Summary
Command Name | LPOP |
Usage | Pop item from Left/HEAD of list |
Group | ![]() |
ACL Category | @write @list @fast |
Time Complexity | O(N) |
Flag | WRITE FAST |
Arity | -2 |
Notes
- In the time complexity, N is the number of returned items.
Signature
LPOP <key> [ count ]
Usage
LPOP removes the first element from the Left/HEAD of the list and then returns it. Count is an optional argument provided for the command, this is used to pop multiple items.
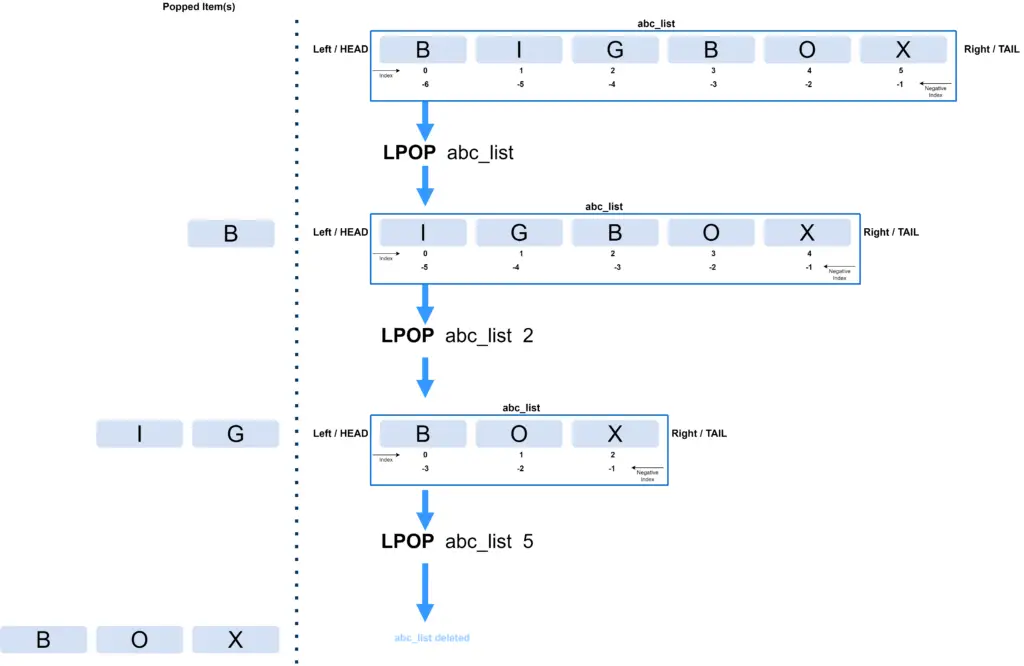
Notes
- If the list has no element left after executing the LPOP command, then the list is deleted after the item(s) popped.
Arguments
Parameter | Description | Name | Type | Optional |
---|---|---|---|---|
<key> | key name of the list | key | key | |
<count> | Number of items to return | count | integer | True |
Notes
- <count> can not be less than Zero(0). If count <count> is less than zero(0), then following error is returned-
(error) ERR value is out of range, must be positive
Return Value
Return value | Case for the return value | Type |
---|---|---|
First item | If <count> is not provided and the command was successful | string |
List of item | If the <count> is provided and the command was successful | array[string] |
(nil) | If key does not exist | null |
error | If the key exists but the data type of the key is not a list | error |
Notes
- If the command is applied on ken which is not of type list, then the following error is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Command Examples
Here are a few examples of the LPOP command usage-
# Redis LPOP command examples
# Push elements and create list
127.0.0.1:6379> rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
(integer) 5
# Pop 1 item from HEAD
127.0.0.1:6379> lpop bigboxlist
"Item A"
# Pop 2 items from HEAD
127.0.0.1:6379> lpop bigboxlist 2
1) "Item B"
2) "Item C"
# Try to pass negative value for the count
# We get an error message
127.0.0.1:6379> lpop bigboxlist -2
(error) ERR value is out of range, must be positive
# Pass Zero(0) as count
# Empty array is returned
127.0.0.1:6379> lpop bigboxlist 0
(empty array)
# Try to pop 5 items from list
# The list has only 2 items
# 2 items are popped and command is successful
127.0.0.1:6379> lpop bigboxlist 5
1) "Item D"
2) "Item E"
# Check if list exits after all items are popped
# List does not exist any more
127.0.0.1:6379> exists bigboxlist
(integer) 0
# Try to pop from a non existing list
# returns (nil)
127.0.0.1:6379> lpop bigboxlist
(nil)
# Create an string value
127.0.0.1:6379> set bigboxstr "my string value here"
OK
# Try to apply LPOP on the string
# Returns an error message
127.0.0.1:6379> lpop bigboxstr
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- If Zero(0) is passed as <count> then an empty list is returned.
Code Implementations
Here are the usage examples of the Redis LPOP command in different programming languages.
// Redis LPOP command example in Golang
package main
import (
"context"
"fmt"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
// Push elements and create list
// Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
// Result: (integer) 5
rpushResult, err := rdb.RPush(ctx, "bigboxlist", "Item A", "Item B", "Item C", "Item D", "Item E").Result()
if err != nil {
fmt.Println("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: ", rpushResult)
// Check item list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "Item A"
// 2) "Item B"
// 3) "Item C"
// 4) "Item D"
// 5) "Item E"
lrangeResult, err := rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Pop 1 item from HEAD
// Command: lpop bigboxlist
// Result: "Item A"
lpopResult, err := rdb.LPop(ctx, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist | Result: " + lpopResult)
// Pop 2 items from HEAD
// Command: lpop bigboxlist 2
// Result:
// 1) "Item B"
// 2) "Item C"
lpopResults, err := rdb.LPopCount(ctx, "bigboxlist", 2).Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist 2 | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist 2 | Result: ", lpopResults)
// Try to pass negative value for the count
// We get an error message
// Command: lpop bigboxlist -2
// Result: (error) ERR value is out of range, must be positive
lpopResults, err = rdb.LPopCount(ctx, "bigboxlist", -2).Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist -2 | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist -2 | Result: ", lpopResults)
// Pass Zero(0) as count
// Empty array is returned
// Command: lpop bigboxlist 0
// Result: (empty array)
lpopResults, err = rdb.LPopCount(ctx, "bigboxlist", 0).Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist 0 | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist 0 | Result: ", lpopResults)
// Try to pop 5 items from list
// The list has only 2 items
// 2 items are popped and command is successful
// Command: lpop bigboxlist 5
// Result:
// 1) "Item D"
// 2) "Item E"
lpopResults, err = rdb.LPopCount(ctx, "bigboxlist", 5).Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist 5 | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist 5 | Result: ", lpopResults)
// Check if list exits after all items are popped
// List does not exist any more
// Command: exists bigboxlist
// Result: (integer) 0
existsResult, err := rdb.Exists(ctx, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: exists bigboxlist | Error: " + err.Error())
}
fmt.Println("Command: exists bigboxlist | Result: ", existsResult)
// Try to pop from a non existing list
// returns (nil)
// Command: lpop bigboxlist
// Result: (nil)
lpopResult, err = rdb.LPop(ctx, "bigboxlist").Result()
if err != nil {
fmt.Println("Command: lpop bigboxlist | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxlist | Result: " + lpopResult)
// Create an string value
// Command: set bigboxstr "my string value here"
// Result: OK
setResult, err := rdb.Set(ctx, "bigboxstr", "my string value here", 0).Result()
if err != nil {
fmt.Println("Command: set bigboxstr "my string value here" | Error: " + err.Error())
}
fmt.Println("Command: set bigboxstr "my string value here" | Result: " + setResult)
// Try to apply LPOP on the string
// Returns an error message
// Command: lpop bigboxstr
// Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
lpopResult, err = rdb.LPop(ctx, "bigboxstr").Result()
if err != nil {
fmt.Println("Command: lpop bigboxstr | Error: " + err.Error())
}
fmt.Println("Command: lpop bigboxstr | Result: " + lpopResult)
}
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: [Item A Item B Item C Item D Item E]
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: [Item B Item C]
Command: lpop bigboxlist -2 | Error: ERR value is out of range, must be positive
Command: lpop bigboxlist -2 | Result: []
Command: lpop bigboxlist 0 | Result: []
Command: lpop bigboxlist 5 | Result: [Item D Item E]
Command: exists bigboxlist | Result: 0
Command: lpop bigboxlist | Error: redis: nil
Command: lpop bigboxlist | Result:
Command: set bigboxstr "my string value here" | Result: OK
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: lpop bigboxstr | Result:
Notes
- Use “LPop” or “LPopCount” methods from redis-go module.
- Signature of the methods is-
func (c cmdable) LPop(ctx context.Context, key string) *StringCmd
func (c cmdable) LPopCount(ctx context.Context, key string, count int) *StringSliceCmd
// Redis LPOP command example in JavaScript(NodeJS)
import { createClient } from "redis";
// Create redis client
const redisClient = createClient({
url: "redis://default:@localhost:6379",
});
redisClient.on("error", (err) =>
console.log("Error while connecting to Redis", err)
);
// Connect Redis client
await redisClient.connect();
/**
* Push elements and create list
*
* Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
* Result: (integer) 5
*/
let commandResult = await redisClient.rPush("bigboxlist", [
"Item A",
"Item B",
"Item C",
"Item D",
"Item E",
]);
console.log(
'Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: ' +
commandResult
);
/**
* Check item list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "Item A"
* 2) "Item B"
* 3) "Item C"
* 4) "Item D"
* 5) "Item E"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Pop 1 item from HEAD
*
* Command: lpop bigboxlist
* Result: "Item A"
*/
commandResult = await redisClient.lPop("bigboxlist");
console.log("Command: lpop bigboxlist | Result: " + commandResult);
/**
* Pop 2 items from HEAD
*
* Command: lpop bigboxlist 2
* Result:
* 1) "Item B"
* 2) "Item C"
*/
commandResult = await redisClient.lPopCount("bigboxlist", 2);
console.log("Command: lpop bigboxlist 2 | Result: ", commandResult);
/**
* Try to pass negative value for the count
* We get an error message
*
* Command: lpop bigboxlist -2
* Result: (error) ERR value is out of range, must be positive
*/
try {
commandResult = await redisClient.lPopCount("bigboxlist", -2);
console.log("Command: lpop bigboxlist -2 | Result: ", commandResult);
} catch (err) {
console.log("Command: lpop bigboxlist -2 | Error: ", err);
}
/**
* Pass Zero(0) as count
* Empty array is returned
*
* Command: lpop bigboxlist 0
* Result: (empty array)
*/
commandResult = await redisClient.lPopCount("bigboxlist", 0);
console.log("Command: lpop bigboxlist 0 | Result: ", commandResult);
/**
* Try to pop 5 items from list
* The list has only 2 items
* 2 items are popped and command is successful
*
* Command: lpop bigboxlist 5
* Result:
* 1) "Item D"
* 2) "Item E"
*/
commandResult = await redisClient.lPopCount("bigboxlist", 5);
console.log("Command: lpop bigboxlist 5 | Result: ", commandResult);
/**
* Check if list exits after all items are popped
* List does not exist any more
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
commandResult = await redisClient.exists("bigboxlist");
console.log("Command: exists bigboxlist | Result: " + commandResult);
/**
* Try to pop from a non existing list
* returns (nil)
*
* Command: lpop bigboxlist
* Result: (nil)
*/
commandResult = await redisClient.lPop("bigboxlist");
console.log("Command: lpop bigboxlist | Result: " + commandResult);
/**
* Create an string value
*
* Command: set bigboxstr "my string value here"
* Result: OK
*/
commandResult = await redisClient.set("bigboxstr", "my string value here");
console.log(
'Command: set bigboxstr "my string value here" | Result: ' + commandResult
);
/**
* Try to apply LPOP on the string
* Returns an error message
*
* Command: lpop bigboxstr
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.lPop("bigboxstr");
console.log("Command: lpop bigboxstr | Result: " + commandResult);
} catch (err) {
console.log("Command: lpop bigboxstr | Error: ", err);
}
process.exit(0);
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: [ 'Item A', 'Item B', 'Item C', 'Item D', 'Item E' ]
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: [ 'Item B', 'Item C' ]
Command: lpop bigboxlist -2 | Error: [ErrorReply: ERR value is out of range, must be positive]
Command: lpop bigboxlist 0 | Result: []
Command: lpop bigboxlist 5 | Result: [ 'Item D', 'Item E' ]
Command: exists bigboxlist | Result: 0
Command: lpop bigboxlist | Result: null
Command: set bigboxstr "my string value here" | Result: OK
Command: lpop bigboxstr | Error: [ErrorReply: WRONGTYPE Operation against a key holding the wrong kind of value]
Notes
- Use the function “lPop” and “lPopCount” from the package node-redis.
- Here is the signature of the method –
lPop(key: RedisCommandArgument)
lPop(key: RedisCommandArgument, count: number)
// Redis LPOP command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class Lpop {
public static void main(String[] args) {
// Create connection pool
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Push elements and create list
*
* Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
* Result: (integer) 5
*/
long rpushResult = jedis.rpush("bigboxlist", "Item A", "Item B", "Item C", "Item D", "Item E");
System.out.println("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: " + rpushResult);
/**
* Check item list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "Item A"
* 2) "Item B"
* 3) "Item C"
* 4) "Item D"
* 5) "Item E"
*/
List<String> lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Pop 1 item from HEAD
*
* Command: lpop bigboxlist
* Result: "Item A"
*/
String lpopResult = jedis.lpop("bigboxlist");
System.out.println("Command: lpop bigboxlist | Result: " + lpopResult);
/**
* Pop 2 items from HEAD
*
* Command: lpop bigboxlist 2
* Result:
* 1) "Item B"
* 2) "Item C"
*/
List<String> lpopResults = jedis.lpop("bigboxlist", 2);
System.out.println("Command: lpop bigboxlist 2 | Result: " + lpopResults.toString());
/**
* Try to pass negative value for the count
* We get an error message
*
* Command: lpop bigboxlist -2
* Result: (error) ERR value is out of range, must be positive
*/
try {
lpopResults = jedis.lpop("bigboxlist", -2);
System.out.println("Command: lpop bigboxlist -2 | Result: " + lpopResults.toString());
} catch (Exception e) {
System.out.println("Command: lpop bigboxlist -2 | Error: " + e.getMessage());
}
/**
* Pass Zero(0) as count
* Empty array is returned
*
* Command: lpop bigboxlist 0
* Result: (empty array)
*/
lpopResults = jedis.lpop("bigboxlist", 0);
System.out.println("Command: lpop bigboxlist 0 | Result: " + lpopResults.toString());
/**
* Try to pop 5 items from list
* The list has only 2 items
* 2 items are popped and command is successful
*
* Command: lpop bigboxlist 5
* Result:
* 1) "Item D"
* 2) "Item E"
*/
lpopResults = jedis.lpop("bigboxlist", 5);
System.out.println("Command: lpop bigboxlist 5 | Result: " + lpopResults.toString());
/**
* Check if list exits after all items are popped
* List does not exist any more
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
boolean existsResult = jedis.exists("bigboxlist");
System.out.println("Command: exists bigboxlist | Result: " + existsResult);
/**
* Try to pop from a non existing list
* returns (nil)
*
* Command: lpop bigboxlist
* Result: (nil)
*/
lpopResult = jedis.lpop("bigboxlist");
System.out.println("Command: lpop bigboxlist | Result: " + lpopResult);
/**
* Create an string value
*
* Command: set bigboxstr "my string value here"
* Result: OK
*/
String setResult = jedis.set("bigboxstr", "my string value here");
System.out.println("Command: set bigboxstr "my string value here" | Result: " + setResult);
/**
* Try to apply LPOP on the string
* Returns an error message
*
* Command: lpop bigboxstr
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
lpopResult = jedis.lpop("bigboxstr");
System.out.println("Command: lpop bigboxstr | Result: " + lpopResult);
} catch (Exception e) {
System.out.println("Command: lpop bigboxstr | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: [Item A, Item B, Item C, Item D, Item E]
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: [Item B, Item C]
Command: lpop bigboxlist -2 | Error: ERR value is out of range, must be positive
Command: lpop bigboxlist 0 | Result: []
Command: lpop bigboxlist 5 | Result: [Item D, Item E]
Command: exists bigboxlist | Result: false
Command: lpop bigboxlist | Result: null
Command: set bigboxstr "my string value here" | Result: OK
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpop” from Jedis package.
- This “lpop” method has 2 variations. Signatures of the methods are-
public String lpop(final String key)
public List<String> lpop(final String key, final int count)
// Redis LPOP command examples in C#
using StackExchange.Redis;
namespace Lpop
{
internal class Program
{
static void Main(string[] args)
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase rdb = redis.GetDatabase();
/**
* Push elements and create list
*
* Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
* Result: (integer) 5
*/
long rpushResult = rdb.ListRightPush("bigboxlist", new RedisValue[] { "Item A", "Item B", "Item C", "Item D", "Item E" });
Console.WriteLine("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: " + rpushResult);
/**
* Check item list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "Item A"
* 2) "Item B"
* 3) "Item C"
* 4) "Item D"
* 5) "Item E"
*/
RedisValue[] lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Pop 1 item from HEAD
*
* Command: lpop bigboxlist
* Result: "Item A"
*/
RedisValue lpopResult = rdb.ListLeftPop("bigboxlist");
Console.WriteLine("Command: lpop bigboxlist | Result: " + lpopResult);
/**
* Pop 2 items from HEAD
*
* Command: lpop bigboxlist 2
* Result:
* 1) "Item B"
* 2) "Item C"
*/
RedisValue[] lpopResults = rdb.ListLeftPop("bigboxlist", 2);
Console.WriteLine("Command: lpop bigboxlist 2 | Result: " + string.Join(", ", lpopResults));
/**
* Try to pass negative value for the count
* We get an error message
*
* Command: lpop bigboxlist -2
* Result: (error) ERR value is out of range, must be positive
*/
try
{
lpopResults = rdb.ListLeftPop("bigboxlist", -2);
Console.WriteLine("Command: lpop bigboxlist -2 | Result: " + string.Join(", ", lpopResults));
}
catch (Exception e)
{
Console.WriteLine("Command: lpop bigboxlist -2 | Error: " + e.Message);
}
/**
* Pass Zero(0) as count
* Empty array is returned
*
* Command: lpop bigboxlist 0
* Result: (empty array)
*/
lpopResult = rdb.ListLeftPop("bigboxlist", 0);
Console.WriteLine("Command: lpop bigboxlist 0 | Result: " + lpopResult);
/**
* Try to pop 5 items from list
* The list has only 2 items
* 2 items are popped and command is successful
*
* Command: lpop bigboxlist 5
* Result:
* 1) "Item D"
* 2) "Item E"
*/
lpopResults = rdb.ListLeftPop("bigboxlist", 5);
Console.WriteLine("Command: lpop bigboxlist 5 | Result: " + string.Join(", ", lpopResults));
/**
* Check if list exits after all items are popped
* List does not exist any more
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
bool existsResult = rdb.KeyExists("bigboxlist");
Console.WriteLine("Command: exists bigboxlist | Result: " + existsResult);
/**
* Try to pop from a non existing list
* returns (nil)
*
* Command: lpop bigboxlist
* Result: (nil)
*/
lpopResult = rdb.ListLeftPop("bigboxlist");
Console.WriteLine("Command: lpop bigboxlist | Result: " + lpopResult);
/**
* Create an string value
*
* Command: set bigboxstr "my string value here"
* Result: OK
*/
bool setResult = rdb.StringSet("bigboxstr", "my string value here");
Console.WriteLine("Command: set bigboxstr "my string value here" | Result: " + setResult);
/**
* Try to apply LPOP on the string
* Returns an error message
*
* Command: lpop bigboxstr
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try
{
lpopResult = rdb.ListLeftPop("bigboxstr");
Console.WriteLine("Command: lpop bigboxstr | Result: " + lpopResult);
}
catch (Exception e)
{
Console.WriteLine("Command: lpop bigboxstr | Error: " + e.Message);
}
}
}
}
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: Item A, Item B, Item C, Item D, Item E
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: Item B, Item C
Command: lpop bigboxlist -2 | Error: ERR value is out of range, must be positive
Command: lpop bigboxlist 0 | Result: Item D
Command: lpop bigboxlist 5 | Result: Item E
Command: exists bigboxlist | Result: False
Command: lpop bigboxlist | Result:
Command: set bigboxstr "my string value here" | Result: True
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the methods “ListLeftPop” from StackExchange.Redis.
- Signature of the method for LMPOP is as below –
RedisValue ListLeftPop(RedisKey key, CommandFlags flags = CommandFlags.None)
RedisValue[] ListLeftPop(RedisKey key, long count, CommandFlags flags = CommandFlags.None)
<?php
// Redis LPOP command example in PHP
require 'vendor/autoload.php';
// Connect to Redis
$redisClient = new PredisClient([
'scheme' => 'tcp',
'host' => 'localhost',
'port' => 6379,
]);
/**
* Push elements and create list
*
* Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
* Result: (integer) 5
*/
$commandResult = $redisClient->rpush("bigboxlist", [
"Item A",
"Item B",
"Item C",
"Item D",
"Item E",
]);
echo 'Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: ' . $commandResult . "n";
/**
* Check item list
*
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "Item A"
* 2) "Item B"
* 3) "Item C"
* 4) "Item D"
* 5) "Item E"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Pop 1 item from HEAD
*
* Command: lpop bigboxlist
* Result: "Item A"
*/
$commandResult = $redisClient->lpop("bigboxlist");
echo "Command: lpop bigboxlist | Result: " . $commandResult . "n";
/**
* Pop 2 items from HEAD
*
* Command: lpop bigboxlist 2
* Result:
* 1) "Item B"
* 2) "Item C"
*/
$commandResult = $redisClient->lmpop(["bigboxlist"], "left", 2);
echo "Command: lpop bigboxlist 2 | Result: ";
print_r($commandResult);
/**
* Try to pass negative value for the count
* We get an error message
*
* Command: lpop bigboxlist -2
* Result: (error) ERR value is out of range, must be positive
*/
try {
$commandResult = $redisClient->lmpop(["bigboxlist"], "left", -2);
echo "Command: lpop bigboxlist -2 | Result: ";
print_r($commandResult);
} catch (Exception $e) {
echo "Command: lpop bigboxlist -2 | Error: " . $e->getMessage() . "n";
}
/**
* Try to pop 5 items from list
* The list has only 2 items
* 2 items are popped and command is successful
*
* Command: lpop bigboxlist 5
* Result:
* 1) "Item D"
* 2) "Item E"
*/
$commandResult = $redisClient->lmpop(["bigboxlist"], "left", 5);
echo "Command: lpop bigboxlist 5 | Result: ";
print_r($commandResult);
/**
* Check if list exits after all items are popped
* List does not exist any more
*
* Command: exists bigboxlist
* Result: (integer) 0
*/
$commandResult = $redisClient->exists("bigboxlist");
echo "Command: exists bigboxlist | Result: " . $commandResult . "n";
/**
* Try to pop from a non existing list
* returns (nil)
*
* Command: lpop bigboxlist
* Result: (nil)
*/
$commandResult = $redisClient->lpop("bigboxlist");
echo "Command: lpop bigboxlist | Result: " . $commandResult . "n";
/**
* Create an string value
*
* Command: set bigboxstr "my string value here"
* Result: OK
*/
$commandResult = $redisClient->set("bigboxstr", "my string value here");
echo "Command: set bigboxstr "my string value here" | Result: " . $commandResult . "n";
/**
* Try to apply LPOP on the string
* Returns an error message
*
* Command: lpop bigboxstr
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
$commandResult = $redisClient->lpop("bigboxstr");
echo "Command: lpop bigboxstr | Result: " . $commandResult . "n";
} catch (Exception $e) {
echo "Command: lpop bigboxstr | Error: " . $e->getMessage() . "n";
}
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => Item A
[1] => Item B
[2] => Item C
[3] => Item D
[4] => Item E
)
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: Array
(
[bigboxlist] => Array
(
[0] => Item B
[1] => Item C
)
)
Command: lpop bigboxlist -2 | Error: Wrong count argument value or position offset
Command: lpop bigboxlist 5 | Result: Array
(
[bigboxlist] => Array
(
[0] => Item D
[1] => Item E
)
)
Command: exists bigboxlist | Result: 0
Command: lpop bigboxlist | Result:
Command: set bigboxstr "my string value here" | Result: OK
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “lpop” of predis.
- Signature of the method is-
lpop(string $key): string|null - lpop method does not have the option for the count. If you want to use the count then use lmpop method.
# Redis LPOP command example in Python
import redis
import time
# Create Redis client
redisClient = redis.Redis(host='localhost', port=6379,
username='default', password='',
decode_responses=True)
# Push elements and create list
# Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
# Result: (integer) 5
commandResult = redisClient.rpush("bigboxlist",
"Item A",
"Item B",
"Item C",
"Item D",
"Item E",
)
print("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: {}".format(commandResult))
# Check item list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "Item A"
# 2) "Item B"
# 3) "Item C"
# 4) "Item D"
# 5) "Item E"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Pop 1 item from HEAD
# Command: lpop bigboxlist
# Result: "Item A"
commandResult = redisClient.lpop("bigboxlist")
print("Command: lpop bigboxlist | Result: {}".format(commandResult))
# Pop 2 items from HEAD
# Command: lpop bigboxlist 2
# Result:
# 1) "Item B"
# 2) "Item C"
commandResult = redisClient.lpop("bigboxlist", 2)
print("Command: lpop bigboxlist 2 | Result: {}".format(commandResult))
# Try to pass negative value for the count
# We get an error message
# Command: lpop bigboxlist -2
# Result: (error) ERR value is out of range, must be positive
try:
commandResult = redisClient.lpop("bigboxlist", -2)
print("Command: lpop bigboxlist -2 | Result: {}".format(commandResult))
except Exception as error:
print("Command: lpop bigboxlist -2 | Error: ", error)
# Pass Zero(0) as count
# Empty array is returned
#
# Command: lpop bigboxlist 0
# Result: (empty array)
commandResult = redisClient.lpop("bigboxlist", 0)
print("Command: lpop bigboxlist 0 | Result: {}".format(commandResult))
# Try to pop 5 items from list
# The list has only 2 items
# 2 items are popped and command is successful
# Command: lpop bigboxlist 5
# Result:
# 1) "Item D"
# 2) "Item E"
commandResult = redisClient.lpop("bigboxlist", 5)
print("Command: lpop bigboxlist 5 | Result: {}".format(commandResult))
# Check if list exits after all items are popped
# List does not exist any more
# Command: exists bigboxlist
# Result: (integer) 0
commandResult = redisClient.exists("bigboxlist")
print("Command: exists bigboxlist | Result: {}".format(commandResult))
# Try to pop from a non existing list
# returns (nil)
# Command: lpop bigboxlist
# Result: (nil)
commandResult = redisClient.lpop("bigboxlist")
print("Command: lpop bigboxlist | Result: {}".format(commandResult))
# Create an string value
# Command: set bigboxstr "my string value here"
# Result: OK
commandResult = redisClient.set("bigboxstr", "my string value here")
print("Command: set bigboxstr "my string value here" | Result: {}".format(commandResult))
# Try to apply LPOP on the string
# Returns an error message
# Command: lpop bigboxstr
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
try:
commandResult = redisClient.lpop("bigboxstr")
print("Command: lpop bigboxstr | Result: {}".format(commandResult))
except Exception as error:
print("Command: lpop bigboxstr | Error: ", error)
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: ['Item A', 'Item B', 'Item C', 'Item D', 'Item E']
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: ['Item B', 'Item C']
Command: lpop bigboxlist -2 | Error: value is out of range, must be positive
Command: lpop bigboxlist 0 | Result: []
Command: lpop bigboxlist 5 | Result: ['Item D', 'Item E']
Command: exists bigboxlist | Result: 0
Command: lpop bigboxlist | Result: None
Command: set bigboxstr "my string value here" | Result: True
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpop” from redis-py.
- Signature of the method is –
def lpop(self, name: str, count: Optional[int] = None,) -> Union[Awaitable[Union[str, List, None]], Union[str, List, None]]:
# Redis LPOP command example in Ruby
require 'redis'
redis = Redis.new(host: "localhost", port: 6379)
# Push elements and create list
# Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E"
# Result: (integer) 5
commandResult = redis.rpush("bigboxlist", [
"Item A",
"Item B",
"Item C",
"Item D",
"Item E",
])
print("Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: ", commandResult, "n")
# Check item list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "Item A"
# 2) "Item B"
# 3) "Item C"
# 4) "Item D"
# 5) "Item E"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Pop 1 item from HEAD
# Command: lpop bigboxlist
# Result: "Item A"
commandResult = redis.lpop("bigboxlist")
print("Command: lpop bigboxlist | Result: ", commandResult, "n")
# Pop 2 items from HEAD
# Command: lpop bigboxlist 2
# Result:
# 1) "Item B"
# 2) "Item C"
commandResult = redis.lpop("bigboxlist", 2)
print("Command: lpop bigboxlist 2 | Result: ", commandResult, "n")
# Try to pass negative value for the count
# We get an error message
# Command: lpop bigboxlist -2
# Result: (error) ERR value is out of range, must be positive
begin
commandResult = redis.lpop("bigboxlist", -2)
print("Command: lpop bigboxlist -2 | Result: ", commandResult, "n")
rescue => e
print("Command: lpop bigboxlist -2 | Error: ", e, "n")
end
# Pass Zero(0) as count
# Empty array is returned
#
# Command: lpop bigboxlist 0
# Result: (empty array)
commandResult = redis.lpop("bigboxlist", 0)
print("Command: lpop bigboxlist 0 | Result: ", commandResult, "n")
# Try to pop 5 items from list
# The list has only 2 items
# 2 items are popped and command is successful
# Command: lpop bigboxlist 5
# Result:
# 1) "Item D"
# 2) "Item E"
commandResult = redis.lpop("bigboxlist", 5)
print("Command: lpop bigboxlist 5 | Result: ", commandResult, "n")
# Check if list exits after all items are popped
# List does not exist any more
# Command: exists bigboxlist
# Result: (integer) 0
commandResult = redis.exists("bigboxlist")
print("Command: exists bigboxlist | Result: ", commandResult, "n")
# Try to pop from a non existing list
# returns (nil)
# Command: lpop bigboxlist
# Result: (nil)
commandResult = redis.lpop("bigboxlist")
print("Command: lpop bigboxlist | Result: ", commandResult, "n")
# Create an string value
# Command: set bigboxstr "my string value here"
# Result: OK
commandResult = redis.set("bigboxstr", "my string value here")
print("Command: set bigboxstr "my string value here" | Result: ", commandResult, "n")
# Try to apply LPOP on the string
# Returns an error message
# Command: lpop bigboxstr
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
begin
commandResult = redis.lpop("bigboxstr")
print("Command: lpop bigboxstr | Result: ", commandResult, "n")
rescue => e
print("Command: lpop bigboxstr | Error: ", e, "n")
end
Output:
Command: rpush bigboxlist "Item A" "Item B" "Item C" "Item D" "Item E" | Result: 5
Command: lrange bigboxlist 0 -1 | Result: ["Item A", "Item B", "Item C", "Item D", "Item E"]
Command: lpop bigboxlist | Result: Item A
Command: lpop bigboxlist 2 | Result: ["Item B", "Item C"]
Command: lpop bigboxlist -2 | Error: ERR value is out of range, must be positive
Command: lpop bigboxlist 0 | Result: []
Command: lpop bigboxlist 5 | Result: ["Item D", "Item E"]
Command: exists bigboxlist | Result: 0
Command: lpop bigboxlist | Result:
Command: set bigboxstr "my string value here" | Result: OK
Command: lpop bigboxstr | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “lpop” from the redis-rb.
- Signature of the method is-
# @param [String] key
# @param [Integer] count number of elements to remove
# @return [nil, String, Array<String>] the values of the first elements
def lpop(key, count = nil)
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LPUSH | ![]() |
RPUSH | ![]() |
LRANGE | ![]() |