Summary
Command Name | BLPOP |
Usage | Block until popping from HEAD of first non-empty list |
Group | ![]() |
ACL Category | @write @list @slow @blocking |
Time Complexity | O(N) |
Flag | WRITE BLOCKING |
Arity | -3 |
Notes
- In the time complexity, N is the number of provided keys.
Signature
BLPOP <key> [ <key> … ] <timeout>
Usage
BLPOP extracts/pops an item from the Left/HEAD of the first non-empty list. This is a blocking operation, so if no item is available in the provided list, this command will wait.
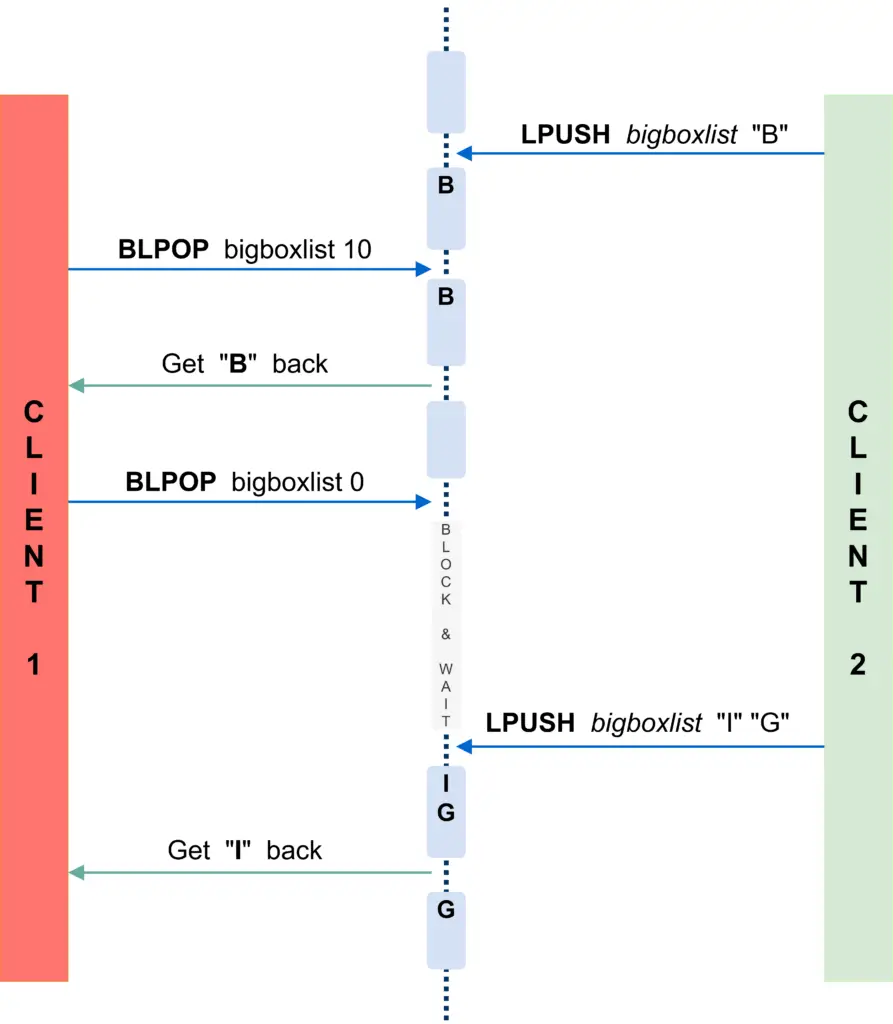
If there is an item available in any of the provided lists, then the command fetches the item, and command execution is complete.
If there is no item available in any of the provided lists, then the command will wait (based on the provided <timeout> time). When any of the lists get any item pushed from some other client, then the BLPOP command will extract the list item from Left/HEAD and complete command execution.
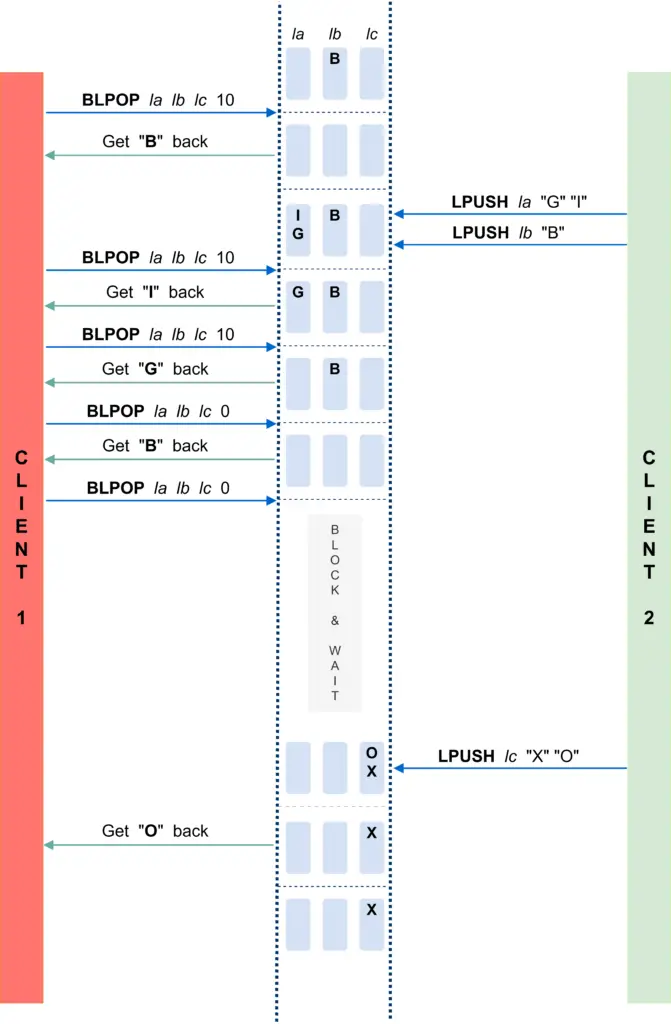
Arguments
Parameter | Description | Name | Type | Multiple |
---|---|---|---|---|
<key> | Key name(s) of the list | key | key | True |
<timeout> | Max time(in seconds) before stop waiting for data | timeout | double |
Notes
- <timeout> argument is the value in seconds. Indicates, how many seconds we want to wait at the maximum for popping data from list(s).
- If <timeout> is Zero(0) then the command will wait, until it can pop a value from the list. There will be no time limit.
Return Value
Return value | Case for the return value | Type |
---|---|---|
List name and item | Name of the list and item that is popped are returned togather | array |
(nil) | If list does not exist and/or the command is not able to pop data | null |
error | If the command is applied to data other than a list | error |
Notes
- Try to apply BLPOP to a list that does not exist- the command will behave like an empty list.
- If the key is not of type list, the following error message is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Examples
Here are a few examples of the BLPOP command usage-
# Redis BLPOP command examples
# Push item to list
127.0.0.1:6379> lpush bigboxlist B
(integer) 1
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "B"
# Apply BLPOP on the list with 10 second
127.0.0.1:6379> blpop bigboxlist 10
1) "bigboxlist"
2) "B"
# Apply BLPOP and wait for unlimited time, until data can be popped
127.0.0.1:6379> blpop bigboxlist 0
# Block and wait
# Executed the following LPUSH command in another terminal/client
# while the above BLPOP command is waiting
# 127.0.0.1:6379> lpush bigboxlist G I
# (integer) 2
# Result from above BLPOP command
1) "bigboxlist"
2) "I"
(15.25s)
# Apply BLPOP and wait 10 seconds
127.0.0.1:6379> blpop bigboxlist 10
1) "bigboxlist"
2) "G"
# Apply BLPOP and wait 10 seconds
# List is empty so no items are returned
127.0.0.1:6379> blpop bigboxlist 10
(nil)
(10.02s)
# Check if bigboxlist still exists, when all the items are popped
# The list does not exist anymore
127.0.0.1:6379> exists bigboxlist
(integer) 0
# Let's deal with multiple lists
# Here we are considering 3 lists - la, lb, lc
# Push data to list named lb
127.0.0.1:6379> lpush lb B
(integer) 1
# Apply BLPOP on la, lb, lc
# We get data from lb
127.0.0.1:6379> blpop la lb lc 10
1) "lb"
2) "B"
# Push G and I to la
127.0.0.1:6379> lpush la G I
(integer) 2
# Push B to lb
127.0.0.1:6379> lpush lb B
(integer) 1
# Apply BLPOP on la, lb, lc
# We get data from la
127.0.0.1:6379> blpop la lb lc 10
1) "la"
2) "I"
# Apply BLPOP on la, lb, lc
# We get data from la
127.0.0.1:6379> blpop la lb lc 10
1) "la"
2) "G"
# Apply BLPOP on la, lb, lc
# We get data from lb
127.0.0.1:6379> blpop la lb lc 0
1) "lb"
2) "B"
# Apply BLPOP with unlimited waiting time
# none of the la, lb, lc has any data
# so the command will block and wait
127.0.0.1:6379> blpop la lb lc 0
# block the and wait
# Apply following command in another terminal/client
# 127.0.0.1:6379> lpush lc X O
# (integer) 2
# Result from the above BLPOP
1) "lc"
2) "O"
(17.74s)
# Try to apply BLPOP to a non exiting list
# (nil) is returned
127.0.0.1:6379> blpop nonexistinglist 10
(nil)
(10.01s)
# Set a string value
127.0.0.1:6379> set bigboxstr "Some string in the big box"
OK
# Try to apply BLPOP on a string
# We get an error
127.0.0.1:6379> blpop bigboxstr 0
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Make sure to run the commands after running BLPOP, while the BLPOP is waiting. The commands are mentioned after the BLPOP command (if applicable in those cases).
Code Implementations
Here are the usage examples of the Redis BLPOP command in different programming languages.
// Redis BLPOP command example in Golang
package main
import (
"context"
"fmt"
"time"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
// Push item to list
// Command: lpush bigboxlist B
// Result: (integer) 1
pushResult, err := rdb.LPush(ctx, "bigboxlist", "B").Result()
if err != nil {
fmt.Println("Command: lpush bigboxlist B | Error: " + err.Error())
}
fmt.Println("Command: lpush bigboxlist B | Result: ", pushResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "B"
lrangeResult, err := rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Apply BLPOP on the list with 10 second
// Command: blpop bigboxlist 10
// Result:
// 1) "bigboxlist"
// 2) "B"
blpopResult, err := rdb.BLPop(ctx, 10 * time.Second, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: blpop bigboxlist 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop bigboxlist 10 | Result: ", blpopResult)
// Apply BLPOP and wait for unlimited time, until data can be popped
// Command: blpop bigboxlist 0
fmt.Println("Command: blpop bigboxlist 0")
fmt.Println("Waiting for result from BLPOP...")
// Block and wait
// Executed the following LPUSH command in another terminal/client
// while the above BLPOP command is waiting
// Command: lpush bigboxlist G I
// Result: (integer) 2
fmt.Println("Execute following command from separate terminal/client.nCommand: lpush bigboxlist G I")
// Result from above BLPOP command
// Result:
// 1) "bigboxlist"
// 2) "I"
// (15.25s)
blpopResult, err = rdb.BLPop(ctx, 0, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: blpop bigboxlist 0 | Error: " + err.Error())
}
fmt.Println("Command: blpop bigboxlist 0 | Result: ", blpopResult)
// Apply BLPOP and wait 10 seconds
// Command: blpop bigboxlist 10
// Result:
// 1) "bigboxlist"
// 2) "G"
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: blpop bigboxlist 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop bigboxlist 10 | Result: ", blpopResult)
// Apply BLPOP and wait 10 seconds
// List is empty so no items are returned
// Command: blpop bigboxlist 10
// Result:
// (nil)
// (10.02s)
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: blpop bigboxlist 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop bigboxlist 10 | Result: ", blpopResult)
// Check if bigboxlist still exists, when all the items are popped
// The list does not exist anymore
// Command: exists bigboxlist
// Result: (integer) 0
existsResult, err := rdb.Exists(ctx, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: exists bigboxlist | Error: " + err.Error())
}
fmt.Println("Command: exists bigboxlist | Result: ", existsResult)
// Let's deal with multiple lists
// Here we are considering 3 lists - la, lb, lc
fmt.Println("Let's deal with multiple listsnHere we are considering 3 lists - la, lb, lc")
// Push data to list named lb
// Command: lpush lb B
// Result: (integer) 1
pushResult, err = rdb.LPush(ctx, "lb", "B").Result()
if err != nil {
fmt.Println("Command: lpush lb B | Error: " + err.Error())
}
fmt.Println("Command: lpush lb B | Result: ", pushResult)
// Apply BLPOP on la, lb, lc
// We get data from lb
// Command: blpop la lb lc 10
// Result:
// 1) "lb"
// 2) "B"
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "la", "lb", "lc").Result()
if err != nil {
fmt.Println("Command: blpop la lb lc 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop la lb lc 10 | Result: ", blpopResult)
// Push G and I to la
// Command: lpush la G I
// Result: (integer) 2
pushResult, err = rdb.LPush(ctx, "la", "G", "I").Result()
if err != nil {
fmt.Println("Command: lpush la G I | Error: " + err.Error())
}
fmt.Println("Command: lpush la G I | Result: ", pushResult)
// Push B to lb
// Command: lpush lb B
// Result: (integer) 1
pushResult, err = rdb.LPush(ctx, "lb", "B").Result()
if err != nil {
fmt.Println("Command: lpush lb B | Error: " + err.Error())
}
fmt.Println("Command: lpush lb B | Result: ", pushResult)
// Apply BLPOP on la, lb, lc
// We get data from la
// Command: blpop la lb lc 10
// Result:
// 1) "la"
// 2) "I"
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "la", "lb", "lc").Result()
if err != nil {
fmt.Println("Command: blpop la lb lc 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop la lb lc 10 | Result: ", blpopResult)
// Apply BLPOP on la, lb, lc
// We get data from la
// Command: blpop la lb lc 10
// Result:
// 1) "la"
// 2) "G"
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "la", "lb", "lc").Result()
if err != nil {
fmt.Println("Command: blpop la lb lc 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop la lb lc 10 | Result: ", blpopResult)
// Apply BLPOP on la, lb, lc
// We get data from lb
// Command: blpop la lb lc 0
// Result:
// 1) "lb"
// 2) "B"
blpopResult, err = rdb.BLPop(ctx, 0, "la", "lb", "lc").Result()
if err != nil {
fmt.Println("Command: blpop la lb lc 0 | Error: " + err.Error())
}
fmt.Println("Command: blpop la lb lc 0 | Result: ", blpopResult)
// Apply BLPOP with unlimited waiting time
// none of the la, lb, lc has any data
// so the command will block and wait
// Command: blpop la lb lc 0
fmt.Println("Command: blpop la lb lc 0")
fmt.Println("Waiting for BLPOP to receive data...")
// block the and wait
// Apply following command in another terminal/client
// Command: lpush lc X O
// Result: (integer) 2
fmt.Println("Apply following command in separate terminal/client")
fmt.Println("Command: lpush lc X O")
// Result from the above BLPOP
// Result:
// 1) "lc"
// 2) "O"
// (17.74s)
blpopResult, err = rdb.BLPop(ctx, 0, "la", "lb", "lc").Result()
if err != nil {
fmt.Println("Command: blpop la lb lc 0 | Error: " + err.Error())
}
fmt.Println("Command: blpop la lb lc 0 | Result: ", blpopResult)
// Try to apply BLPOP to a non exiting list
// (nil) is returned
// Command: blpop nonexistinglist 10
// Result:
// (nil)
// (10.01s)
blpopResult, err = rdb.BLPop(ctx, 10 * time.Second, "nonexistinglist").Result()
if err != nil {
fmt.Println("Command: blpop nonexistinglist 10 | Error: " + err.Error())
}
fmt.Println("Command: blpop nonexistinglist 10 | Result: ", blpopResult)
// Set a string value
// Command: set bigboxstr "Some string in the big box"
// Result: OK
setResult, err := rdb.Set(ctx, "bigboxstr", "", 0).Result()
if err != nil {
fmt.Println("Command: set bigboxstr "Some string in the big box" | Error: " + err.Error())
}
fmt.Println("Command: set bigboxstr "Some string in the big box" | Result: " + setResult)
// Try to apply BLPOP on a string
// We get an error
// Command: blpop bigboxstr 0
// Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
blpopResult, err = rdb.BLPop(ctx, 0, "bigboxstr").Result()
if err != nil {
fmt.Println("Command: blpop bigboxstr 0 | Error: " + err.Error())
}
fmt.Println("Command: blpop bigboxstr 0 | Result: ", blpopResult)
}
Output:
Command: lpush bigboxlist B | Result: 1
Command: lrange bigboxlist 0 -1 | Result: [B]
Command: blpop bigboxlist 10 | Result: [bigboxlist B]
Command: blpop bigboxlist 0
Waiting for result from BLPOP...
Execute following command from separate terminal/client.
Command: lpush bigboxlist G I
Command: blpop bigboxlist 0 | Result: [bigboxlist I]
Command: blpop bigboxlist 10 | Result: [bigboxlist G]
Command: blpop bigboxlist 10 | Error: redis: nil
Command: blpop bigboxlist 10 | Result: []
Command: exists bigboxlist | Result: 0
Let's deal with multiple lists
Here we are considering 3 lists - la, lb, lc
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: [lb B]
Command: lpush la G I | Result: 2
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: [la I]
Command: blpop la lb lc 10 | Result: [la G]
Command: blpop la lb lc 0 | Result: [lb B]
Command: blpop la lb lc 0
Waiting for BLPOP to receive data...
Apply following command in separate terminal/client
Command: lpush lc X O
Command: blpop la lb lc 0 | Result: [lc O]
Command: blpop nonexistinglist 10 | Error: redis: nil
Command: blpop nonexistinglist 10 | Result: []
Command: set bigboxstr "Some string in the big box" | Result: OK
Command: blpop bigboxstr 0 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: blpop bigboxstr 0 | Result: []
Notes
- Use “BLPop” method from redis-go module.
- Signature of the method is-
BLPop(ctx context.Context, timeout time.Duration, keys …string) *StringSliceCmd
// Redis BLPOP command example in JavaScript(NodeJS)
import { createClient } from 'redis';
// Create redis client
const redisClient = createClient({
url: 'redis://default:@localhost:6379'
});
await redisClient.on('error', err => console.log('Error while connecting to Redis', err));
// Connect Redis client
await redisClient.connect();
/**
* Push item to list
*
* Command: lpush bigboxlist B
* Result: (integer) 1
*/
let commandResult = await redisClient.lPush("bigboxlist", "B");
console.log("Command: lpush bigboxlist B | Result: " + commandResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: " + commandResult);
/**
* Apply BLPOP on the list with 10 second
*
* Command: blpop bigboxlist 10
* Result:
* 1) "bigboxlist"
* 2) "B"
*/
commandResult = await redisClient.blPop("bigboxlist", 10);
console.log("Command: blpop bigboxlist 10 | Result: ", commandResult);
/**
* Apply BLPOP and wait for unlimited time, until data can be popped
*
* Command: blpop bigboxlist 0
*/
console.log("Command: blpop bigboxlist 0");
console.log("Waiting for result from BLPOP...");
// Block and wait
/**
* Executed the following LPUSH command in another terminal/client
* while the above BLPOP command is waiting
*
* Command: lpush bigboxlist G I
* Result: (integer) 2
*/
console.log("Execute following command from separate terminal/client.nCommand: lpush bigboxlist G I");
/**
* Result from above BLPOP command
* Result:
* 1) "bigboxlist"
* 2) "I"
* (15.25s)
*/
commandResult = await redisClient.blPop("bigboxlist", 0);
console.log("Command: blpop bigboxlist 0 | Result: ", commandResult);
/**
* Apply BLPOP and wait 10 seconds
*
* Command: blpop bigboxlist 10
* Result:
* 1) "bigboxlist"
* 2) "G"
*/
commandResult = await redisClient.blPop("bigboxlist", 10);
console.log("Command: blpop bigboxlist 10 | Result: ", commandResult);
/**
* Apply BLPOP and wait 10 seconds
* List is empty so no items are returned
*
* Command: blpop bigboxlist 10
* Result:
* (nil)
* (10.02s)
*/
commandResult = await redisClient.blPop("bigboxlist", 10);
console.log("Command: blpop bigboxlist 10 | Result: " + commandResult);
/**
* Check if bigboxlist still exists, when all the items are popped
* The list does not exist anymore
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
commandResult = await redisClient.exists("bigboxlist");
console.log("Command: exists bigboxlist | Result: " + commandResult);
/**
* Let's deal with multiple lists
* Here we are considering 3 lists - la, lb, lc
*/
console.log("Let's deal with multiple listsnHere we are considering 3 lists - la, lb, lc");
/**
* Push data to list named lb
*
* Command: lpush lb B
* Result: (integer) 1
*/
commandResult = await redisClient.lPush("lb", "B");
console.log("Command: lpush lb B | Result: " + commandResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from lb
*
* Command: blpop la lb lc 10
* Result:
* 1) "lb"
* 2) "B"
*/
commandResult = await redisClient.blPop(["la", "lb", "lc"], 10);
console.log("Command: blpop la lb lc 10 | Result: ", commandResult);
/**
* Push G and I to la
*
* Command: lpush la G I
* Result: (integer) 2
*/
commandResult = await redisClient.lPush("la", "G", "I");
console.log("Command: lpush la G I | Result: " + commandResult);
/**
* Push B to lb
*
* Command: lpush lb B
* Result: (integer) 1
*/
commandResult = await redisClient.lPush("lb", "B");
console.log("Command: lpush lb B | Result: " + commandResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from la
*
* Command: blpop la lb lc 10
* Result:
* 1) "la"
* 2) "I"
*/
commandResult = await redisClient.blPop(["la", "lb", "lc"], 10);
console.log("Command: blpop la lb lc 10 | Result: ", commandResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from la
*
* Command: blpop la lb lc 10
* Result:
* 1) "la"
* 2) "G"
*/
commandResult = await redisClient.blPop(["la", "lb", "lc"], 10);
console.log("Command: blpop la lb lc 10 | Result: ", commandResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from lb
*
* Command: blpop la lb lc 0
* Result:
* 1) "lb"
* 2) "B"
*/
commandResult = await redisClient.blPop(["la", "lb", "lc"], 10);
console.log("Command: blpop la lb lc 0 | Result: ", commandResult);
/**
* Apply BLPOP with unlimited waiting time
* none of the la, lb, lc has any data
* so the command will block and wait
*
* Command: blpop la lb lc 0
*/
console.log("Command: blpop la lb lc 0");
console.log("Waiting for BLPOP to receive data...");
// block the and wait
/**
* Apply following command in another terminal/client
*
* Command: lpush lc X O
* Result: (integer) 2
*/
console.log("Apply following command in separate terminal/client");
console.log("Command: lpush lc X O");
/**
* Result from the above BLPOP
* Result:
* 1) "lc"
* 2) "O"
* (17.74s)
*/
commandResult = await redisClient.blPop(["la", "lb", "lc"], 0);
console.log("Command: blpop la lb lc 0 | Result: ", commandResult);
/**
* Try to apply BLPOP to a non exiting list
* (nil) is returned
*
* Command: blpop nonexistinglist 10
* Result:
* (nil)
* (10.01s)
*/
commandResult = await redisClient.blPop("nonexistinglist", 10);
console.log("Command: blpop nonexistinglist 10 | Result: ", commandResult);
/**
* Set a string value
*
* Command: set bigboxstr "Some string in the big box"
* Result: OK
*/
commandResult = await redisClient.set("bigboxstr", "Some string in the big box");
console.log("Command: set bigboxstr "Some string in the big box" | Result: " + commandResult);
/**
* Try to apply BLPOP on a string
* We get an error
*
* Command: blpop bigboxstr 0
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.blPop("bigboxstr", 0);
console.log("Command: blpop bigboxstr 0 | Result: ", commandResult);
} catch (err) {
console.log("Command: blpop bigboxstr 0 | Error: ", err);
}
process.exit(0);
Output:
Command: lpush bigboxlist B | Result: 1
Command: lrange bigboxlist 0 -1 | Result: B
Command: blpop bigboxlist 10 | Result: { key: 'bigboxlist', element: 'B' }
Command: blpop bigboxlist 0
Waiting for result from BLPOP...
Execute following command from separate terminal/client.
Command: lpush bigboxlist G I
Command: blpop bigboxlist 0 | Result: { key: 'bigboxlist', element: 'I' }
Command: blpop bigboxlist 10 | Result: { key: 'bigboxlist', element: 'G' }
Command: blpop bigboxlist 10 | Result: null
Command: exists bigboxlist | Result: 0
Let's deal with multiple lists
Here we are considering 3 lists - la, lb, lc
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: { key: 'lb', element: 'B' }
Command: lpush la G I | Result: 1
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: { key: 'la', element: 'G' }
Command: blpop la lb lc 10 | Result: { key: 'lb', element: 'B' }
Command: blpop la lb lc 0 | Result: null
Command: blpop la lb lc 0
Waiting for BLPOP to receive data...
Apply following command in separate terminal/client
Command: lpush lc X O
Command: blpop la lb lc 0 | Result: { key: 'lc', element: 'O' }
Command: blpop nonexistinglist 10 | Result: null
Command: set bigboxstr "Some string in the big box" | Result: OK
Command: blpop bigboxstr 0 | Error: [ErrorReply: WRONGTYPE Operation against a key holding the wrong kind of value]
Notes
- Use the function “lTrim” from the package node-redis.
- Signature of the method is-
lTrim(key: RedisCommandArgument, start: number, stop: number)
// Redis BLPOP Command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class Blpop {
public static void main(String[] args) {
// Create connection pool
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Push item to list
*
* Command: lpush bigboxlist B
* Result: (integer) 1
*/
long pushResult = jedis.lpush("bigboxlist", "B");
System.out.println("Command: lpush bigboxlist B | Result: " + pushResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
*/
List<String> lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Apply BLPOP on the list with 10 second
*
* Command: blpop bigboxlist 10
* Result:
* 1) "bigboxlist"
* 2) "B"
*/
List<String> blpopResult = jedis.blpop(10, "bigboxlist");
System.out.println("Command: blpop bigboxlist 10 | Result: " + blpopResult.toString());
/**
* Apply BLPOP and wait for unlimited time, until data can be popped
*
* Command: blpop bigboxlist 0
*/
System.out.println("Command: blpop bigboxlist 0");
System.out.println("Waiting for result from BLPOP...");
// Block and wait
/**
* Executed the following LPUSH command in another terminal/client
* while the above BLPOP command is waiting
*
* Command: lpush bigboxlist G I
* Result: (integer) 2
*/
System.out.println("Execute following command from separate terminal/client.nCommand: lpush bigboxlist G I");
/**
* Result from above BLPOP command
* Result:
* 1) "bigboxlist"
* 2) "I"
* (15.25s)
*/
blpopResult = jedis.blpop(0, "bigboxlist");
System.out.println("Command: blpop bigboxlist 0 | Result: " + blpopResult.toString());
/**
* Apply BLPOP and wait 10 seconds
*
* Command: blpop bigboxlist 10
* Result:
* 1) "bigboxlist"
* 2) "G"
*/
blpopResult = jedis.blpop(10, "bigboxlist");
System.out.println("Command: blpop bigboxlist 10 | Result: " + blpopResult.toString());
/**
* Apply BLPOP and wait 10 seconds
* List is empty so no items are returned
*
* Command: blpop bigboxlist 10
* Result:
* (nil)
* (10.02s)
*/
blpopResult = jedis.blpop(10, "bigboxlist");
System.out.println("Command: blpop bigboxlist 10 | Result: " + blpopResult);
/**
* Check if bigboxlist still exists, when all the items are popped
* The list does not exist anymore
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
boolean existsResult = jedis.exists("bigboxlist");
System.out.println("Command: exists bigboxlist | Result: " + existsResult);
/**
* Let's deal with multiple lists
* Here we are considering 3 lists - la, lb, lc
*/
System.out.println("Let's deal with multiple listsnHere we are considering 3 lists - la, lb, lc");
/**
* Push data to list named lb
*
* Command: lpush lb B
* Result: (integer) 1
*/
pushResult = jedis.lpush("lb", "B");
System.out.println("Command: lpush lb B | Result: " + pushResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from lb
*
* Command: blpop la lb lc 10
* Result:
* 1) "lb"
* 2) "B"
*/
blpopResult = jedis.blpop(10, "la", "lb", "lc");
System.out.println("Command: blpop la lb lc 10 | Result: " + blpopResult.toString());
/**
* Push G and I to la
*
* Command: lpush la G I
* Result: (integer) 2
*/
pushResult = jedis.lpush("la", "G", "I");
System.out.println("Command: lpush la G I | Result: " + pushResult);
/**
* Push B to lb
*
* Command: lpush lb B
* Result: (integer) 1
*/
pushResult = jedis.lpush("lb", "B");
System.out.println("Command: lpush lb B | Result: " + pushResult);
/**
* Apply BLPOP on la, lb, lc
* We get data from la
*
* Command: blpop la lb lc 10
* Result:
* 1) "la"
* 2) "I"
*/
blpopResult = jedis.blpop(10, "la", "lb", "lc");
System.out.println("Command: blpop la lb lc 10 | Result: " + blpopResult.toString());
/**
* Apply BLPOP on la, lb, lc
* We get data from la
*
* Command: blpop la lb lc 10
* Result:
* 1) "la"
* 2) "G"
*/
blpopResult = jedis.blpop(10, "la", "lb", "lc");
System.out.println("Command: blpop la lb lc 10 | Result: " + blpopResult.toString());
/**
* Apply BLPOP on la, lb, lc
* We get data from lb
*
* Command: blpop la lb lc 0
* Result:
* 1) "lb"
* 2) "B"
*/
blpopResult = jedis.blpop(0, "la", "lb", "lc");
System.out.println("Command: blpop la lb lc 0 | Result: " + blpopResult.toString());
/**
* Apply BLPOP with unlimited waiting time
* none of the la, lb, lc has any data
* so the command will block and wait
*
* Command: blpop la lb lc 0
*/
System.out.println("Command: blpop la lb lc 0");
System.out.println("Waiting for BLPOP to receive data...");
// block the and wait
/**
* Apply following command in another terminal/client
*
* Command: lpush lc X O
* Result: (integer) 2
*/
System.out.println("Apply following command in separate terminal/client");
System.out.println("Command: lpush lc X O");
/**
* Result from the above BLPOP
* Result:
* 1) "lc"
* 2) "O"
* (17.74s)
*/
blpopResult = jedis.blpop(0, "la", "lb", "lc");
System.out.println("Command: blpop la lb lc 0 | Result: " + blpopResult.toString());
/**
* Try to apply BLPOP to a non exiting list
* (nil) is returned
*
* Command: blpop nonexistinglist 10
* Result:
* (nil)
* (10.01s)
*/
blpopResult = jedis.blpop(10, "nonexistinglist");
System.out.println("Command: blpop nonexistinglist 10 | Result: " + blpopResult);
/**
* Set a string value
*
* Command: set bigboxstr "Some string in the big box"
* Result: OK
*/
String setResult = jedis.set("bigboxstr", "");
System.out.println("Command: set bigboxstr "Some string in the big box" | Result: " + setResult);
/**
* Try to apply BLPOP on a string
* We get an error
*
* Command: blpop bigboxstr 0
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
blpopResult = jedis.blpop(0, "bigboxstr");
System.out.println("Command: blpop bigboxstr 0 | Result: " + blpopResult.toString());
} catch (Exception e) {
System.out.println("Command: blpop bigboxstr 0 | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: lpush bigboxlist B | Result: 1
Command: lrange bigboxlist 0 -1 | Result: [B]
Command: blpop bigboxlist 10 | Result: [bigboxlist, B]
Command: blpop bigboxlist 0
Waiting for result from BLPOP...
Execute following command from separate terminal/client.
Command: lpush bigboxlist G I
Command: blpop bigboxlist 0 | Result: [bigboxlist, I]
Command: blpop bigboxlist 10 | Result: [bigboxlist, G]
Command: blpop bigboxlist 10 | Result: null
Command: exists bigboxlist | Result: false
Let's deal with multiple lists
Here we are considering 3 lists - la, lb, lc
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: [lb, B]
Command: lpush la G I | Result: 2
Command: lpush lb B | Result: 1
Command: blpop la lb lc 10 | Result: [la, I]
Command: blpop la lb lc 10 | Result: [la, G]
Command: blpop la lb lc 0 | Result: [lb, B]
Command: blpop la lb lc 0
Waiting for BLPOP to receive data...
Apply following command in separate terminal/client
Command: lpush lc X O
Command: blpop la lb lc 0 | Result: [lc, O]
Command: blpop nonexistinglist 10 | Result: null
Command: set bigboxstr "Some string in the big box" | Result: OK
Command: blpop bigboxstr 0 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “blpop” from Jedis package.
- Signatures of the method are-
public List<String> blpop(final int timeout, final String key)
public KeyValue<String, String> blpop(double timeout, String key)
KeyValue<String, String> blpop(double timeout, String key)
public KeyValue<String, String> blpop(double timeout, String… keys)
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: B, I, G, B, O, X, C, O, D, E, B, I, O
Command: ltrim bigboxlist 3 -1
Command: lrange bigboxlist 0 -1 | Result: B, O, X, C, O, D, E, B, I, O
Command: ltrim bigboxlist 0 6
Command: lrange bigboxlist 0 -1 | Result: B, O, X, C, O, D, E
Command: ltrim bigboxlist 3 100
Command: lrange bigboxlist 0 -1 | Result: C, O, D, E
Command: ltrim bigboxlist 2 1
Command: lrange bigboxlist 0 -1 | Result:
Command: ltrim nonexistinglist 0 1
Command: set bigboxstr "Some string for test" | Result: True
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ListTrim” from StackExchange.Redis.
- Signature of the method is-
void ListTrim(RedisKey key, long start, long stop, CommandFlags flags = CommandFlags.None)
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => I
[2] => G
[3] => B
[4] => O
[5] => X
[6] => C
[7] => O
[8] => D
[9] => E
[10] => B
[11] => I
[12] => O
)
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => O
[2] => X
[3] => C
[4] => O
[5] => D
[6] => E
[7] => B
[8] => I
[9] => O
)
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => O
[2] => X
[3] => C
[4] => O
[5] => D
[6] => E
)
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => C
[1] => O
[2] => D
[3] => E
)
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
)
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ltrim” of predis.
- Signature of the method is-
ltrim(string $key, int $start, int $stop): mixed
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ['B', 'I', 'G', 'B', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: ltrim bigboxlist 3 -1 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['B', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: ltrim bigboxlist 0 6 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['B', 'O', 'X', 'C', 'O', 'D', 'E']
Command: ltrim bigboxlist 3 100 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['C', 'O', 'D', 'E']
Command: ltrim bigboxlist 2 1 | Result: True
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: True
Command: set bigboxstr "Some string for test" | Result: True
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “ltrim” from redis-py.
- Signature of the method is –
def ltrim(self, name: str, start: int, end: int) -> Union[Awaitable[str], str]
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["B", "O", "X", "C", "O", "D", "E"]
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["C", "O", "D", "E"]
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “ltrim” from the redis-rb.
- Signature of the method is-
# @param [String] key
# @param [Integer] start start index
# @param [Integer] stop stop index
# @return [String] `OK`
def ltrim(key, start, stop)
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LPUSH | ![]() |
LRANGE | ![]() |