Summary
Command Name | LREM |
Usage | Remove item(s) from list |
Group | ![]() |
ACL Category | @write @list @slow |
Time Complexity | O(N+M) |
Flag | WRITE |
Arity | 4 |
Notes
- In the time complexity, N is the length of the list, and M is the removed items.
Signature
LSET <key> <count> <item>
Usage
Remove items from a list. We can provide a <count> argument, in that case, the command will remove <count> number of occurrences of the item.
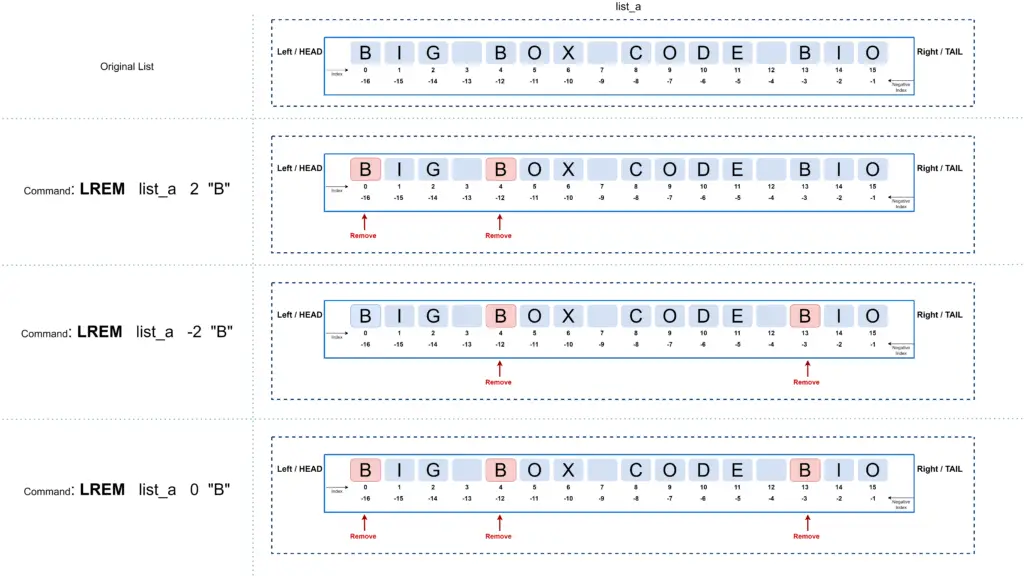
Notes
- If all the items of the list are removed by this command, then the list is deleted.
Arguments
Parameter | Description | Name | Type |
---|---|---|---|
<key> | Name of the key of the list | key | key |
<count> | Number of occurrences to remove | count | integer |
<item> | Item to remove | element | string |
Notes
- Here is what the value of argument <count> behaves like-
- count = 0 : Remove all occurrences of the item
- count > 0 : Remove <count> number of occurrences from the list, starting from the Left/HEAD and then moving to the Right/TAIL
- count < 0 : Remove <count> number of occurrences, starting from the Right/TAIL and then moving backward to the Left/HEAD
Return Value
Return value | Case for the return value | Type |
---|---|---|
Number of removed items | On successful execution of the command | integer |
error | If command applied on data that is not a list | error |
Notes
- If the type of the value in the provided key is not a string then the following error is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value - If the key does not exist then the command treats it like an empty list, and will return Zero(0).
Examples
Here are a few examples of the LREM command usage-
# Redis LREM command examples
# Create list and push items
127.0.0.1:6379> rpush bigboxlist B I G B O X C O D E B I O
(integer) 13
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "B"
2) "I"
3) "G"
4) "B"
5) "O"
6) "X"
7) "C"
8) "O"
9) "D"
10) "E"
11) "B"
12) "I"
13) "O"
# Remove 2 occurrences of "B" starting from the Left/HEAD
127.0.0.1:6379> lrem bigboxlist 2 "B"
(integer) 2
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "I"
2) "G"
3) "O"
4) "X"
5) "C"
6) "O"
7) "D"
8) "E"
9) "B"
10) "I"
11) "O"
# Remove 2 occurrences of "O" starting from the Right/TAIL
127.0.0.1:6379> lrem bigboxlist -2 "O"
(integer) 2
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "I"
2) "G"
3) "O"
4) "X"
5) "C"
6) "D"
7) "E"
8) "B"
9) "I"
# Remove all occurrences of "I"
127.0.0.1:6379> lrem bigboxlist 0 "I"
(integer) 2
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "G"
2) "O"
3) "X"
4) "C"
5) "D"
6) "E"
7) "B"
# Try to remove 1000 occurrences of "B" starting from the HEAD
# Only 1 occurrence removed as there was only 1 "B" in the list
127.0.0.1:6379> lrem bigboxlist 1000 "B"
(integer) 1
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "G"
2) "O"
3) "X"
4) "C"
5) "D"
6) "E"
# Try to delete a non existing item
127.0.0.1:6379> lrem bigboxlist 0 "non existing item"
(integer) 0
# Try to delete from a non existing list
# It is treated as an empty list and returns zero(0)
127.0.0.1:6379> lrem nonexistinglist 0 A
(integer) 0
# Set some string value
127.0.0.1:6379> set bigboxstr "Some str value"
OK
# Try to use LREM on a string
# We get an error
127.0.0.1:6379> lrem bigboxstr 0 "S"
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- <count> is the maximum number of occurrences that LREM command will remove. If the real occurrences are less than the <count> argument, then all are removed.
Code Implementations
Here are the usage examples of the Redis LREM command in different programming languages.
// Redis LREM command example in Golang
package main
import (
"context"
"fmt"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
// Create list and push items
// Command: rpush bigboxlist B I G B O X C O D E B I O
// Result: (integer) 13
rpushResult, err := rdb.RPush(ctx, "bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O").Result()
if err != nil {
fmt.Println("Command: rpush bigboxlist B I G B O X C O D E B I O | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: ", rpushResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "B"
// 2) "I"
// 3) "G"
// 4) "B"
// 5) "O"
// 6) "X"
// 7) "C"
// 8) "O"
// 9) "D"
// 10) "E"
// 11) "B"
// 12) "I"
// 13) "O"
lrangeResult, err := rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Remove 2 occurrences of "B" starting from the Left/HEAD
// Command: lrem bigboxlist 2 "B"
// Result: (integer) 2
lremResult, err := rdb.LRem(ctx, "bigboxlist", 2, "B").Result()
if err != nil {
fmt.Println("Command: lrem bigboxlist 2 "B" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxlist 2 "B" | Result: ", lremResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "I"
// 2) "G"
// 3) "O"
// 4) "X"
// 5) "C"
// 6) "O"
// 7) "D"
// 8) "E"
// 9) "B"
// 10) "I"
// 11) "O"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Remove 2 occurrences of "O" starting from the Right/TAIL
// Command: lrem bigboxlist -2 "O"
// Result: (integer) 2
lremResult, err = rdb.LRem(ctx, "bigboxlist", -2, "O").Result()
if err != nil {
fmt.Println("Command: lrem bigboxlist -2 "O" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxlist -2 "O" | Result: ", lremResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "I"
// 2) "G"
// 3) "O"
// 4) "X"
// 5) "C"
// 6) "D"
// 7) "E"
// 8) "B"
// 9) "I"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Remove all occurrences of "I"
// Command: lrem bigboxlist 0 "I"
// Result: (integer) 2
lremResult, err = rdb.LRem(ctx, "bigboxlist", 0, "I").Result()
if err != nil {
fmt.Println("Command: lrem bigboxlist 0 "I" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxlist 0 "I" | Result: ", lremResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "G"
// 2) "O"
// 3) "X"
// 4) "C"
// 5) "D"
// 6) "E"
// 7) "B"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Try to remove 1000 occurrences of "B" starting from the HEAD
// Only 1 occurrence removed as there was only 1 "B" in the list
// Command: lrem bigboxlist 1000 "B"
// Result: (integer) 1
lremResult, err = rdb.LRem(ctx, "bigboxlist", 1000, "B").Result()
if err != nil {
fmt.Println("Command: lrem bigboxlist 1000 "B" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxlist 1000 "B" | Result: ", lremResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "G"
// 2) "O"
// 3) "X"
// 4) "C"
// 5) "D"
// 6) "E"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Try to delete a non existing item
// Command: lrem bigboxlist 0 "non existing item"
// Result: (integer) 0
lremResult, err = rdb.LRem(ctx, "bigboxlist", 0, "non existing item").Result()
if err != nil {
fmt.Println("Command: lrem bigboxlist 0 "non existing item" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxlist 0 "non existing item" | Result: ", lremResult)
// Try to delete from a non existing list
// It is treated as an empty list and returns zero(0)
// Command: lrem nonexistinglist 0 A
// Result: (integer) 0
lremResult, err = rdb.LRem(ctx, "nonexistinglist", 0, "A").Result()
if err != nil {
fmt.Println("Command: lrem nonexistinglist 0 A | Error: " + err.Error())
}
fmt.Println("Command: lrem nonexistinglist 0 A | Result: ", lremResult)
// Set some string value
// Command: set bigboxstr "Some str value"
// Result: OK
setResult, err := rdb.Set(ctx, "bigboxstr", "Some str value", 0).Result()
if err != nil {
fmt.Println("Command: set bigboxstr "Some str value" | Error: " + err.Error())
}
fmt.Println("Command: set bigboxstr "Some str value" | Result: " + setResult)
// Try to use LREM on a string
// We get an error
// Command: lrem bigboxstr 0 "S"
// Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
lremResult, err = rdb.LRem(ctx, "bigboxstr", 0, "S").Result()
if err != nil {
fmt.Println("Command: lrem bigboxstr 0 "S" | Error: " + err.Error())
}
fmt.Println("Command: lrem bigboxstr 0 "S" | Result: ", lremResult)
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [B I G B O X C O D E B I O]
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [I G O X C O D E B I O]
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [I G O X C D E B I]
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [G O X C D E B]
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: [G O X C D E]
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: OK
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: lrem bigboxstr 0 "S" | Result: 0
Notes
- Use “LRem” method from redis-go module.
- Signature of the method is-
func (c cmdable) LRem(ctx context.Context, key string, count int64, value interface{}) *IntCmd
// Redis LREM command example in JavaScript(NodeJS)
import { createClient } from "redis";
// Create redis client
const redisClient = createClient({
url: "redis://default:@localhost:6379",
});
await redisClient.on("error", (err) =>
console.log("Error while connecting to Redis", err)
);
// Connect Redis client
await redisClient.connect();
/**
* Create list and push items
*
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
let commandResult = await redisClient.rPush("bigboxlist", [
"B",
"I",
"G",
"B",
"O",
"X",
"C",
"O",
"D",
"E",
"B",
"I",
"O",
]);
console.log(
"Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " +
commandResult
);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
* 2) "I"
* 3) "G"
* 4) "B"
* 5) "O"
* 6) "X"
* 7) "C"
* 8) "O"
* 9) "D"
* 10) "E"
* 11) "B"
* 12) "I"
* 13) "O"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Remove 2 occurrences of "B" starting from the Left/HEAD
*
* Command: lrem bigboxlist 2 "B"
* Result: (integer) 2
*/
commandResult = await redisClient.lRem("bigboxlist", 2, "B");
console.log('Command: lrem bigboxlist 2 "B" | Result: ' + commandResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "O"
* 7) "D"
* 8) "E"
* 9) "B"
* 10) "I"
* 11) "O"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Remove 2 occurrences of "O" starting from the Right/TAIL
*
* Command: lrem bigboxlist -2 "O"
* Result: (integer) 2
*/
commandResult = await redisClient.lRem("bigboxlist", -2, "O");
console.log('Command: lrem bigboxlist -2 "O" | Result: ' + commandResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "D"
* 7) "E"
* 8) "B"
* 9) "I"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Remove all occurrences of "I"
*
* Command: lrem bigboxlist 0 "I"
* Result: (integer) 2
*/
commandResult = await redisClient.lRem("bigboxlist", 0, "I");
console.log('Command: lrem bigboxlist 0 "I" | Result: ' + commandResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
* 7) "B"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Try to remove 1000 occurrences of "B" starting from the HEAD
* Only 1 occurrence removed as there was only 1 "B" in the list
*
* Command: lrem bigboxlist 1000 "B"
* Result: (integer) 1
*/
commandResult = await redisClient.lRem("bigboxlist", 1000, "B");
console.log('Command: lrem bigboxlist 1000 "B" | Result: ' + commandResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Try to delete a non existing item
*
* Command: lrem bigboxlist 0 "non existing item"
* Result: (integer) 0
*/
commandResult = await redisClient.lRem("bigboxlist", 0, "non existing item");
console.log(
'Command: lrem bigboxlist 0 "non existing item" | Result: ' + commandResult
);
/**
* Try to delete from a non existing list
* It is treated as an empty list and returns zero(0)
*
* Command: lrem nonexistinglist 0 A
* Result: (integer) 0
*/
commandResult = await redisClient.lRem("nonexistinglist", 0, "A");
console.log("Command: lrem nonexistinglist 0 A | Result: " + commandResult);
/**
* Set some string value
*
* Command: set bigboxstr "Some str value"
* Result: OK
*/
commandResult = await redisClient.set("bigboxstr", "Some str value");
console.log(
'Command: set bigboxstr "Some str value" | Result: ' + commandResult
);
/**
* Try to use LREM on a string
* We get an error
*
* Command: lrem bigboxstr 0 "S"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.lRem("bigboxstr", 0, "S");
console.log('Command: lrem bigboxstr 0 "S" | Result: ' + commandResult);
} catch (err) {
console.log('Command: lrem bigboxstr 0 "S" | Error: ', err);
}
process.exit(0);
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [
'B', 'I', 'G', 'B',
'O', 'X', 'C', 'O',
'D', 'E', 'B', 'I',
'O'
]
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [
'I', 'G', 'O', 'X',
'C', 'O', 'D', 'E',
'B', 'I', 'O'
]
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [
'I', 'G', 'O',
'X', 'C', 'D',
'E', 'B', 'I'
]
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [
'G', 'O', 'X',
'C', 'D', 'E',
'B'
]
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: [ 'G', 'O', 'X', 'C', 'D', 'E' ]
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: OK
Command: lrem bigboxstr 0 "S" | Error: [ErrorReply: WRONGTYPE Operation against a key holding the wrong kind of value]
Notes
- Use the function “lRem” of node-redis.
- Signature of the method-
lRem(key: RedisCommandArgument, count: number, element: RedisCommandArgument)
// Redis LREM Command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class Lrem {
public static void main(String[] args) {
// Create connection pool
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Create list and push items
*
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
long rpushResult = jedis.rpush("bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O");
System.out.println("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " + rpushResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
* 2) "I"
* 3) "G"
* 4) "B"
* 5) "O"
* 6) "X"
* 7) "C"
* 8) "O"
* 9) "D"
* 10) "E"
* 11) "B"
* 12) "I"
* 13) "O"
*/
List<String> lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Remove 2 occurrences of "B" starting from the Left/HEAD
*
* Command: lrem bigboxlist 2 "B"
* Result: (integer) 2
*/
long lremResult = jedis.lrem("bigboxlist", 2, "B");
System.out.println("Command: lrem bigboxlist 2 "B" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "O"
* 7) "D"
* 8) "E"
* 9) "B"
* 10) "I"
* 11) "O"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Remove 2 occurrences of "O" starting from the Right/TAIL
*
* Command: lrem bigboxlist -2 "O"
* Result: (integer) 2
*/
lremResult = jedis.lrem("bigboxlist", -2, "O");
System.out.println("Command: lrem bigboxlist -2 "O" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "D"
* 7) "E"
* 8) "B"
* 9) "I"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Remove all occurrences of "I"
*
* Command: lrem bigboxlist 0 "I"
* Result: (integer) 2
*/
lremResult = jedis.lrem("bigboxlist", 0, "I");
System.out.println("Command: lrem bigboxlist 0 "I" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
* 7) "B"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Try to remove 1000 occurrences of "B" starting from the HEAD
* Only 1 occurrence removed as there was only 1 "B" in the list
*
* Command: lrem bigboxlist 1000 "B"
* Result: (integer) 1
*/
lremResult = jedis.lrem("bigboxlist", 1000, "B");
System.out.println("Command: lrem bigboxlist 1000 "B" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Try to delete a non existing item
*
* Command: lrem bigboxlist 0 "non existing item"
* Result: (integer) 0
*/
lremResult = jedis.lrem("bigboxlist", 0, "non existing item");
System.out.println("Command: lrem bigboxlist 0 "non existing item" | Result: " + lremResult);
/**
* Try to delete from a non existing list
* It is treated as an empty list and returns zero(0)
*
* Command: lrem nonexistinglist 0 A
* Result: (integer) 0
*/
lremResult = jedis.lrem("nonexistinglist", 0, "A");
System.out.println("Command: lrem nonexistinglist 0 A | Result: " + lremResult);
/**
* Set some string value
*
* Command: set bigboxstr "Some str value"
* Result: OK
*/
String setResult = jedis.set("bigboxstr", "Some str value");
System.out.println("Command: set bigboxstr "Some str value" | Result: " + setResult);
/**
* Try to use LREM on a string
* We get an error
*
* Command: lrem bigboxstr 0 "S"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
lremResult = jedis.lrem("bigboxstr", 0, "S");
System.out.println("Command: lrem bigboxstr 0 "S" | Result: " + lremResult);
} catch (Exception e) {
System.out.println("Command: lrem bigboxstr 0 "S" | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [B, I, G, B, O, X, C, O, D, E, B, I, O]
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [I, G, O, X, C, O, D, E, B, I, O]
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [I, G, O, X, C, D, E, B, I]
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: [G, O, X, C, D, E, B]
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: [G, O, X, C, D, E]
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: OK
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lrem” from Jedis package.
- The signature of the method is-
public long lrem(final String key, final long count, final String value)
// Redis LREM command examples in C#
using StackExchange.Redis;
namespace Lrem
{
internal class Program
{
static void Main(string[] args)
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase rdb = redis.GetDatabase();
/**
* Create list and push items
*
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
long rpushResult = rdb.ListRightPush("bigboxlist", new RedisValue[] { "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O" });
Console.WriteLine("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " + rpushResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
* 2) "I"
* 3) "G"
* 4) "B"
* 5) "O"
* 6) "X"
* 7) "C"
* 8) "O"
* 9) "D"
* 10) "E"
* 11) "B"
* 12) "I"
* 13) "O"
*/
RedisValue[] lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Remove 2 occurrences of "B" starting from the Left/HEAD
*
* Command: lrem bigboxlist 2 "B"
* Result: (integer) 2
*/
long lremResult = rdb.ListRemove("bigboxlist", "B", 2);
Console.WriteLine("Command: lrem bigboxlist 2 "B" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "O"
* 7) "D"
* 8) "E"
* 9) "B"
* 10) "I"
* 11) "O"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Remove 2 occurrences of "O" starting from the Right/TAIL
*
* Command: lrem bigboxlist -2 "O"
* Result: (integer) 2
*/
lremResult = rdb.ListRemove("bigboxlist", "O", -2);
Console.WriteLine("Command: lrem bigboxlist -2 "O" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "D"
* 7) "E"
* 8) "B"
* 9) "I"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Remove all occurrences of "I"
*
* Command: lrem bigboxlist 0 "I"
* Result: (integer) 2
*/
lremResult = rdb.ListRemove("bigboxlist", "I", 0);
Console.WriteLine("Command: lrem bigboxlist 0 "I" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
* 7) "B"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Try to remove 1000 occurrences of "B" starting from the HEAD
* Only 1 occurrence removed as there was only 1 "B" in the list
*
* Command: lrem bigboxlist 1000 "B"
* Result: (integer) 1
*/
lremResult = rdb.ListRemove("bigboxlist", "B", 1000);
Console.WriteLine("Command: lrem bigboxlist 1000 "B" | Result: " + lremResult);
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Try to delete a non existing item
*
* Command: lrem bigboxlist 0 "non existing item"
* Result: (integer) 0
*/
lremResult = rdb.ListRemove("bigboxlist", "non existing item", 0);
Console.WriteLine("Command: lrem bigboxlist 0 "non existing item" | Result: " + lremResult);
/**
* Try to delete from a non existing list
* It is treated as an empty list and returns zero(0)
*
* Command: lrem nonexistinglist 0 A
* Result: (integer) 0
*/
lremResult = rdb.ListRemove("nonexistinglist", "A", 0);
Console.WriteLine("Command: lrem nonexistinglist 0 A | Result: " + lremResult);
/**
* Set some string value
*
* Command: set bigboxstr "Some str value"
* Result: OK
*/
bool setResult = rdb.StringSet("bigboxstr", "Some str value");
Console.WriteLine("Command: set bigboxstr "Some str value" | Result: " + setResult);
/**
* Try to use LREM on a string
* We get an error
*
* Command: lrem bigboxstr 0 "S"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try
{
lremResult = rdb.ListRemove("bigboxstr", "S", 0);
Console.WriteLine("Command: lrem bigboxstr 0 "S" | Result: " + lremResult);
}
catch (Exception e)
{
Console.WriteLine("Command: lrem bigboxstr 0 "S" | Error: " + e.Message);
}
}
}
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: B, I, G, B, O, X, C, O, D, E, B, I, O
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: I, G, O, X, C, O, D, E, B, I, O
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: I, G, O, X, C, D, E, B, I
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: G, O, X, C, D, E, B
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: G, O, X, C, D, E
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: True
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ListRemove” from StackExchange.Redis.
- Signature of the method is-
long ListRemove(RedisKey key, RedisValue value, long count = 0, CommandFlags flags = CommandFlags.None)
<?php
// Redis LREM command example in PHP
require 'vendor/autoload.php';
// Connect to Redis
$redisClient = new PredisClient([
'scheme' => 'tcp',
'host' => 'localhost',
'port' => 6379,
]);
/**
* Create list and push items
*
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
$commandResult = $redisClient->rpush("bigboxlist", ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]);
echo "Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " . $commandResult . "n";
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B"
* 2) "I"
* 3) "G"
* 4) "B"
* 5) "O"
* 6) "X"
* 7) "C"
* 8) "O"
* 9) "D"
* 10) "E"
* 11) "B"
* 12) "I"
* 13) "O"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Remove 2 occurrences of "B" starting from the Left/HEAD
*
* Command: lrem bigboxlist 2 "B"
* Result: (integer) 2
*/
$commandResult = $redisClient->lrem("bigboxlist", 2, "B");
echo "Command: lrem bigboxlist 2 "B" | Result: " . $commandResult . "n";
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "O"
* 7) "D"
* 8) "E"
* 9) "B"
* 10) "I"
* 11) "O"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Remove 2 occurrences of "O" starting from the Right/TAIL
*
* Command: lrem bigboxlist -2 "O"
* Result: (integer) 2
*/
$commandResult = $redisClient->lrem("bigboxlist", -2, "O");
echo "Command: lrem bigboxlist -2 "O" | Result: " . $commandResult . "n";
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "I"
* 2) "G"
* 3) "O"
* 4) "X"
* 5) "C"
* 6) "D"
* 7) "E"
* 8) "B"
* 9) "I"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Remove all occurrences of "I"
*
* Command: lrem bigboxlist 0 "I"
* Result: (integer) 2
*/
$commandResult = $redisClient->lrem("bigboxlist", 0, "I");
echo "Command: lrem bigboxlist 0 "I" | Result: " . $commandResult . "n";
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
* 7) "B"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Try to remove 1000 occurrences of "B" starting from the HEAD
* Only 1 occurrence removed as there was only 1 "B" in the list
*
* Command: lrem bigboxlist 1000 "B"
* Result: (integer) 1
*/
$commandResult = $redisClient->lrem("bigboxlist", 1000, "B");
echo "Command: lrem bigboxlist 1000 "B" | Result: " . $commandResult . "n";
/**
* Check list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "G"
* 2) "O"
* 3) "X"
* 4) "C"
* 5) "D"
* 6) "E"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Try to delete a non existing item
*
* Command: lrem bigboxlist 0 "non existing item"
* Result: (integer) 0
*/
$commandResult = $redisClient->lrem("bigboxlist", 0, "non existing item");
echo "Command: lrem bigboxlist 0 "non existing item" | Result: " . $commandResult . "n";
/**
* Try to delete from a non existing list
* It is treated as an empty list and returns zero(0)
*
* Command: lrem nonexistinglist 0 A
* Result: (integer) 0
*/
$commandResult = $redisClient->lrem("nonexistinglist", 0, "A");
echo "Command: lrem nonexistinglist 0 A | Result: " . $commandResult . "n";
/**
* Set some string value
*
* Command: set bigboxstr "Some str value"
* Result: OK
*/
$commandResult = $redisClient->set("bigboxstr", "Some str value");
echo "Command: set bigboxstr "Some str value" | Result: " . $commandResult . "n";
/**
* Try to use LREM on a string
* We get an error
*
* Command: lrem bigboxstr 0 "S"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
$commandResult = $redisClient->lrem("bigboxstr", 0, "S");
echo "Command: lrem bigboxstr 0 "S" | Result: " . $commandResult . "n";
} catch (Exception $e) {
echo "Command: lrem bigboxstr 0 "S" | Error: " . $e->getMessage() . "n";
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => I
[2] => G
[3] => B
[4] => O
[5] => X
[6] => C
[7] => O
[8] => D
[9] => E
[10] => B
[11] => I
[12] => O
)
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => I
[1] => G
[2] => O
[3] => X
[4] => C
[5] => O
[6] => D
[7] => E
[8] => B
[9] => I
[10] => O
)
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => I
[1] => G
[2] => O
[3] => X
[4] => C
[5] => D
[6] => E
[7] => B
[8] => I
)
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => G
[1] => O
[2] => X
[3] => C
[4] => D
[5] => E
[6] => B
)
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => G
[1] => O
[2] => X
[3] => C
[4] => D
[5] => E
)
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: OK
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “lrem” of predis.
- Signature of the method is-
lrem(string $key, int $count, string $value): int
# Redis LREM command example in Python
import redis
import time
# Create Redis client
redisClient = redis.Redis(host='localhost', port=6379,
username='default', password='',
decode_responses=True)
# Create list and push items
# Command: rpush bigboxlist B I G B O X C O D E B I O
# Result: (integer) 13
commandResult = redisClient.rpush("bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O")
print("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B"
# 2) "I"
# 3) "G"
# 4) "B"
# 5) "O"
# 6) "X"
# 7) "C"
# 8) "O"
# 9) "D"
# 10) "E"
# 11) "B"
# 12) "I"
# 13) "O"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Remove 2 occurrences of "B" starting from the Left/HEAD
# Command: lrem bigboxlist 2 "B"
# Result: (integer) 2
commandResult = redisClient.lrem("bigboxlist", 2, "B")
print("Command: lrem bigboxlist 2 "B" | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "I"
# 2) "G"
# 3) "O"
# 4) "X"
# 5) "C"
# 6) "O"
# 7) "D"
# 8) "E"
# 9) "B"
# 10) "I"
# 11) "O"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Remove 2 occurrences of "O" starting from the Right/TAIL
# Command: lrem bigboxlist -2 "O"
# Result: (integer) 2
commandResult = redisClient.lrem("bigboxlist", -2, "O")
print("Command: lrem bigboxlist -2 "O" | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "I"
# 2) "G"
# 3) "O"
# 4) "X"
# 5) "C"
# 6) "D"
# 7) "E"
# 8) "B"
# 9) "I"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Remove all occurrences of "I"
# Command: lrem bigboxlist 0 "I"
# Result: (integer) 2
commandResult = redisClient.lrem("bigboxlist", 0, "I")
print("Command: lrem bigboxlist 0 "I" | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "G"
# 2) "O"
# 3) "X"
# 4) "C"
# 5) "D"
# 6) "E"
# 7) "B"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Try to remove 1000 occurrences of "B" starting from the HEAD
# Only 1 occurrence removed as there was only 1 "B" in the list
# Command: lrem bigboxlist 1000 "B"
# Result: (integer) 1
commandResult = redisClient.lrem("bigboxlist", 1000, "B")
print("Command: lrem bigboxlist 1000 "B" | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "G"
# 2) "O"
# 3) "X"
# 4) "C"
# 5) "D"
# 6) "E"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Try to delete a non existing item
# Command: lrem bigboxlist 0 "non existing item"
# Result: (integer) 0
commandResult = redisClient.lrem("bigboxlist", 0, "non existing item")
print("Command: lrem bigboxlist 0 "non existing item" | Result: {}".format(commandResult))
# Try to delete from a non existing list
# It is treated as an empty list and returns zero(0)
# Command: lrem nonexistinglist 0 A
# Result: (integer) 0
commandResult = redisClient.lrem("nonexistinglist", 0, "A")
print("Command: lrem nonexistinglist 0 A | Result: {}".format(commandResult))
# Set some string value
# Command: set bigboxstr "Some str value"
# Result: OK
commandResult = redisClient.set("bigboxstr", "Some str value")
print("Command: set bigboxstr "Some str value" | Result: {}".format(commandResult))
# Try to use LREM on a string
# We get an error
# Command: lrem bigboxstr 0 "S"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
try:
commandResult = redisClient.lrem("bigboxstr", 0, "S")
print("Command: lrem bigboxstr 0 "S" | Result: {}".format(commandResult))
except Exception as error:
print("Command: lrem bigboxstr 0 "S" | Error: ", error)
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ['B', 'I', 'G', 'B', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ['I', 'G', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ['I', 'G', 'O', 'X', 'C', 'D', 'E', 'B', 'I']
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ['G', 'O', 'X', 'C', 'D', 'E', 'B']
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: ['G', 'O', 'X', 'C', 'D', 'E']
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: True
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lrem” from redis-py.
- Signature of the method is –
def lrem(self, name: str, count: int, value: str) -> Union[Awaitable[int], int]
# Redis LREM command example in Ruby
require 'redis'
redis = Redis.new(host: "localhost", port: 6379)
# Create list and push items
# Command: rpush bigboxlist B I G B O X C O D E B I O
# Result: (integer) 13
commandResult = redis.rpush("bigboxlist", ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"])
print("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B"
# 2) "I"
# 3) "G"
# 4) "B"
# 5) "O"
# 6) "X"
# 7) "C"
# 8) "O"
# 9) "D"
# 10) "E"
# 11) "B"
# 12) "I"
# 13) "O"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Remove 2 occurrences of "B" starting from the Left/HEAD
# Command: lrem bigboxlist 2 "B"
# Result: (integer) 2
commandResult = redis.lrem("bigboxlist", 2, "B")
print("Command: lrem bigboxlist 2 "B" | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "I"
# 2) "G"
# 3) "O"
# 4) "X"
# 5) "C"
# 6) "O"
# 7) "D"
# 8) "E"
# 9) "B"
# 10) "I"
# 11) "O"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Remove 2 occurrences of "O" starting from the Right/TAIL
# Command: lrem bigboxlist -2 "O"
# Result: (integer) 2
commandResult = redis.lrem("bigboxlist", -2, "O")
print("Command: lrem bigboxlist -2 "O" | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "I"
# 2) "G"
# 3) "O"
# 4) "X"
# 5) "C"
# 6) "D"
# 7) "E"
# 8) "B"
# 9) "I"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Remove all occurrences of "I"
# Command: lrem bigboxlist 0 "I"
# Result: (integer) 2
commandResult = redis.lrem("bigboxlist", 0, "I")
print("Command: lrem bigboxlist 0 "I" | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "G"
# 2) "O"
# 3) "X"
# 4) "C"
# 5) "D"
# 6) "E"
# 7) "B"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Try to remove 1000 occurrences of "B" starting from the HEAD
# Only 1 occurrence removed as there was only 1 "B" in the list
# Command: lrem bigboxlist 1000 "B"
# Result: (integer) 1
commandResult = redis.lrem("bigboxlist", 1000, "B")
print("Command: lrem bigboxlist 1000 "B" | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "G"
# 2) "O"
# 3) "X"
# 4) "C"
# 5) "D"
# 6) "E"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Try to delete a non existing item
# Command: lrem bigboxlist 0 "non existing item"
# Result: (integer) 0
commandResult = redis.lrem("bigboxlist", 0, "non existing item")
print("Command: lrem bigboxlist 0 "non existing item" | Result: ", commandResult, "n")
# Try to delete from a non existing list
# It is treated as an empty list and returns zero(0)
# Command: lrem nonexistinglist 0 A
# Result: (integer) 0
commandResult = redis.lrem("nonexistinglist", 0, "A")
print("Command: lrem nonexistinglist 0 A | Result: ", commandResult, "n")
# Set some string value
# Command: set bigboxstr "Some str value"
# Result: OK
commandResult = redis.set("bigboxstr", "Some str value")
print("Command: set bigboxstr "Some str value" | Result: ", commandResult, "n")
# Try to use LREM on a string
# We get an error
# Command: lrem bigboxstr 0 "S"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
begin
commandResult = redis.lrem("bigboxstr", 0, "S")
print("Command: lrem bigboxstr 0 "S" | Result: ", commandResult, "n")
rescue => e
print("Command: lrem bigboxstr 0 "S" | Error: ", e)
end
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: lrem bigboxlist 2 "B" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ["I", "G", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: lrem bigboxlist -2 "O" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ["I", "G", "O", "X", "C", "D", "E", "B", "I"]
Command: lrem bigboxlist 0 "I" | Result: 2
Command: lrange bigboxlist 0 -1 | Result: ["G", "O", "X", "C", "D", "E", "B"]
Command: lrem bigboxlist 1000 "B" | Result: 1
Command: lrange bigboxlist 0 -1 | Result: ["G", "O", "X", "C", "D", "E"]
Command: lrem bigboxlist 0 "non existing item" | Result: 0
Command: lrem nonexistinglist 0 A | Result: 0
Command: set bigboxstr "Some str value" | Result: OK
Command: lrem bigboxstr 0 "S" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lrem” from the redis-rb.
- Signature of the method is-
# @param [String] key
# @param [Integer] count
# @param [String] value
# @return [Integer] the number of removed elements
def lrem(key, count, value)
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LINDEX | ![]() |
LPUSH | ![]() |
LPOP | ![]() |
LSET | ![]() |