Summary
Command Name | LPUSH |
Usage | Prepend item(s) to list |
Group | ![]() |
ACL Category | @write @list @fast |
Time Complexity | O(1) |
Flag | WRITE DENYOM FAST |
Arity | -3 |
Signature
LPUSH <key> <string_element> [ <string_element> … ]
Usage
Prepend one or more elements to the list. The element is added to the head/left of the list, this command is named LPUSH(L+PUSH).
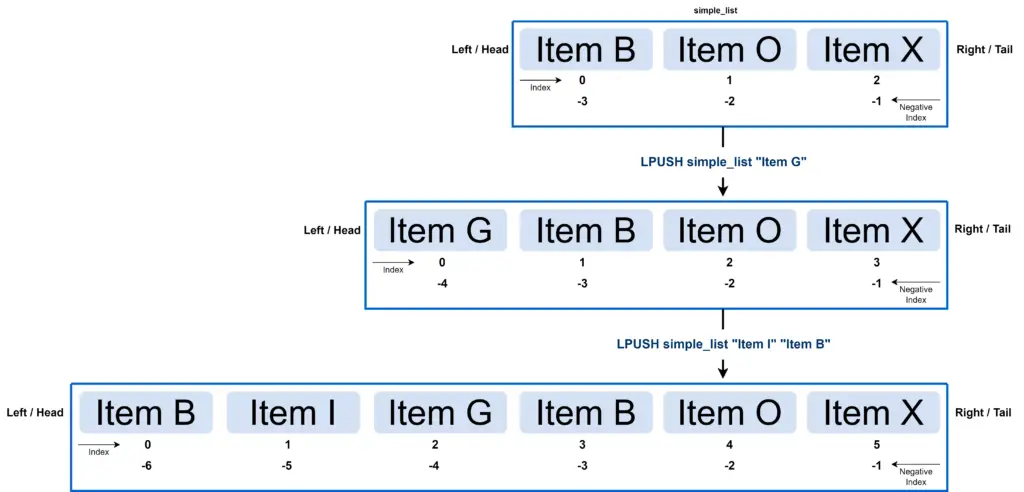
Notes
- If LPUSH command is used and a list does not exist for the key, then a list is created first and LPUSH is applied after that.
Arguments
Parameter | Description | Name | Type |
---|---|---|---|
<key> | Name of the key | key | key |
<string_element> | Element to add to the list | element | string |
Return Value
Return value | Case for the return value | Type |
---|---|---|
Integer value | Length of the list is returned, if the item is added successfully | integer |
error | If the element is not a list. | error |
Notes
- If the type of the value in the provided key is not a string then the following error is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Examples
Here are a few examples of the LPUSH command usage-
# Redis LPUSH command examples
# Push item to simplelist
# List is created as it does not already exist
127.0.0.1:6379> lpush simplelist "first item"
(integer) 1
# Prepend another element to list
127.0.0.1:6379> lpush simplelist "second item"
(integer) 2
# Check list items with LRANGE
127.0.0.1:6379> lrange simplelist 0 -1
1) "second item"
2) "first item"
# Create list and push an item to a new list
127.0.0.1:6379> lpush user:16:cart 986
(integer) 1
# Prepend item to list
127.0.0.1:6379> lpush user:16:cart 32
(integer) 2
# Prepend another item
127.0.0.1:6379> lpush user:16:cart 102
(integer) 3
# Check list items
127.0.0.1:6379> lrange user:16:cart 0 -1
1) "102"
2) "32"
3) "986"
# Prepend multiple times to list
127.0.0.1:6379> lpush user:16:cart 1049 167 348 2055
(integer) 7
# Check the list
127.0.0.1:6379> lrange user:16:cart 0 -1
1) "2055"
2) "348"
3) "167"
4) "1049"
5) "102"
6) "32"
7) "986"
# Set a string value
127.0.0.1:6379> set firstkey "my site"
OK
# Try to use lpush on a string type
# We get an error
127.0.0.1:6379> lpush firstkey "another site"
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- When we push multiple elements in the same LPUSH command, the items are pushed from left to right. So the first item is LPUSH first.
Code Implementations
Here are the usage examples of the Redis LPUSH command in different programming languages.
// Redis LPUSH command example in Golang
package main
import (
"context"
"fmt"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
// Push item to simplelist
// List is created as it does not already exist
// Command: lpush simplelist "first item"
// Result: (integer) 1
pushResult, err := rdb.LPush(ctx, "simplelist", "first item").Result()
if err != nil {
fmt.Println("Command: lpush simplelist "first item" | Error: " + err.Error())
}
fmt.Printf("Command: lpush simplelist "first item" | Result: %vn", pushResult)
// Prepend another element to list
// Command: lpush simplelist "second item"
// Result: (integer) 2
pushResult, err = rdb.LPush(ctx, "simplelist", "second item").Result()
if err != nil {
fmt.Println("Command: lpush simplelist "second item" | Error: " + err.Error())
}
fmt.Printf("Command: lpush simplelist "second item" | Result: %vn", pushResult)
// Check list items with LRANGE
// Command: lrange simplelist 0 -1
// Result:
// 1) "second item"
// 2) "first item"
listItems, err := rdb.LRange(ctx, "simplelist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange simplelist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange simplelist 0 -1 | Result: ")
for _, item := range listItems {
fmt.Println(item)
}
// Create list and push an item to a new list
// Command: lpush user:16:cart 986
// Result: (integer) 1
pushResult, err = rdb.LPush(ctx, "user:16:cart", "986").Result()
if err != nil {
fmt.Println("Command: lpush user:16:cart 986 | Error: " + err.Error())
}
fmt.Printf("Command: lpush user:16:cart 986 | Result: %vn", pushResult)
// Prepend item to list
// Command: lpush user:16:cart 32
// Result: (integer) 2
pushResult, err = rdb.LPush(ctx, "user:16:cart", "32").Result()
if err != nil {
fmt.Println("Command: lpush user:16:cart 32 | Result: " + err.Error())
}
fmt.Printf("Command: lpush user:16:cart 32 | Result: %vn", pushResult)
// Prepend another item
// Command: lpush user:16:cart 102
// Result: (integer) 3
pushResult, err = rdb.LPush(ctx, "user:16:cart", "102").Result()
if err != nil {
fmt.Println("Command: lpush user:16:cart 102 | Error: " + err.Error())
}
fmt.Printf("Command: lpush user:16:cart 102 | Result: %vn", pushResult)
// Check list items
// Command: lrange user:16:cart 0 -1
// Result:
// 1) "102"
// 2) "32"
// 3) "986"
listItems, err = rdb.LRange(ctx, "user:16:cart", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange user:16:cart 0 -1 | Error:" + err.Error())
}
fmt.Println("Command: lrange user:16:cart 0 -1 | Result:")
for _, item := range listItems {
fmt.Println(item)
}
// Prepend multiple times to list
// Command: lpush user:16:cart 1049 167 348 2055
// Result: (integer) 7
pushResult, err = rdb.LPush(ctx, "user:16:cart", "1049", "167", "348", "2055").Result()
if err != nil {
fmt.Println("Command: lpush user:16:cart 1049 167 348 2055 | Error: " + err.Error())
}
fmt.Printf("Command: lpush user:16:cart 1049 167 348 2055 | Result: %vn", pushResult)
// Check the list
// Command: lrange user:16:cart 0 -1
// Result:
// 1) "2055"
// 2) "348"
// 3) "167"
// 4) "1049"
// 5) "102"
// 6) "32"
// 7) "986"
listItems, err = rdb.LRange(ctx, "user:16:cart", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange user:16:cart 0 -1 | Error:" + err.Error())
}
fmt.Println("Command: lrange user:16:cart 0 -1 | Result:")
for _, item := range listItems {
fmt.Println(item)
}
// Set a string value
// Command: set firstkey "my site"
// Result: OK
setResult, err := rdb.Set(ctx, "firstkey", "my site", 0).Result()
if err != nil {
fmt.Println("Command: set firstkey "my site" | Error: " + err.Error())
}
fmt.Println("Command: set firstkey "my site" | Result: " + setResult)
// Try to use lpush on a string type
// We get an error
// Command: lpush firstkey "another site"
// Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
pushResult, err = rdb.LPush(ctx, "firstkey", "another site").Result()
if err != nil {
fmt.Println("Command: lpush firstkey "another site" | Error: " + err.Error())
}
fmt.Printf("Command: lpush firstkey "another site" | Result: %vn", pushResult)
}
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:
second item
first item
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
102
32
986
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:
2055
348
167
1049
102
32
986
Command: set firstkey "my site" | Result: OK
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: lpush firstkey "another site" | Result: 0
Notes
- Use “LPush” method from redis-go module.
- Signature of the method is-
LPush(ctx context.Context, key string, values …interface{}) *IntCmd
// Redis LPUSH command example in JavaScript(NodeJS)
import { createClient } from 'redis';
// Create redis client
const redisClient = createClient({
url: 'redis://default:@localhost:6379'
});
redisClient.on('error', err => console.log('Error while connecting to Redis', err));
// Connect Redis client
await redisClient.connect();
/**
* Push item to simplelist
* List is created as it does not already exist
*
* Command: lpush simplelist "first item"
* Result: (integer) 1
*/
let commandResult = await redisClient.lPush("simplelist", "first item");
console.log("Command: lpush simplelist "first item" | Result: " + commandResult);
/**
* Prepend another element to list
*
* Command: lpush simplelist "second item"
* Result: (integer) 2
*/
commandResult = await redisClient.lPush("simplelist", "second item");
console.log("Command: lpush simplelist "second item" | Result: " + commandResult);
/**
* Check list items with LRANGE
*
* Command: lrange simplelist 0 -1
* Result:
* 1) "second item"
* 2) "first item"
*/
commandResult = await redisClient.lRange("simplelist", 0, -1);
console.log("Command: lrange simplelist 0 -1 | Result: ");
for (let item of commandResult) {
console.log(item);
}
/**
* Create list and push an item to a new list
*
* Command: lpush user:16:cart 986
* Result: (integer) 1
*/
commandResult = await redisClient.lPush("user:16:cart", "986");
console.log("Command: lpush user:16:cart 986 | Result: " + commandResult);
/**
* Prepend item to list
*
* Command: lpush user:16:cart 32
* Result: (integer) 2
*/
commandResult = await redisClient.lPush("user:16:cart", "32");
console.log("Command: lpush user:16:cart 32 | Result: " + commandResult);
/**
* Prepend another item
*
* Command: lpush user:16:cart 102
* Result: (integer) 3
*/
commandResult = await redisClient.lPush("user:16:cart", "102");
console.log("Command: lpush user:16:cart 102 | Result: " + commandResult);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "102"
* 2) "32"
* 3) "986"
*/
commandResult = await redisClient.lRange("user:16:cart", 0, -1);
console.log("Command: lrange user:16:cart 0 -1 | Result:");
for (let item of commandResult) {
console.log(item);
}
/**
* Prepend multiple times to list
*
* Command: lpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
commandResult = await redisClient.lPush("user:16:cart", "1049", "167", "348", "2055");
console.log("Command: lpush user:16:cart 1049 167 348 2055 | Result: " + commandResult);
/**
* Check the list
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "2055"
* 2) "348"
* 3) "167"
* 4) "1049"
* 5) "102"
* 6) "32"
* 7) "986"
*/
commandResult = await redisClient.lRange("user:16:cart", 0, -1);
console.log("Command: lrange user:16:cart 0 -1 | Result:");
for (let item of commandResult) {
console.log(item);
}
/**
* Set a string value
*
* Command: set firstkey "my site"
* Result: OK
*/
commandResult = await redisClient.set("firstkey", "my site");
console.log("Command: set firstkey "my site" | Result: " + commandResult);
/**
* Try to use lPush on a string type
* We get an error
*
* Command: lpush firstkey "another site"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.lPush("firstkey", "another site");
console.log("Command: lpush firstkey "another site" | Result: " + commandResult);
} catch (e) {
console.log("Command: lpush firstkey "another site" | Error: " + e);
}
process.exit(0);
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:
second item
first item
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
102
32
986
Command: lpush user:16:cart 1049 167 348 2055 | Result: 4
Command: lrange user:16:cart 0 -1 | Result:
1049
102
32
986
Command: set firstkey "my site" | Result: OK
Command: lpush firstkey "another site" | Error: Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use function “lPush” of node-redis for using Redis LPUSH command.
// Redis LPUSH Command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class Lpush {
public static void main(String[] args) {
// Create connection pool
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Push item to simplelist
* List is created as it does not already exist
*
* Command: lpush simplelist "first item"
* Result: (integer) 1
*/
long pushResult = jedis.lpush("simplelist", "first item");
System.out.println("Command: lpush simplelist "first item" | Result: " + pushResult);
/**
* Prepend another element to list
*
* Command: lpush simplelist "second item"
* Result: (integer) 2
*/
pushResult = jedis.lpush("simplelist", "second item");
System.out.println("Command: lpush simplelist "second item" | Result: " + pushResult);
/**
* Check list items with LRANGE
*
* Command: lrange simplelist 0 -1
* Result:
* 1) "second item"
* 2) "first item"
*/
List<String> listItems = jedis.lrange("simplelist", 0, -1);
System.out.println("Command: lrange simplelist 0 -1 | Result: ");
for (String item : listItems) {
System.out.println(item);
}
/**
* Create list and push an item to a new list
*
* Command: lpush user:16:cart 986
* Result: (integer) 1
*/
pushResult = jedis.lpush("user:16:cart", "986");
System.out.println("Command: lpush user:16:cart 986 | Result: " + pushResult);
/**
* Prepend item to list
*
* Command: lpush user:16:cart 32
* Result: (integer) 2
*/
pushResult = jedis.lpush("user:16:cart", "32");
System.out.println("Command: lpush user:16:cart 32 | Result: " + pushResult);
/**
* Prepend another item
*
* Command: lpush user:16:cart 102
* Result: (integer) 3
*/
pushResult = jedis.lpush("user:16:cart", "102");
System.out.println("Command: lpush user:16:cart 102 | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "102"
* 2) "32"
* 3) "986"
*/
listItems = jedis.lrange("user:16:cart", 0, -1);
System.out.println("Command: lrange user:16:cart 0 -1 | Result:");
for (String item : listItems) {
System.out.println(item);
}
/**
* Prepend multiple times to list
*
* Command: lpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
pushResult = jedis.lpush("user:16:cart", "1049", "167", "348", "2055");
System.out.println("Command: lpush user:16:cart 1049 167 348 2055 | Result: " + pushResult);
/**
* Check the list
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "2055"
* 2) "348"
* 3) "167"
* 4) "1049"
* 5) "102"
* 6) "32"
* 7) "986"
*/
listItems = jedis.lrange("user:16:cart", 0, -1);
System.out.println("Command: lrange user:16:cart 0 -1 | Result:");
for (String item : listItems) {
System.out.println(item);
}
/**
* Set a string value
*
* Command: set firstkey "my site"
* Result: OK
*/
String setResult = jedis.set("firstkey", "my site");
System.out.println("Command: set firstkey "my site" | Result: " + setResult);
/**
* Try to use lpush on a string type
* We get an error
*
* Command: lpush firstkey "another site"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
pushResult = jedis.lpush("firstkey", "another site");
System.out.println("Command: lpush firstkey "another site" | Result: " + pushResult);
} catch (Exception e) {
System.out.println("Command: lpush firstkey "another site" | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:
second item
first item
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
102
32
986
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:
2055
348
167
1049
102
32
986
Command: set firstkey "my site" | Result: OK
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpush” from Jedis package.
- The signature of the “lpush” is-
public long lpush(final String key, final String… strings)
or
public long lpush(byte[] key, byte[]… args)
// Redis LPUSH command examples in C#
using StackExchange.Redis;
namespace Lpush
{
internal class Program
{
static void Main(string[] args)
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase rdb = redis.GetDatabase();
/**
* Push item to simplelist
* List is created as it does not already exist
*
* Command: lpush simplelist "first item"
* Result: (integer) 1
*/
long pushResult = rdb.ListLeftPush("simplelist", "first item");
Console.WriteLine("Command: lpush simplelist "first item" | Result: " + pushResult);
/**
* Prepend another element to list
*
* Command: lpush simplelist "second item"
* Result: (integer) 2
*/
pushResult = rdb.ListLeftPush("simplelist", "second item");
Console.WriteLine("Command: lpush simplelist "second item" | Result: " + pushResult);
/**
* Check list items with LRANGE
*
* Command: lrange simplelist 0 -1
* Result:
* 1) "second item"
* 2) "first item"
*/
RedisValue[] listItems = rdb.ListRange("simplelist", 0, -1);
Console.WriteLine("Command: lrange simplelist 0 -1 | Result: ");
foreach (var item in listItems)
{
Console.WriteLine(item);
}
/**
* Create list and push an item to a new list
*
* Command: lpush user:16:cart 986
* Result: (integer) 1
*/
pushResult = rdb.ListLeftPush("user:16:cart", "986");
Console.WriteLine("Command: lpush user:16:cart 986 | Result: " + pushResult);
/**
* Prepend item to list
*
* Command: lpush user:16:cart 32
* Result: (integer) 2
*/
pushResult = rdb.ListLeftPush("user:16:cart", "32");
Console.WriteLine("Command: lpush user:16:cart 32 | Result: " + pushResult);
/**
* Prepend another item
*
* Command: lpush user:16:cart 102
* Result: (integer) 3
*/
pushResult = rdb.ListLeftPush("user:16:cart", "102");
Console.WriteLine("Command: lpush user:16:cart 102 | Result: " + pushResult);
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "102"
* 2) "32"
* 3) "986"
*/
listItems = rdb.ListRange("user:16:cart", 0, -1);
Console.WriteLine("Command: lrange user:16:cart 0 -1 | Result:");
foreach (var item in listItems)
{
Console.WriteLine(item);
}
/**
* Prepend multiple times to list
*
* Command: lpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
pushResult = rdb.ListLeftPush("user:16:cart", new RedisValue[] { "1049", "167", "348", "2055" });
Console.WriteLine("Command: lpush user:16:cart 1049 167 348 2055 | Result: " + pushResult);
/**
* Check the list
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "2055"
* 2) "348"
* 3) "167"
* 4) "1049"
* 5) "102"
* 6) "32"
* 7) "986"
*/
listItems = rdb.ListRange("user:16:cart", 0, -1);
Console.WriteLine("Command: lrange user:16:cart 0 -1 | Result:");
foreach (var item in listItems)
{
Console.WriteLine(item);
}
/**
* Set a string value
*
* Command: set firstkey "my site"
* Result: OK
*/
bool setResult = rdb.StringSet("firstkey", "my site");
Console.WriteLine("Command: set firstkey "my site" | Result: " + setResult);
/**
* Try to use lpush on a string type
* We get an error
*
* Command: lpush firstkey "another site"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try
{
pushResult = rdb.ListLeftPush("firstkey", "another site");
Console.WriteLine("Command: lpush firstkey "another site" | Result: " + pushResult);
}
catch (Exception e)
{
Console.WriteLine("Command: lpush firstkey "another site" | Error: " + e.Message);
}
}
}
}
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:
second item
first item
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
102
32
986
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:
2055
348
167
1049
102
32
986
Command: set firstkey "my site" | Result: True
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ListLeftPush” from StackExchange.Redis.
- Signature of the method is-
long ListLeftPush(RedisKey key, RedisValue value, When when = When.Always, CommandFlags flags = CommandFlags.None)
or
long ListLeftPush(RedisKey key, RedisValue[] values, When when = When.Always, CommandFlags flags = CommandFlags.None)
<?php
// Redis LPUSH command example in PHP
require 'vendor/autoload.php';
// Connect to Redis
$redisClient = new PredisClient([
'scheme' => 'tcp',
'host' => 'localhost',
'port' => 6379,
]);
/**
* Push item to simplelist
* List is created as it does not already exist
*
* Command: lpush simplelist "first item"
* Result: (integer) 1
*/
$commandResult = $redisClient->lpush("simplelist", ["first item"]);
echo "Command: lpush simplelist "first item" | Result: " . $commandResult . "n";
/**
* Prepend another element to list
*
* Command: lpush simplelist "second item"
* Result: (integer) 2
*/
$commandResult = $redisClient->lpush("simplelist", ["second item"]);
echo "Command: lpush simplelist "second item" | Result: " . $commandResult . "n";
/**
* Check list items with LRANGE
*
* Command: lrange simplelist 0 -1
* Result:
* 1) "second item"
* 2) "first item"
*/
$commandResult = $redisClient->lrange("simplelist", 0, -1);
echo "Command: lrange simplelist 0 -1 | Result:n";
print_r($commandResult);
/**
* Create list and push an item to a new list
*
* Command: lpush user:16:cart 986
* Result: (integer) 1
*/
$commandResult = $redisClient->lpush("user:16:cart", ["986"]);
echo "Command: lpush user:16:cart 986 | Result: " . $commandResult . "n";
/**
* Prepend item to list
*
* Command: lpush user:16:cart 32
* Result: (integer) 2
*/
$commandResult = $redisClient->lpush("user:16:cart", ["32"]);
echo "Command: lpush user:16:cart 32 | Result: " . $commandResult . "n";
/**
* Prepend another item
*
* Command: lpush user:16:cart 102
* Result: (integer) 3
*/
$commandResult = $redisClient->lpush("user:16:cart", ["102"]);
echo "Command: lpush user:16:cart 102 | Result: " . $commandResult . "n";
/**
* Check list items
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "102"
* 2) "32"
* 3) "986"
*/
$commandResult = $redisClient->lrange("user:16:cart", 0, -1);
echo "Command: lrange user:16:cart 0 -1 | Result:n";
print_r($commandResult);
/**
* Prepend multiple times to list
*
* Command: lpush user:16:cart 1049 167 348 2055
* Result: (integer) 7
*/
$commandResult = $redisClient->lpush("user:16:cart", ["1049", "167", "348", "2055"]);
echo "Command: lpush user:16:cart 1049 167 348 2055 | Result: " . $commandResult . "n";
/**
* Check the list
*
* Command: lrange user:16:cart 0 -1
* Result:
* 1) "2055"
* 2) "348"
* 3) "167"
* 4) "1049"
* 5) "102"
* 6) "32"
* 7) "986"
*/
$commandResult = $redisClient->lrange("user:16:cart", 0, -1);
echo "Command: lrange user:16:cart 0 -1 | Result:n";
print_r($commandResult);
/**
* Set a string value
*
* Command: set firstkey "my site"
* Result: OK
*/
$commandResult = $redisClient->set("firstkey", "my site");
echo "Command: set firstkey "my site" | Result: " . $commandResult . "n";
/**
* Try to use lPush on a string type
* We get an error
*
* Command: lpush firstkey "another site"
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
$commandResult = $redisClient->lpush("firstkey", ["another site"]);
echo "Command: lpush firstkey "another site" | Result: " . $commandResult . "n";
} catch (Exception $e) {
echo "Command: lpush firstkey "another site" | Error: " . $e->getMessage() . "n";
}
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:
Array
(
[0] => second item
[1] => first item
)
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:
Array
(
[0] => 102
[1] => 32
[2] => 986
)
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result:
Array
(
[0] => 2055
[1] => 348
[2] => 167
[3] => 1049
[4] => 102
[5] => 32
[6] => 986
)
Command: set firstkey "my site" | Result: OK
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “lpush” of predis.
- Signature of the method is-
lpush(string $key, array $values): int
# Redis LPUSH command example in Python
import redis
import time
# Create Redis client
redisClient = redis.Redis(host='localhost', port=6379,
username='default', password='',
decode_responses=True)
# Push item to simplelist
# List is created as it does not already exist
# Command: lpush simplelist "first item"
# Result: (integer) 1
commandResult = redisClient.lpush("simplelist", "first item")
print("Command: lpush simplelist "first item" | Result: {}".format(commandResult))
# Prepend another element to list
# Command: lpush simplelist "second item"
# Result: (integer) 2
commandResult = redisClient.lpush("simplelist", "second item")
print("Command: lpush simplelist "second item" | Result: {}".format(commandResult))
# Check list items with LRANGE
# Command: lrange simplelist 0 -1
# Result:
# 1) "second item"
# 2) "first item"
commandResult = redisClient.lrange("simplelist", 0, -1)
print("Command: lrange simplelist 0 -1 | Result:{}".format(commandResult))
# Create list and push an item to a new list
# Command: lpush user:16:cart 986
# Result: (integer) 1
commandResult = redisClient.lpush("user:16:cart", "986")
print("Command: lpush user:16:cart 986 | Result: {}".format(commandResult))
# Prepend item to list
# Command: lpush user:16:cart 32
# Result: (integer) 2
commandResult = redisClient.lpush("user:16:cart", "32")
print("Command: lpush user:16:cart 32 | Result: {}".format(commandResult))
# Prepend another item
# Command: lpush user:16:cart 102
# Result: (integer) 3
commandResult = redisClient.lpush("user:16:cart", "102")
print("Command: lpush user:16:cart 102 | Result: {}".format(commandResult))
# Check list items
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "102"
# 2) "32"
# 3) "986"
commandResult = redisClient.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result:{}".format(commandResult))
# Prepend multiple times to list
# Command: lpush user:16:cart 1049 167 348 2055
# Result: (integer) 7
commandResult = redisClient.lpush("user:16:cart", "1049", "167", "348", "2055")
print("Command: lpush user:16:cart 1049 167 348 2055 | Result: {}".format(commandResult))
# Check the list
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "2055"
# 2) "348"
# 3) "167"
# 4) "1049"
# 5) "102"
# 6) "32"
# 7) "986"
commandResult = redisClient.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result: {}".format(commandResult))
# Set a string value
# Command: set firstkey "my site"
# Result: OK
commandResult = redisClient.set("firstkey", "my site")
print("Command: set firstkey "my site" | Result: {}".format(commandResult))
# Try to use lPush on a string type
# We get an error
# Command: lpush firstkey "another site"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
try:
commandResult = redisClient.lpush("firstkey", "another site")
print("Command: lpush firstkey "another site" | Result: {}".format(commandResult))
except Exception as error:
print("Command: lpush firstkey "another site" | Error: ", error)
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:['second item', 'first item']
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:['102', '32', '986']
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: ['2055', '348', '167', '1049', '102', '32', '986']
Command: set firstkey "my site" | Result: True
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpush” from redis-py.
- Signature of the method is –
def lpush(self, name: str, *values: FieldT) -> Union[Awaitable[int], int]
# Redis LPUSH command example in Ruby
require 'redis'
redis = Redis.new(host: "localhost", port: 6379)
# Push item to simplelist
# List is created as it does not already exist
# Command: lpush simplelist "first item"
# Result: (integer) 1
commandResult = redis.lpush("simplelist", "first item")
print("Command: lpush simplelist "first item" | Result: ", commandResult, "n")
# Prepend another element to list
# Command: lpush simplelist "second item"
# Result: (integer) 2
commandResult = redis.lpush("simplelist", "second item")
print("Command: lpush simplelist "second item" | Result: ", commandResult, "n")
# Check list items with LRANGE
# Command: lrange simplelist 0 -1
# Result:
# 1) "second item"
# 2) "first item"
commandResult = redis.lrange("simplelist", 0, -1)
print("Command: lrange simplelist 0 -1 | Result:", commandResult, "n")
# Create list and push an item to a new list
# Command: lpush user:16:cart 986
# Result: (integer) 1
commandResult = redis.lpush("user:16:cart", "986")
print("Command: lpush user:16:cart 986 | Result: ", commandResult, "n")
# Prepend item to list
# Command: lpush user:16:cart 32
# Result: (integer) 2
commandResult = redis.lpush("user:16:cart", "32")
print("Command: lpush user:16:cart 32 | Result: ", commandResult, "n")
# Prepend another item
# Command: lpush user:16:cart 102
# Result: (integer) 3
commandResult = redis.lpush("user:16:cart", "102")
print("Command: lpush user:16:cart 102 | Result: ", commandResult, "n")
# Check list items
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "102"
# 2) "32"
# 3) "986"
commandResult = redis.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result:", commandResult, "n")
# Prepend multiple times to list
# Command: lpush user:16:cart 1049 167 348 2055
# Result: (integer) 7
commandResult = redis.lpush("user:16:cart", ["1049", "167", "348", "2055"])
print("Command: lpush user:16:cart 1049 167 348 2055 | Result: ", commandResult, "n")
# Check the list
# Command: lrange user:16:cart 0 -1
# Result:
# 1) "2055"
# 2) "348"
# 3) "167"
# 4) "1049"
# 5) "102"
# 6) "32"
# 7) "986"
commandResult = redis.lrange("user:16:cart", 0, -1)
print("Command: lrange user:16:cart 0 -1 | Result: ", commandResult, "n")
# Set a string value
# Command: set firstkey "my site"
# Result: OK
commandResult = redis.set("firstkey", "my site")
print("Command: set firstkey "my site" | Result: ", commandResult, "n")
# Try to use lPush on a string type
# We get an error
# Command: lpush firstkey "another site"
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
begin
commandResult = redis.lpush("firstkey", "another site")
print("Command: lpush firstkey "another site" | Result: ", commandResult, "n")
rescue => e
print("Command: lpush firstkey "another site" | Error: ", e, "n")
end
Output:
Command: lpush simplelist "first item" | Result: 1
Command: lpush simplelist "second item" | Result: 2
Command: lrange simplelist 0 -1 | Result:["second item", "first item"]
Command: lpush user:16:cart 986 | Result: 1
Command: lpush user:16:cart 32 | Result: 2
Command: lpush user:16:cart 102 | Result: 3
Command: lrange user:16:cart 0 -1 | Result:["102", "32", "986"]
Command: lpush user:16:cart 1049 167 348 2055 | Result: 7
Command: lrange user:16:cart 0 -1 | Result: ["2055", "348", "167", "1049", "102", "32", "986"]
Command: set firstkey "my site" | Result: OK
Command: lpush firstkey "another site" | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpush” from the redis-rb.
- Signature of the method is-
def lpush(key, value)
The value can be “string” or “Array<String>”.
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LRANGE | ![]() |
RPUSH | ![]() |