Abstract Factory pattern is used to generate multiple factory generators.
NOTES
Abstract factory pattern works as a factory of the Factory pattern, so before learning the details of the Abstract factory pattern make sure to have a clear idea about the Factory pattern.
NOTES
In this article, we discuss the implementation of the Abstract Factory Pattern in Java.
See the Abstract Factory in other languages in the “Other Code Implementations” section. Or, use the link below to check the details of the Abstract Factory Pattern-
Examples
Here are some Abstract Factory Pattern examples in Java-
Example #1: Transport
Let’s take the example of a transport system. Take a look at the class diagram first.
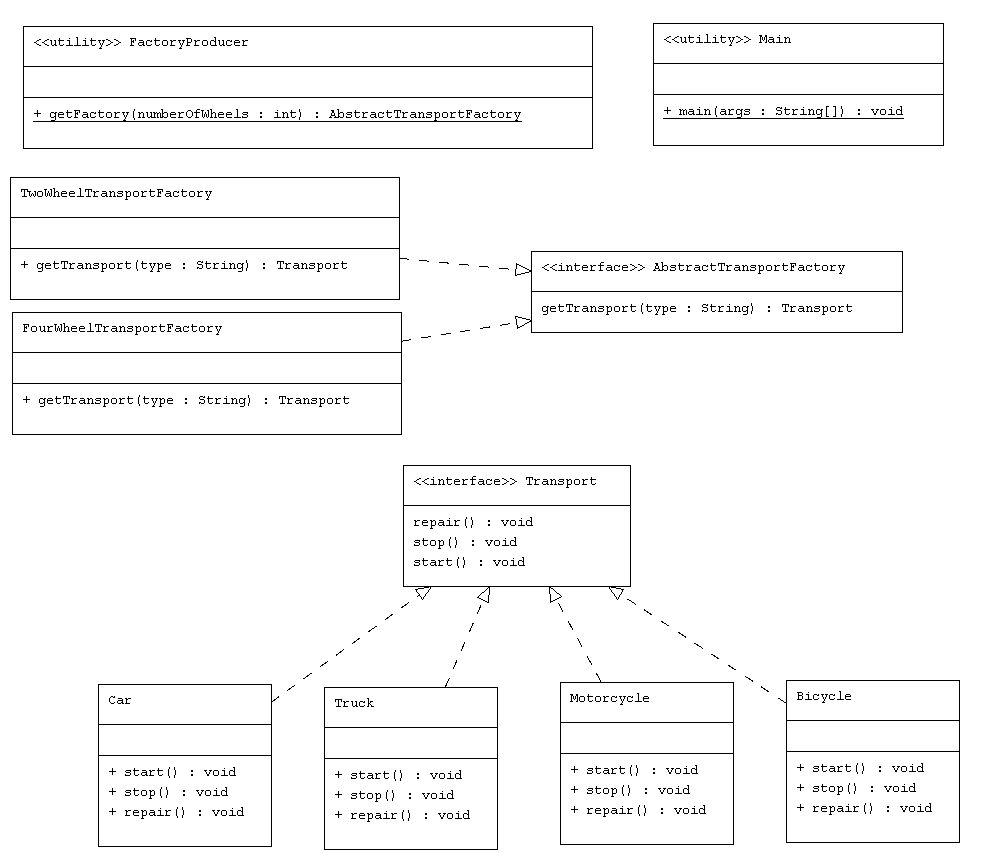
Transport Factory Interface
// Transport.java
package com.bigboxcode;
public interface Transport {
void start();
void stop();
void repair();
}
Bicycle Class
// Bicycle.java
package com.bigboxcode;
public class Bicycle implements Transport {
@Override
public void start() {
System.out.println("Bicycle Started");
}
@Override
public void stop() {
System.out.println("Bicycle Stopped");
}
@Override
public void repair() {
System.out.println("Bicycle Repair");
}
}
Motorcycle Class
// Motorcycle.java
package com.bigboxcode;
public class Motorcycle implements Transport {
@Override
public void start() {
System.out.println("Motorcycle Started");
}
@Override
public void stop() {
System.out.println("Motorcycle Stopped");
}
@Override
public void repair() {
System.out.println("Motorcycle Repair");
}
}
Car Class
// Car.java
package com.bigboxcode;
public class Car implements Transport {
@Override
public void start() {
System.out.println("Car Started");
}
@Override
public void stop() {
System.out.println("Car Stopped");
}
@Override
public void repair() {
System.out.println("Car Repair");
}
}
Truck Class
// Truck.java
package com.bigboxcode;
public class Truck implements Transport {
@Override
public void start() {
System.out.println("Truck Started");
}
@Override
public void stop() {
System.out.println("Truck Stopped");
}
@Override
public void repair() {
System.out.println("Truck Repair");
}
}
Abstract Transport Factory Interface
// AbstractTransportFactory.java
package com.bigboxcode;
public interface AbstractTransportFactory {
Transport getTransport(String type);
}
Two Wheel Transport Factory Class
// TwoWheelTransportFactory.java
package com.bigboxcode;
public class TwoWheelTransportFactory implements AbstractTransportFactory{
@Override
public Transport getTransport(String type) {
if (type.equalsIgnoreCase("bicycle")) {
return new Bicycle();
}
if (type.equalsIgnoreCase("motorcycle")) {
return new Motorcycle();
}
return null;
}
}
Four Wheel Transport Factory Class
// FourWheelTransportFactory.java
package com.bigboxcode;
public class FourWheelTransportFactory implements AbstractTransportFactory{
@Override
public Transport getTransport(String type) {
if (type.equalsIgnoreCase("car")) {
return new Car();
}
if (type.equalsIgnoreCase("truck")) {
return new Truck();
}
return null;
}
}
Factory Producer Class
// FactoryProducer.java
package com.bigboxcode;
public class FactoryProducer {
public static AbstractTransportFactory getFactory(int numberOfWheels) {
if (numberOfWheels == 2) {
return new TwoWheelTransportFactory();
}
if (numberOfWheels == 4) {
return new FourWheelTransportFactory();
}
return null;
}
}
Demo
// Main.java
package com.bigboxcode;
public class Main {
public static void main(String[] args) {
AbstractTransportFactory transportFactory1 = FactoryProducer.getFactory(2);
Transport transport1 = transportFactory1.getTransport("bicycle");
transport1.start();
AbstractTransportFactory transportFactory2 = FactoryProducer.getFactory(4);
Transport transport2 = transportFactory2.getTransport("truck");
transport2.start();
}
}
Output
Bicycle Started
Truck Started
Source Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: Transport | ![]() |
Other Code Implementations
Use the following links to check Abstract Factory pattern implementation in other programming languages.