Proxy pattern is used where we can not use the actual class object, and we want to represent the actual object with some proxy object.
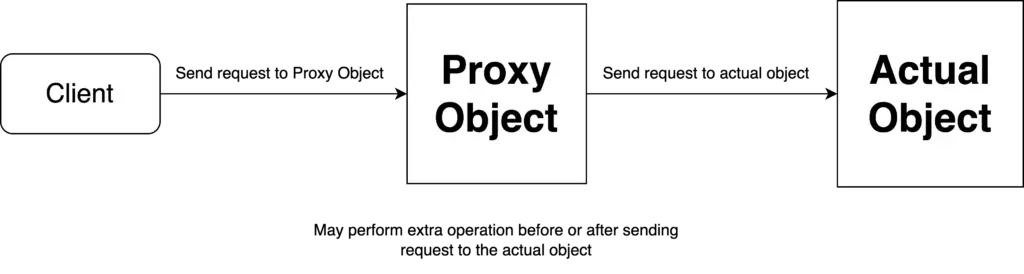
This article demonstrates Proxy pattern implementations in Java. Check the following examples.
Examples
Here are a few examples of the proxy pattern implementation in Java.
Example #1: Simple Proxy
Here is the simplest proxy implementation example.
Proxy Interface
Here is the proxy interface that ensures that the actual and proxy object matches.
// ProxyInterface.java
package com.bigboxcode.designpattern.proxy.simple;
public interface ProxyInterface {
void operation1();
void operation2();
}
Actual Class
Here is the actual class implementing the proxy interface.
// Actual.java
package com.bigboxcode.designpattern.proxy.simple;
public class Actual implements ProxyInterface {
@Override
public void operation1() {
System.out.println("Performing operation 1 in the Actual Object");
}
@Override
public void operation2() {
System.out.println("Performing operation 2 in the Actual Object");
}
}
Proxy Class
Here is the proxy class implementing proxy interface. Here are a few key points:
- The proxy class stores a reference to the actual class object.
- A new object will be created if the actual object is not already created. If the actual object reference already exists, then that will be used.
- Operations from the actual class object are called from the proxy class, to perform operations.
// Proxy.java
package com.bigboxcode.designpattern.proxy.simple;
public class Proxy implements ProxyInterface {
private ProxyInterface actual;
@Override
public void operation1() {
if (actual == null) {
actual = new Actual();
}
actual.operation1();
}
@Override
public void operation2() {
if (actual == null) {
actual = new Actual();
}
actual.operation2();
}
}
Demo
Using the proxy is simple. Just create an instance of the proxy class and use that instance to call any operation.
// Demo.java
package com.bigboxcode.designpattern.proxy.simple;
public class Demo {
public static void main(String[] args) {
ProxyInterface proxy = new Proxy();
proxy.operation1();
proxy.operation2();
}
}
Output
The output will be like the below.
Performing operation 1 in the Actual Object
Performing operation 2 in the Actual Object
Source Code
Use the following link to get the source code:
Other Code Implementations
Use the following links to check Proxy pattern implementation in other programming languages.