Summary
Command Name | RPUSH |
Usage | Append item(s) to list |
Group | ![]() |
ACL Category | @write @list @fast |
Time Complexity | O(1) |
Flag | WRITE DENYOM FAST |
Arity | -3 |
NOTES
- For a single element time complexity of this command is O(1).
- If we push N number of elements at the same time using RPUSH command then the complexity will be O(N).
Signature
RPUSH <key> <string_element> [ <string_element> ...]
Usage
Append one or more elements to the list. The element is added to the tail/right of the list, that’s why this command is named RPUSH(R+PUSH).
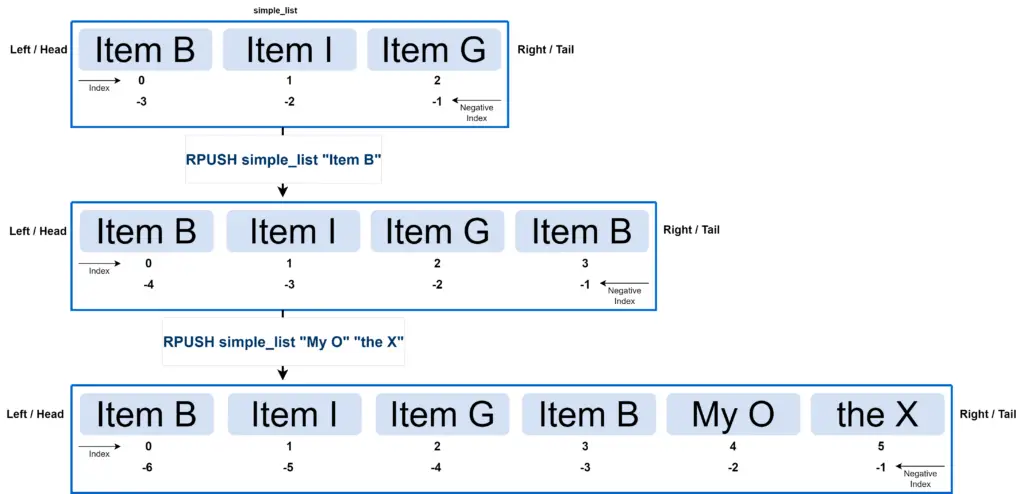
Items are inserted to the right one by one. If multiple elements are provided to the command, then the first item is pushed to the right, then the next ones.
NOTES
- If the list does not exist, then this command creates the list and then pushes the item(s).
Arguments
Parameter | Description | Name | Type |
---|---|---|---|
<key> | Name of the key | key | key |
<string_element> | Element to add to the list | element | string |
Return Value
Return value | Case for the return value | Type |
---|---|---|
Length of list | If the item is added successfully, the length of the list is returned | integer |
error | If the element is not a list. | error |
NOTES
- If the type of the value in the provided key is not a string then the following error is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Examples
Here are a few examples of the RPUSH command usage-
# Redis RPUSH command examples
# Push item to bigboxlist
# list does not exist yet,
# so first list is created then item pushed into it
127.0.0.1:6379> rpush bigboxlist "first item"
(integer) 1
# Push item to list
127.0.0.1:6379> rpush bigboxlist "second item"
(integer) 2
# Check list items
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "first item"
2) "second item"
# Push item to user card for user id 16
# The key we are using here is user:16:cart
127.0.0.1:6379> rpush user:16:cart 986
(integer) 1
# Push another item
127.0.0.1:6379> rpush user:16:cart 32
(integer) 2
# Push another item to list
127.0.0.1:6379> rpush user:16:cart 102
(integer) 3
# Check list item
127.0.0.1:6379> lrange user:16:cart 0 -1
1) "986"
2) "32"
3) "102"
# Push multiple items to list
127.0.0.1:6379> rpush user:16:cart 1049 167 348 2055
(integer) 7
# Check list items
127.0.0.1:6379> lrange user:16:cart 0 -1
1) "986"
2) "32"
3) "102"
4) "1049"
5) "167"
6) "348"
7) "2055"
# Create a new string type key
127.0.0.1:6379> set bigboxstr "test string here"
OK
# Try to use RPUSH command on a string
# We get an error as the type does not match
127.0.0.1:6379> rpush bigboxstr "changed string here"
(error) WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- When we push multiple elements in the same RPUSH command, the items are pushed from left to right. So the leftmost item is pushed first.
Code Implementations
Redis RPUSH command example implementation in Golang, NodeJS, Java, C#, PHP, Python, Ruby-
// Redis RPUSH command example in Golang
package main
import (
"context"
"fmt"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
/**
* Push item to bigboxlist
* list does not exist yet,
* so first list is created then item pushed into it
*
* Command: rpush bigboxlist "first item"
* Result: (integer) 1
*/
pushResult, err := rdb.RPush(ctx, "bigboxlist", "first item").Result()
if err != nil {
fmt.Println("Command: rpush bigboxlist "first item" | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxlist "first item" | Result: ", pushResult)
/**
* Push item to list
*
* Command: rpush bigboxlist "second item"
* Result: (integer) 2
*/
pushResult, err = rdb.RPush(ctx, "bigboxlist", "second item").Result()
if err != nil {
fmt.Println("Command: rpush bigboxlist "second item" | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxlist "second item" | Result: ", pushResult)
/**
* Check list items
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "first item"
* 2) "second item"
*/
listItems, err := rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", listItems)
/**
* Push item to user card for user id 16
* The key we are using here is user:16:cart
*
* Command: rpush user:16:cart 986
* Result: (integer) 1
*/
pushResult, err = rdb.RPush(ctx, "user:16:cart", "986").Result()
if err != nil {
fmt.Println("Command: rpush user:16:cart 986 | Error: " + err.Error())
}
fmt.Println("Command: rpush user:16:cart 986 | Result: ", pushResult)
/**
* Push another item
*
* Command: rpush user:16:cart 32
* Result: (integer) 2
*/
pushResult, err = rdb.RPush(ctx, "user:16:cart", "32").Result()
if err != nil {
fmt.Println("Command: rpush user:16:cart 32 | Result: " + err.Error())
}
fmt.Println("Command: rpush user:16:cart 32 | Result: ", pushResult)
/**
* Push another item to list
*
* Command: rpush user:16:cart 102
* Result: (integer) 3
*/
pushResult, err = rdb.RPush(ctx, "user:16:cart", "102").Result()
if err != nil {
fmt.Println("Command: rpush user:16:cart 102 | Error: " + err.Error())
}
fmt.Println("Command: rpush user:16:cart 102 | Result: ", pushResult)
/**
* Check list item
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
*/
listItems, err = rdb.LRange(ctx, "user:16:cart", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange user:16:cart 0 -1 | Error:" + err.Error())
}
fmt.Println("Command: lrange user:16:cart 0 -1 | Result: ", listItems)
/**
* Push multiple items to list
*
* Command: rpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
pushResult, err = rdb.RPush(ctx, "user:16:cart", "1049", "167", "348", "2055").Result()
if err != nil {
fmt.Println("Command: rpush user:16:cart 1049 167 348 2055 | Error: " + err.Error())
}
fmt.Println("Command: rpush user:16:cart 1049 167 348 2055 | Result: ", pushResult)
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
* 4) "1049"
* 5) "167"
* 6) "348"
* 7) "2055"
*/
listItems, err = rdb.LRange(ctx, "user:16:cart", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange user:16:cart 0 -1 | Error:" + err.Error())
}
fmt.Println("Command: lrange user:16:cart 0 -1 | Result:", listItems)
/**
* Create a new string type key
*
* Command: set bigboxstr "test string here"
* Result: OK
*/
setResult, err := rdb.Set(ctx, "bigboxstr", "test string here", 0).Result()
if err != nil {
fmt.Println("Command: set bigboxstr "test string here" | Error: " + err.Error())
}
fmt.Println("Command: set bigboxstr "test string here" | Result: " + setResult)
/**
* Try to use RPUSH command on a string
* We get an error as the type does not match
*
* Command: rpush bigboxstr "changed string here"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
pushResult, err = rdb.RPush(ctx, "bigboxstr", "changed string here").Result()
if err != nil {
fmt.Println("Command: rpush bigboxstr "changed string here" | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxstr "changed string here" | Result: ", pushResult)
}
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [first item second item]
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result: [986 32 102]
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: [986 32 102 1049 167 348 2055]
Command: set bigboxstr "test string here" | Result: OK
Command: rpush bigboxstr "changed string here" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: rpush bigboxstr "changed string here" | Result: 0
NOTES
- Use “
RPush
” method from redis-go module. - Signature of the method is-
RPush(ctx context.Context, key string, values ...interface{}) *IntCmd
// Redis RPUSH command example in JavaScript(NodeJS)
import { createClient } from "redis";
// Create redis client
const redisClient = createClient({
url: "redis://default:@localhost:6379",
});
redisClient.on("error", (err) =>
console.log("Error while connecting to Redis", err)
);
// Connect Redis client
await redisClient.connect();
/**
* Push item to bigboxlist
* list does not exist yet,
* so first list is created then item pushed into it
*
* Command: rpush bigboxlist "first item"
* Result: (integer) 1
*/
let commandResult = await redisClient.rPush("bigboxlist", "first item");
console.log(
'Command: rpush bigboxlist "first item" | Result: ' + commandResult
);
/**
* Push item to list
*
* Command: rpush bigboxlist "second item"
* Result: (integer) 2
*/
commandResult = await redisClient.rPush("bigboxlist", "second item");
console.log(
'Command: rpush bigboxlist "second item" | Result: ' + commandResult
);
/**
* Check list items
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "first item"
* 2) "second item"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Push item to user card for user id 16
* The key we are using here is user:16:cart
*
* Command: rpush user:16:cart 986
* Result: (integer) 1
*/
commandResult = await redisClient.rPush("user:16:cart", "986");
console.log("Command: rpush user:16:cart 986 | Result: " + commandResult);
/**
* Push another item
*
* Command: rpush user:16:cart 32
* Result: (integer) 2
*/
commandResult = await redisClient.rPush("user:16:cart", "32");
console.log("Command: rpush user:16:cart 32 | Result: " + commandResult);
/**
* Push another item to list
*
* Command: rpush user:16:cart 102
* Result: (integer) 3
*/
commandResult = await redisClient.rPush("user:16:cart", "102");
console.log("Command: rpush user:16:cart 102 | Result: " + commandResult);
/**
* Check list item
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
*/
commandResult = await redisClient.lRange("user:16:cart", 0, -1);
console.log("Command: lrange user:16:cart 0 -1 | Result:", commandResult);
/**
* Push multiple items to list
*
* Command: rpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
commandResult = await redisClient.rPush("user:16:cart", [
"1049",
"167",
"348",
"2055",
]);
console.log(
"Command: rpush user:16:cart 1049 167 348 2055 | Result: " + commandResult
);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
* 4) "1049"
* 5) "167"
* 6) "348"
* 7) "2055"
*/
commandResult = await redisClient.lRange("user:16:cart", 0, -1);
console.log("Command: lrange user:16:cart 0 -1 | Result: ", commandResult);
/**
* Create a new string type key
*
* Command: set bigboxstr "test string here"
* Result: OK
*/
commandResult = await redisClient.set("bigboxstr", "test string here");
console.log(
'Command: set bigboxstr "test string here" | Result: ' + commandResult
);
/**
* Try to use RPUSH command on a string
* We get an error as the type does not match
*
* Command: rpush bigboxstr "changed string here"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.rPush("bigboxstr", "changed string here");
console.log(
'Command: rpush bigboxstr "changed string here" | Result: ' + commandResult
);
} catch (e) {
console.log('Command: rpush bigboxstr "changed string here" | Error: ' + e);
}
process.exit(0);
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [ 'first item', 'second item' ]
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result: [ '986', '32', '102' ]
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: [
'986', '32',
'102', '1049',
'167', '348',
'2055'
]
Command: set bigboxstr "test string here" | Result: OK
Command: rpush bigboxstr "changed string here" | Error: Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use the function “
rPush
” of node-redis. - Signature of the method-
function rPush(key: RedisCommandArgument, element: RedisCommandArgument | Array<RedisCommandArgument>)
// Redis RPUSH Command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class RPush {
public static void main(String[] args) {
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Push item to bigboxlist
* list does not exist yet,
* so first list is created then item pushed into it
*
* Command: rpush bigboxlist "first item"
* Result: (integer) 1
*/
long pushResult = jedis.rpush("bigboxlist", "first item");
System.out.println("Command: rpush bigboxlist "first item" | Result: " + pushResult);
/**
* Push item to list
*
* Command: rpush bigboxlist "second item"
* Result: (integer) 2
*/
pushResult = jedis.rpush("bigboxlist", "second item");
System.out.println("Command: rpush bigboxlist "second item" | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "first item"
* 2) "second item"
*/
List<String> listItems = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + listItems.toString());
/**
* Push item to user card for user id 16
* The key we are using here is user:16:cart
*
* Command: rpush user:16:cart 986
* Result: (integer) 1
*/
pushResult = jedis.rpush("user:16:cart", "986");
System.out.println("Command: rpush user:16:cart 986 | Result: " + pushResult);
/**
* Push another item
*
* Command: rpush user:16:cart 32
* Result: (integer) 2
*/
pushResult = jedis.rpush("user:16:cart", "32");
System.out.println("Command: rpush user:16:cart 32 | Result: " + pushResult);
/**
* Push another item to list
*
* Command: rpush user:16:cart 102
* Result: (integer) 3
*/
pushResult = jedis.rpush("user:16:cart", "102");
System.out.println("Command: rpush user:16:cart 102 | Result: " + pushResult);
/**
* Check list item
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
*/
listItems = jedis.lrange("user:16:cart", 0, -1);
System.out.println("Command: lrange user:16:cart 0 -1 | Result:" + listItems.toString());
/**
* Push multiple items to list
*
* Command: rpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
pushResult = jedis.rpush("user:16:cart", "1049", "167", "348", "2055");
System.out.println("Command: rpush user:16:cart 1049 167 348 2055 | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
* 4) "1049"
* 5) "167"
* 6) "348"
* 7) "2055"
*/
listItems = jedis.lrange("user:16:cart", 0, -1);
System.out.println("Command: lrange user:16:cart 0 -1 | Result:" + listItems.toString());
/**
* Create a new string type key
*
* Command: set bigboxstr "test string here"
* Result: OK
*/
String setResult = jedis.set("bigboxstr", "test string here");
System.out.println("Command: set bigboxstr "test string here" | Result: " + setResult);
/**
* Try to use RPUSH command on a string
* We get an error as the type does not match
*
* Command: rpush bigboxstr "changed string here"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
pushResult = jedis.rpush("bigboxstr", "changed string here");
System.out.println("Command: rpush bigboxstr "changed string here" | Result: " + pushResult);
} catch (Exception e) {
System.out.println("Command: rpush bigboxstr "changed string here" | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [first item, second item]
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:[986, 32, 102]
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:[986, 32, 102, 1049, 167, 348, 2055]
Command: set bigboxstr "test string here" | Result: OK
Command: rpush bigboxstr "changed string here" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use method “
rpush
” from Jedis package. - The signatures of the method are-
public long rpush(final String key, final String... strings)
// Redis RPUSH command examples in C#
using StackExchange.Redis;
namespace RPush
{
internal class Program
{
static void Main(string[] args)
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase rdb = redis.GetDatabase();
/**
* Push item to bigboxlist
* list does not exist yet,
* so first list is created then item pushed into it
*
* Command: rpush bigboxlist "first item"
* Result: (integer) 1
*/
long pushResult = rdb.ListRightPush("bigboxlist", "first item");
Console.WriteLine("Command: rpush bigboxlist "first item" | Result: " + pushResult);
/**
* Push item to list
*
* Command: rpush bigboxlist "second item"
* Result: (integer) 2
*/
pushResult = rdb.ListRightPush("bigboxlist", "second item");
Console.WriteLine("Command: rpush bigboxlist "second item" | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "first item"
* 2) "second item"
*/
RedisValue[] listItems = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + String.Join(", ", listItems));
/**
* Push item to user card for user id 16
* The key we are using here is user:16:cart
*
* Command: rpush user:16:cart 986
* Result: (integer) 1
*/
pushResult = rdb.ListRightPush("user:16:cart", "986");
Console.WriteLine("Command: rpush user:16:cart 986 | Result: " + pushResult);
/**
* Push another item
*
* Command: rpush user:16:cart 32
* Result: (integer) 2
*/
pushResult = rdb.ListRightPush("user:16:cart", "32");
Console.WriteLine("Command: rpush user:16:cart 32 | Result: " + pushResult);
/**
* Push another item to list
*
* Command: rpush user:16:cart 102
* Result: (integer) 3
*/
pushResult = rdb.ListRightPush("user:16:cart", "102");
Console.WriteLine("Command: rpush user:16:cart 102 | Result: " + pushResult);
/**
* Check list item
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
*/
listItems = rdb.ListRange("user:16:cart", 0, -1);
Console.WriteLine("Command: lrange user:16:cart 0 -1 | Result:" + String.Join(", ", listItems));
/**
* Push multiple items to list
*
* Command: rpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
pushResult = rdb.ListRightPush("user:16:cart", new RedisValue[] { "1049", "167", "348", "2055" });
Console.WriteLine("Command: rpush user:16:cart 1049 167 348 2055 | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
* 4) "1049"
* 5) "167"
* 6) "348"
* 7) "2055"
*/
listItems = rdb.ListRange("user:16:cart", 0, -1);
Console.WriteLine("Command: lrange user:16:cart 0 -1 | Result:" + String.Join(", ", listItems));
/**
* Create a new string type key
*
* Command: set bigboxstr "test string here"
* Result: OK
*/
bool setResult = rdb.StringSet("bigboxstr", "test string here");
Console.WriteLine("Command: set bigboxstr "test string here" | Result: " + setResult);
/**
* Try to use RPUSH command on a string
* We get an error as the type does not match
*
* Command: rpush bigboxstr "changed string here"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try
{
pushResult = rdb.ListRightPush("bigboxstr", "another site");
Console.WriteLine("Command: rpush bigboxstr "another site" | Result: " + pushResult);
}
catch (Exception e)
{
Console.WriteLine("Command: rpush bigboxstr "another site" | Error: " + e.Message);
}
}
}
}
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: first item, second item
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:986, 32, 102
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:986, 32, 102, 1049, 167, 348, 2055
Command: set bigboxstr "test string here" | Result: True
Command: rpush bigboxstr "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use the method “
” from StackExchange.Redis.ListRightPush
- Method signatures-
long ListRightPush(RedisKey key, RedisValue value, When when = When.Always, CommandFlags flags = CommandFlags.None)
long ListRightPush(RedisKey key, RedisValue[] values, When when = When.Always, CommandFlags flags = CommandFlags.None)
long ListRightPush(RedisKey key, RedisValue[] values, CommandFlags flags)
<?php
// Redis RPUSH command example in PHP
require 'vendor/autoload.php';
// Connect to Redis
$redisClient = new PredisClient([
'scheme' => 'tcp',
'host' => 'localhost',
'port' => 6379,
]);
/**
* Push item to bigboxlist
* list does not exist yet,
* so first list is created then item pushed into it
*
* Command: rpush bigboxlist "first item"
* Result: (integer) 1
*/
$commandResult = $redisClient->rpush("bigboxlist", ["first item"]);
echo "Command: rpush bigboxlist "first item" | Result: " . $commandResult . "n";
/**
* Push item to list
*
* Command: rpush bigboxlist "second item"
* Result: (integer) 2
*/
$commandResult = $redisClient->rpush("bigboxlist", ["second item"]);
echo "Command: rpush bigboxlist "second item" | Result: " . $commandResult . "n";
/**
* Check list items
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "first item"
* 2) "second item"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result:n";
print_r($commandResult);
/**
* Push item to user card for user id 16
* The key we are using here is user:16:cart
*
* Command: rpush user:16:cart 986
* Result: (integer) 1
*/
$commandResult = $redisClient->rpush("user:16:cart", ["986"]);
echo "Command: rpush user:16:cart 986 | Result: " . $commandResult . "n";
/**
* Push another item
*
* Command: rpush user:16:cart 32
* Result: (integer) 2
*/
$commandResult = $redisClient->rpush("user:16:cart", ["32"]);
echo "Command: rpush user:16:cart 32 | Result: " . $commandResult . "n";
/**
* Push another item to list
*
* Command: rpush user:16:cart 102
* Result: (integer) 3
*/
$commandResult = $redisClient->rpush("user:16:cart", ["102"]);
echo "Command: rpush user:16:cart 102 | Result: " . $commandResult . "n";
/**
* Check list item
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
*/
$commandResult = $redisClient->lrange("user:16:cart", 0, -1);
echo "Command: lrange user:16:cart 0 -1 | Result:n";
print_r($commandResult);
/**
* Push multiple items to list
*
* Command: rpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
$commandResult = $redisClient->rpush("user:16:cart", ["1049", "167", "348", "2055"]);
echo "Command: rpush user:16:cart 1049 167 348 2055 | Result: " . $commandResult . "n";
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "986"
* 2) "32"
* 3) "102"
* 4) "1049"
* 5) "167"
* 6) "348"
* 7) "2055"
*/
$commandResult = $redisClient->lrange("user:16:cart", 0, -1);
echo "Command: lrange user:16:cart 0 -1 | Result:n";
print_r($commandResult);
/**
* Create a new string type key
*
* Command: set bigboxstr "test string here"
* Result: OK
*/
$commandResult = $redisClient->set("bigboxstr", "test string here");
echo "Command: set bigboxstr "test string here" | Result: " . $commandResult . "n";
/**
* Try to use RPUSH command on a string
* We get an error as the type does not match
*
* Command: rpush bigboxstr "changed string here"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
$commandResult = $redisClient->rpush("bigboxstr", ["changed string here"]);
echo "Command: rpush bigboxstr "changed string here" | Result: " . $commandResult . "n";
} catch (Exception $e) {
echo "Command: rpush bigboxstr "changed string here" | Error: " . $e->getMessage() . "n";
}
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result:
Array
(
[0] => first item
[1] => second item
)
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
Array
(
[0] => 986
[1] => 32
[2] => 102
)
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:
Array
(
[0] => 986
[1] => 32
[2] => 102
[3] => 1049
[4] => 167
[5] => 348
[6] => 2055
)
Command: set bigboxstr "test string here" | Result: OK
Command: rpush bigboxstr "changed string here" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use the method “
rpush
” of predis. - Signature of the method is-
rpush(string $key, array $values): int
# Redis RPUSH command example in Python
import redis
import time
# Create Redis client
redisClient = redis.Redis(host='localhost', port=6379,
username='default', password='',
decode_responses=True)
# Push item to bigboxlist
# list does not exist yet,
# so first list is created then item pushed into it
# Command: rpush bigboxlist "first item"
# Result: (integer) 1
commandResult = redisClient.rpush("bigboxlist", "first item")
print("Command: rpush bigboxlist "first item" | Result: {}".format(commandResult))
# Push item to list
# Command: rpush bigboxlist "second item"
# Result: (integer) 2
commandResult = redisClient.rpush("bigboxlist", "second item")
print("Command: rpush bigboxlist "second item" | Result: {}".format(commandResult))
# Check list items
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "first item"
# 2) "second item"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result:{}".format(commandResult))
# Push item to user card for user id 16
# The key we are using here is user:16:cart
# Command: rpush user:16:cart 986
# Result: (integer) 1
commandResult = redisClient.rpush("user:16:cart", "986")
print("Command: rpush user:16:cart 986 | Result: {}".format(commandResult))
# Push another item
# Command: rpush user:16:cart 32
# Result: (integer) 2
commandResult = redisClient.rpush("user:16:cart", "32")
print("Command: rpush user:16:cart 32 | Result: {}".format(commandResult))
# Push another item to list
# Command: rpush user:16:cart 102
# Result: (integer) 3
commandResult = redisClient.rpush("user:16:cart", "102")
print("Command: rpush user:16:cart 102 | Result: {}".format(commandResult))
# Check list item
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "986"
# 2) "32"
# 3) "102"
commandResult = redisClient.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result:{}".format(commandResult))
# Push multiple items to list
# Command: rpush user:16:cart 1049 167 348 2055
# Result: (integer) 7
commandResult = redisClient.rpush("user:16:cart", "1049", "167", "348", "2055")
print("Command: rpush user:16:cart 1049 167 348 2055 | Result: {}".format(commandResult))
# Check list items
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "986"
# 2) "32"
# 3) "102"
# 4) "1049"
# 5) "167"
# 6) "348"
# 7) "2055"
commandResult = redisClient.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result: {}".format(commandResult))
# Create a new string type key
# Command: set bigboxstr "test string here"
# Result: OK
commandResult = redisClient.set("bigboxstr", "my site")
print("Command: set bigboxstr "my site" | Result: {}".format(commandResult))
# Try to use RPUSH command on a string
# We get an error as the type does not match
# Command: rpush bigboxstr "changed string here"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
try:
commandResult = redisClient.rpush("bigboxstr", "another site")
print("Command: rpush bigboxstr "another site" | Result: {}".format(commandResult))
except Exception as error:
print("Command: rpush bigboxstr "another site" | Error: ", error)
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result:['first item', 'second item']
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:['986', '32', '102']
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: ['986', '32', '102', '1049', '167', '348', '2055']
Command: set bigboxstr "my site" | Result: True
Command: rpush bigboxstr "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use method “
rpush
” from redis-py. - Signature of the method is –
def rpush(self, name: str, *values: FieldT) -> Union[Awaitable[int], int]
# Redis RPUSH command example in Ruby
require 'redis'
redis = Redis.new(host: "localhost", port: 6379)
# Push item to bigboxlist
# list does not exist yet,
# so first list is created then item pushed into it
# Command: rpush bigboxlist "first item"
# Result: (integer) 1
commandResult = redis.rpush("bigboxlist", "first item")
print("Command: rpush bigboxlist "first item" | Result: ", commandResult, "n")
# Push item to list
# Command: rpush bigboxlist "second item"
# Result: (integer) 2
commandResult = redis.rpush("bigboxlist", "second item")
print("Command: rpush bigboxlist "second item" | Result: ", commandResult, "n")
# Check list items
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "first item"
# 2) "second item"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result:", commandResult, "n")
# Push item to user card for user id 16
# The key we are using here is user:16:cart
# Command: rpush user:16:cart 986
# Result: (integer) 1
commandResult = redis.rpush("user:16:cart", "986")
print("Command: rpush user:16:cart 986 | Result: ", commandResult, "n")
# Push another item
# Command: rpush user:16:cart 32
# Result: (integer) 2
commandResult = redis.rpush("user:16:cart", "32")
print("Command: rpush user:16:cart 32 | Result: ", commandResult, "n")
# Push another item to list
# Command: rpush user:16:cart 102
# Result: (integer) 3
commandResult = redis.rpush("user:16:cart", "102")
print("Command: rpush user:16:cart 102 | Result: ", commandResult, "n")
# Check list item
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "986"
# 2) "32"
# 3) "102"
commandResult = redis.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result:", commandResult, "n")
# Push multiple items to list
# Command: rpush user:16:cart 1049 167 348 2055
# Result: (integer) 7
commandResult = redis.rpush("user:16:cart", ["1049", "167", "348", "2055"])
print("Command: rpush user:16:cart 1049 167 348 2055 | Result: ", commandResult, "n")
# Check list items
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "986"
# 2) "32"
# 3) "102"
# 4) "1049"
# 5) "167"
# 6) "348"
# 7) "2055"
commandResult = redis.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result: ", commandResult, "n")
# Create a new string type key
# Command: set bigboxstr "test string here"
# Result: OK
commandResult = redis.set("bigboxstr", "test string here")
print("Command: set bigboxstr "test string here" | Result: ", commandResult, "n")
# Try to use RPUSH command on a string
# We get an error as the type does not match
# Command: rpush bigboxstr "changed string here"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
begin
commandResult = redis.rpush("bigboxstr", "changed string here")
print("Command: rpush bigboxstr "changed string here" | Result: ", commandResult, "n")
rescue => e
print("Command: rpush bigboxstr "changed string here" | Error: ", e, "n")
end
Output:
Command: rpush bigboxlist "first item" | Result: 1
Command: rpush bigboxlist "second item" | Result: 2
Command: lrange bigboxlist 0 -1 | Result:["first item", "second item"]
Command: rpush user:16:cart 986 | Result: 1
Command: rpush user:16:cart 32 | Result: 2
Command: rpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:["986", "32", "102"]
Command: rpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: ["986", "32", "102", "1049", "167", "348", "2055"]
Command: set bigboxstr "test string here" | Result: OK
Command: rpush bigboxstr "changed string here" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
NOTES
- Use method “
rpush
” from the redis-rb. - Signature of the method is-
# @param [String] key
# @param [String, Array<String>] value string value, or array of string values to push
# @return [Integer] the length of the list after the push operation
def rpush(key, value)
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LPUSH | ![]() |
LRANGE | ![]() |