Proxy pattern is used to represent one object(as a proxy) with another object, where we can not use the original object. This is used to represent a remote object or to control access.
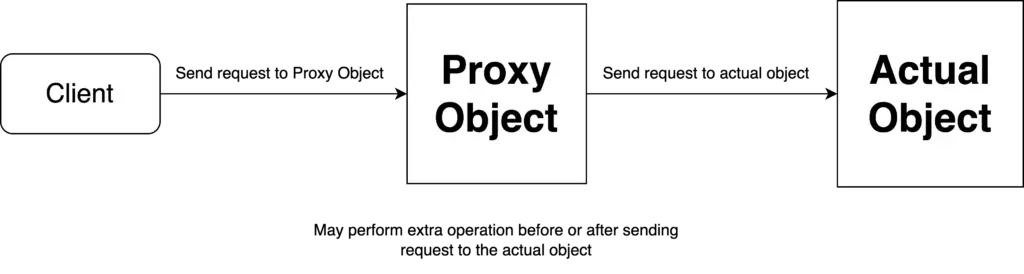
This article demonstrates Proxy pattern implementations in TypeScript. Check the implementation details and examples.
Implementation
Here are the steps for Proxy pattern implementation in TypeScript-
- Make sure there is an interface for the proxy implementation. Then the actual/original class and the proxy class can implement the same interface.
- The actual concrete class does not need any change. This class only needs to implement the interface mentioned above.
- Create a proxy class and implement the interface.
- Declare a property of the type of the original class. This will be used to hold a reference to the actual class object.
- In the method calls, if an object of original class is already created, then use that, else create new object.
- In the proxy method implementation, call the methods from the original object, when required.
Examples
Here are a few examples of the Proxy pattern implementation-
Example #1: Simple Proxy
Here is the simplest proxy implementation example.
Proxy Interface
- Create file “proxy-interface.ts”.
- Create interface “ProxyInterface”.
- Declare some methods as per requirement.
This interface will be used by both the actual class and proxy class, to ensure a common interface between those.
// proxy-interface.ts
interface ProxyInterface {
operation1(): void;
operation2(): void;
}
export default ProxyInterface;
Item Class
- Create file “item.ts”.
- Create class “Item”.
- Implement interface “ProxyInterface”. Implement methods for interface implementation.
// item.ts
import ProxyInterface from "./proxy-interface";
class Item implements ProxyInterface {
operation1(): void {
console.log("Performing operation 1 in the Actual Object");
}
operation2(): void {
console.log("Performing operation 2 in the Actual Object");
}
}
export default Item;
Proxy Class
- Create file “proxy.ts”.
- Create class “Proxy”.
- Implement interface “ProxyInterface”.
- Declare a private property named “item” of type “ProxyInterface”.
- Create an object of the actual class, and assign it to the “item” if that is not set already.
- In the method implementations, call the methods from “item”(actual object).
// proxy.ts
import Item from "./item";
import ProxyInterface from "./proxy-interface";
class Proxy implements ProxyInterface {
private item: ProxyInterface | null = null;
public operation1(): void {
if (this.item == null) {
this.item = new Item();
}
this.item.operation1();
}
public operation2(): void {
if (this.item == null) {
this.item = new Item();
}
this.item.operation2();
}
}
export default Proxy;
Demo
For using the proxy implementation, just create a new instance of the proxy and use that.
// demo.ts
import Proxy from "./proxy";
const proxy = new Proxy();
proxy.operation1();
proxy.operation2();
Output
Following output will be generated.
Performing operation 1 in the Actual Object
Performing operation 2 in the Actual Object
Source Code
Use the following link to get the source code:
Other Code Implementations
Use the following links to check Proxy pattern implementation in other programming languages.