Proxy pattern is used provide a placeholder or surrogate for an original object. Operation performed the proxy, calls operation(s) from the original object. This proxy object existing between the client and the original object to provide security and/or access control and/or additional functionality.
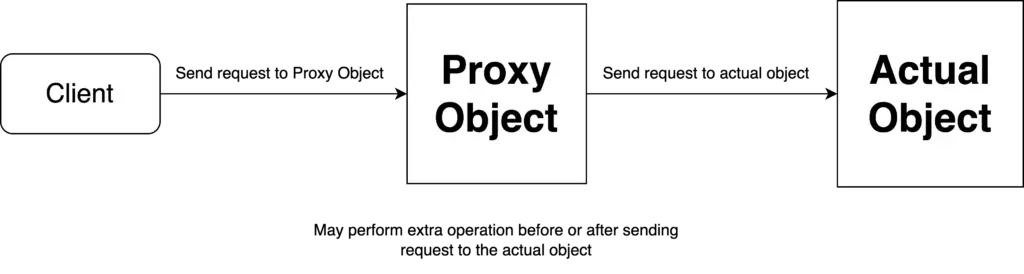
This article demonstrates Proxy pattern implementations in PHP. Check the implementation details and examples.
Implementation
Here are the steps for Proxy pattern implementation-
- Create an interface that will be used by the Original class and proxy class. This will make sure that both the class objects have same interface.
- Make sure the original/actual class implement the provided interface.
- Create proxy class and implement the interface.
- Define property in the proxy class of type of the original class. This property is used to store a reference to an original class object.
- In the method implement in the proxy class, call meths from the original class object (initialize the object if not initialize already). Add any additional functionality steps before or after the method calls.
Examples
Here are a few examples of the Proxy pattern implementation-
Example #1: Simple Proxy
Here is the simplest proxy implementation example.
Proxy Interface
- Create file “ProxyInterface.php”.
- Define interface “ProxyInterface”.
- Declare methods as per requirement.
This interface will be used by both the proxy and actual class.
<?php
// ProxyInterface.php
namespace BigBoxCodeDesignPatternProxySimpleProxy;
interface ProxyInterface {
function operation1(): void;
function operation2(): void;
}
Item Class
- Create file “Item.php”.
- Define class “Item”.
- Implement interface “ProxyInterface”. Implement methods for interface implementation. This class can be pre-existing, just make sure this class implements the “ProxyInteface”.
<?php
// Item.php
namespace BigBoxCodeDesignPatternProxySimpleProxy;
class Item implements ProxyInterface {
public function operation1(): void {
echo "Performing operation 1 in the Actual Objectn";
}
public function operation2(): void {
echo "Performing operation 2 in the Actual Objectn";
}
}
Proxy Class
- Create file “Proxy.php”.
- Define class “Proxy”.
- Implement interface “ProxyInterface” for the class.
- Define a private property named “$item” of type “ProxyInterface” (this can have null as an initial value).
- In the method implementations, call the methods from “$item”(actual object). If the “$item” is not set then create a new “Item” object and assign that to “$item”. In the method implementation there can be additional steps added before or after the method calls, like access checking and/or security measures and/or additional functions.
<?php
// Proxy.php
namespace BigBoxCodeDesignPatternProxySimpleProxy;
class Proxy implements ProxyInterface {
private ?ProxyInterface $item = null;
public function operation1(): void {
if ($this->item == null) {
$this->item = new Item();
}
$this->item->operation1();
}
public function operation2(): void {
if ($this->item == null) {
$this->item = new Item();
}
$this->item->operation2();
}
}
Demo
Usage is simple in this case. Create an proxy object and call methods from the proxy object.
<?php
// demo.php
require __DIR__ . '/../../vendor/autoload.php';
use BigBoxCodeDesignPatternProxySimpleProxyProxy;
$proxy = new Proxy();
$proxy->operation1();
$proxy->operation2();
Output
Output will be as below-
Performing operation 1 in the Actual Object
Performing operation 2 in the Actual Object
Source Code
Use the following link to get the source code:
Other Code Implementations
Use the following links to check Proxy pattern implementation in other programming languages.