table = new Table(new Red());
table.printElement();
// Separator for clear view of demo result
System.out.println("n------------------------------n");
// Print a Green Input box
UIElement input = new Input(new Green());
input.printElement();
// Separator for clear view of demo result
System.out.println("n------------------------------n");
// Print a Blue Button
UIElement button = new Button(new Blue());
button.printElement();
// Separator for clear view of demo result
System.out.println("n------------------------------n");
// Print a Red Button
UIElement button2 = new Button(new Red());
button2.printElement();
}
}
Output
The output of the demo above will be like below.
Printing Table
Setting proper color for Red color schema
------------------------------
Printing Input
Setting proper color for Green color schema
------------------------------
Printing Button
Setting proper color for Blue color schema
------------------------------
Printing Button
Setting proper color for Red color schema
Example #2: Transport Seat
In this example, we are going to demonstrate different types of seat selection in multiple types of transport.
Let’s take a look at the class diagram first.
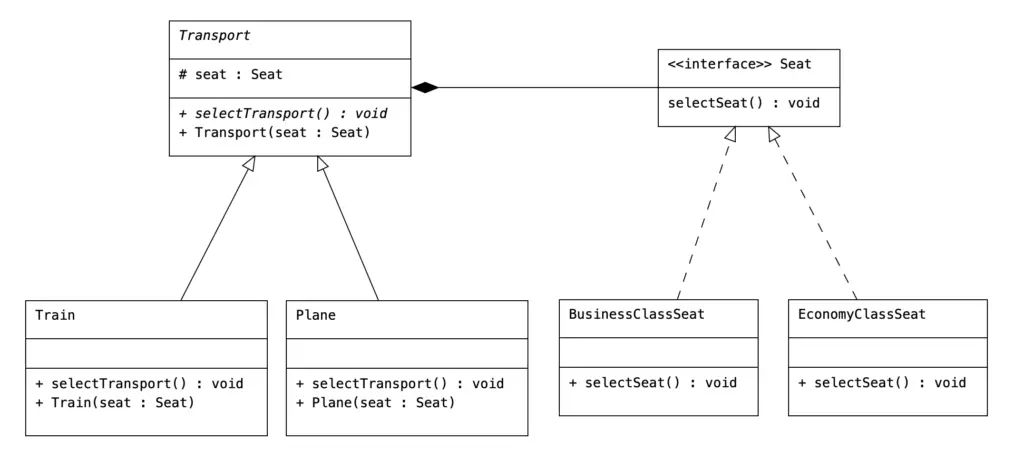
package com.bigboxcode.designpattern.bridge.transport;
public interface Seat {
void selectSeat();
}
package com.bigboxcode.designpattern.bridge.transport;
public class BusinessClassSeat implements Seat {
@Override
public void selectSeat() {
System.out.println("Select an Business class seat");
}
}
package com.bigboxcode.designpattern.bridge.transport;
public class EconomyClassSeat implements Seat{
@Override
public void selectSeat() {
System.out.println("Select an Economy class seat");
}
}
package com.bigboxcode.designpattern.bridge.transport;
public abstract class Transport {
protected Seat seat;
public Transport(Seat seat) {
this.seat = seat;
}
public abstract void selectTransport();
}
package com.bigboxcode.designpattern.bridge.transport;
public class Plane extends Transport {
public Plane(Seat seat) {
super(seat);
}
@Override
public void selectTransport() {
// Write code to select transport
System.out.println("Plane selected for transport");
// Select seat
seat.selectSeat();
}
}
package com.bigboxcode.designpattern.bridge.transport;
public class Train extends Transport {
public Train(Seat seat) {
super(seat);
}
@Override
public void selectTransport() {
// Write code to select transport
System.out.println("Train selected for transport");
// Select seat
seat.selectSeat();
}
}
Demo
package com.bigboxcode.designpattern.bridge.transport;
public class Demo {
public static void main(String args[]) {
Transport plane = new Plane(new BusinessClassSeat());
plane.selectTransport();
// Separator for clear view of demo result
System.out.println("n------------------------------n");
Transport plane2 = new Plane(new EconomyClassSeat());
plane2.selectTransport();
// Separator for clear view of demo result
System.out.println("n------------------------------n");
Transport train = new Train(new EconomyClassSeat());
train.selectTransport();
}
}
Output
Plane selected for transport
Select an Business class seat
------------------------------
Plane selected for transport
Select an Economy class seat
------------------------------
Train selected for transport
Select an Economy class seat
Source Code
Use the following link to get the source code:
Other Code Implementations
Use the following links to check Bridge pattern implementation in other programming languages.