Memento pattern is used to store an object’s history of state changes so that that history can be used to track back the change or be used for some other purpose.
This article demonstrates Memento pattern implementations in Java. Check the following examples.
Examples
Here are few examples of Memento pattern implementation in Java-
Example #1: General Memento
Let’s consider a general example of a simple Memento pattern demonstration.
Class Diagram
Take a look at the class diagram.
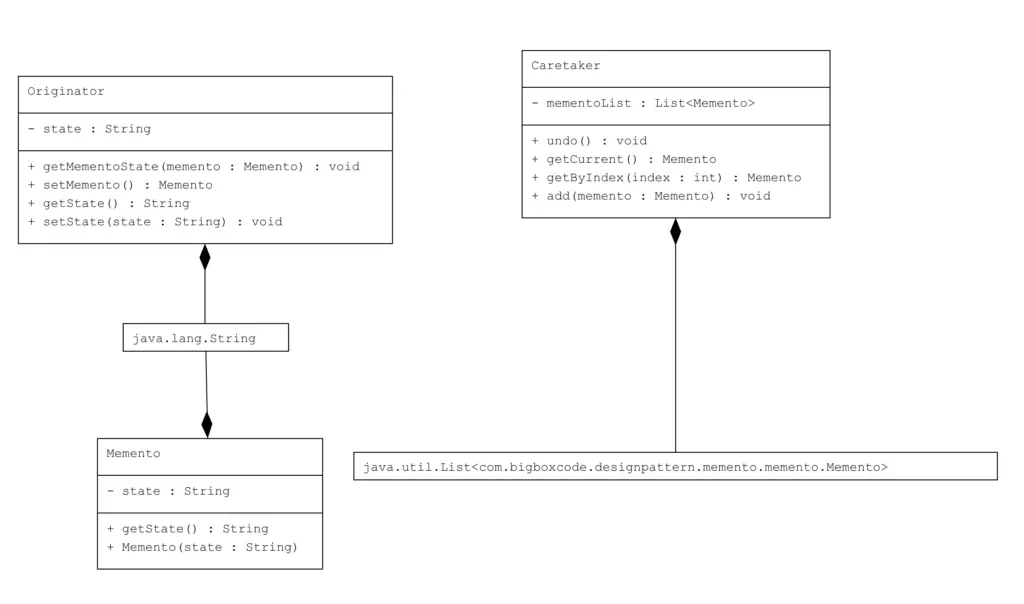
Memento
// Memento.java
package com.bigboxcode.designpattern.memento.memento;
public class Memento {
private String state;
public Memento(String state) {
this.state = state;
}
public String getState() {
return state;
}
}
Originator
// Originator.java
package com.bigboxcode.designpattern.memento.memento;
public class Originator {
private String state;
public void setState(String state) {
this.state = state;
}
public String getState() {
return state;
}
public Memento setMemento() {
System.out.println("Memento Saved with timestamp => " + state);
return new Memento(state);
}
public void getMementoState(Memento memento) {
state = memento.getState();
}
}
Caretaker
// Caretaker.java
package com.bigboxcode.designpattern.memento.memento;
import java.util.ArrayList;
import java.util.List;
public class Caretaker {
private List<Memento> mementoList = new ArrayList<>();
public void add(Memento memento) {
mementoList.add(memento);
}
public Memento getByIndex(int index) {
return mementoList.get(index);
}
public Memento getCurrent() {
return mementoList.get(mementoList.size() - 1);
}
public void undo() {
mementoList.remove(mementoList.size() - 1);
}
}
Demo
// Demo.java
package com.bigboxcode.designpattern.memento.memento;
public class Demo {
public static void main(String[] args) throws InterruptedException {
Caretaker caretaker = new Caretaker();
Originator originator = new Originator();
originator.setState("Time - 1 : " + System.currentTimeMillis());
caretaker.add(originator.setMemento());
// Add delay if required for testing
// Thread.sleep(1000);
originator.setState("Time - 2 : " + System.currentTimeMillis());
caretaker.add(originator.setMemento());
// Add delay if required for testing
// Thread.sleep(1000);
originator.setState("Time - 3 : " + System.currentTimeMillis());
caretaker.add(originator.setMemento());
System.out.println("---------------------------------------------");
System.out.println("Check state at index 1 (index starts at 0):");
Memento stateAtIndex1 = caretaker.getByIndex(1);
System.out.println(stateAtIndex1.getState());
System.out.println("---------------------------------------------");
System.out.println("Check last state:");
Memento lastState = caretaker.getCurrent();
System.out.println(lastState.getState());
System.out.println("---------------------------------------------");
System.out.println("Undoing last state");
caretaker.undo();
System.out.println("---------------------------------------------");
System.out.println("Check last state after undo:");
Memento lastStateAfterUndo = caretaker.getCurrent();
System.out.println(lastStateAfterUndo.getState());
}
}
Output
Memento Saved with timestamp => Time - 1 : 1677468449701
Memento Saved with timestamp => Time - 2 : 1677468450713
Memento Saved with timestamp => Time - 3 : 1677468451726
---------------------------------------------
Check state at index 1 (index starts at 0):
Time - 2 : 1677468450713
---------------------------------------------
Check last state:
Time - 3 : 1677468451726
---------------------------------------------
Undoing last state
---------------------------------------------
Check last state after undo:
Time - 2 : 1677468450713
Source Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: General Memento | ![]() |
Other Code Implementations
Use the following links to check the Memento pattern implementation in other programming languages.