Summary
Command Name | LTRIM |
Usage | Trim list to start and stop indexes |
Group | ![]() |
ACL Category | @write @list @slow |
Time Complexity | O(N) |
Flag | WRITE |
Arity | 4 |
Notes
- In the time complexity, N is the number of removed items.
Signature
LTRIM <key> <start> <stop>
Usage
Trim a Redis list, and remove items from the list from one or both end(s). We provide a starting and ending index for the trim and the items outside that window are removed.
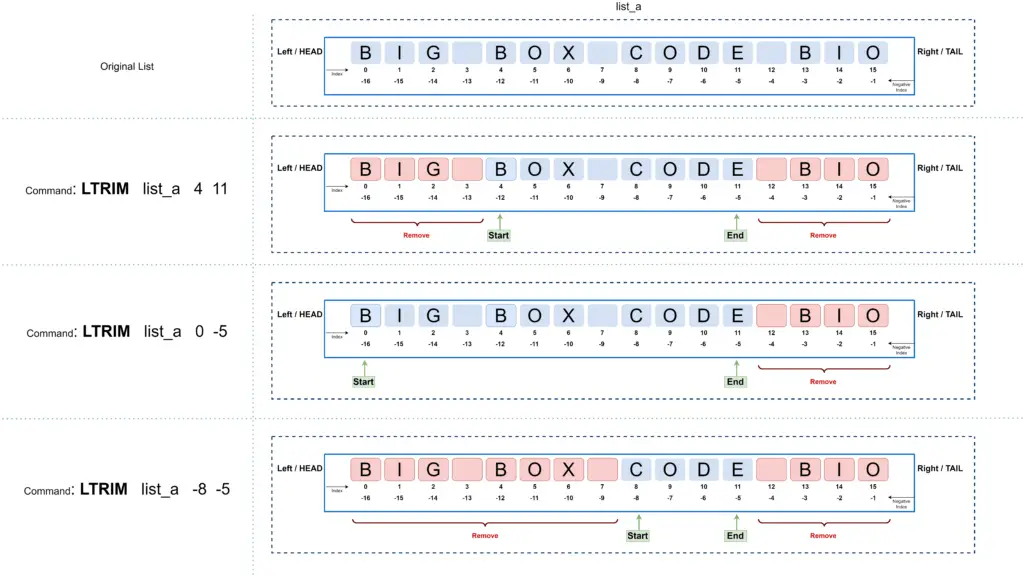
Notes
- If all items are removed while trimming, then the list is deleted.
- Indexes are Zero(0) based.
- Both positive and negative indexing works for this command.
Arguments
Parameter | Description | Name | Type |
---|---|---|---|
<key> | Key name of the list | key | key |
<start> | Index to start trimming | start | integer |
<stop> | Index to stop trimming | stop | integer |
Return Value
Return value | Case for the return value | Type |
---|---|---|
OK | If the operation was successful, and the list exists | const |
error | If the command applied on data other than a list | error |
Notes
- If key is not of type list, the following error message is returned-
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Examples
Here are a few examples of the LTRIM command usage-
# Redis LTRIM command examples
# Push items and create list
127.0.0.1:6379> rpush bigboxlist B I G B O X C O D E B I O
(integer) 13
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "B"
2) "I"
3) "G"
4) "B"
5) "O"
6) "X"
7) "C"
8) "O"
9) "D"
10) "E"
11) "B"
12) "I"
13) "O"
# Trim items outside of index 3 to the end
127.0.0.1:6379> ltrim bigboxlist 3 -1
OK
# Check list. Initial 3 items are deleted
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "B"
2) "O"
3) "X"
4) "C"
5) "O"
6) "D"
7) "E"
8) "B"
9) "I"
10) "O"
# Keep items from index 0 to 6 and delete others
127.0.0.1:6379> ltrim bigboxlist 0 6
OK
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "B"
2) "O"
3) "X"
4) "C"
5) "O"
6) "D"
7) "E"
# Try to trim by keeping items from index 3 to 100
# Max index in existing list is 6. So it will use 6 instead of 100
127.0.0.1:6379> ltrim bigboxlist 3 100
OK
# Check list
127.0.0.1:6379> lrange bigboxlist 0 -1
1) "C"
2) "O"
3) "D"
4) "E"
# Provide ltrim indexes where start index is larger
# This will empty the list
127.0.0.1:6379> ltrim bigboxlist 2 1
OK
# Check list, the list is empty now
127.0.0.1:6379> lrange bigboxlist 0 -1
(empty array)
# Try to trim a list that does not exist
# It will return OK
127.0.0.1:6379> ltrim nonexistinglist 0 1
OK
# Set a string
127.0.0.1:6379> set bigboxstr "Some string for test"
OK
# Try to use LTRIM on a string
# we get an error
127.0.0.1:6379> ltrim bigboxstr 0 1
(error) WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- If the <start> or <stop> index is out of range then, the command will adjust to the max/min possible index.
- If <start> is larger than <stop> then the LTRIM command will return an empty list.
- If we try to use LTRIM on a list that does not exist, then it will return “OK”. Nothing else will happen.
Code Implementations
Here are the usage examples of the Redis LTRIM command in different programming languages.
// Redis LTRIM command example in Golang
package main
import (
"context"
"fmt"
"github.com/redis/go-redis/v9"
)
var rdb *redis.Client
var ctx context.Context
func init() {
rdb = redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Username: "default",
Password: "",
DB: 0,
})
ctx = context.Background()
}
func main() {
// Push items and create list
// Command: rpush bigboxlist B I G B O X C O D E B I O
// Result: (integer) 13
rpushResult, err := rdb.RPush(ctx, "bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O").Result()
if err != nil {
fmt.Println("Command: rpush bigboxlist B I G B O X C O D E B I O | Error: " + err.Error())
}
fmt.Println("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: ", rpushResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
lrangeResult, err := rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Trim items outside of index 3 to the end
// Command: ltrim bigboxlist 3 -1
// Result: OK
ltrimResult, err := rdb.LTrim(ctx, "bigboxlist", 3, -1).Result()
if err != nil {
fmt.Println("Command: ltrim bigboxlist 3 -1 | Error: " + err.Error())
}
fmt.Println("Command: ltrim bigboxlist 3 -1 | Result: " + ltrimResult)
// Check list. Initial 3 items are deleted
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Keep items from index 0 to 6 and delete others
// Command: ltrim bigboxlist 0 6
// Result: OK
ltrimResult, err = rdb.LTrim(ctx, "bigboxlist", 0, 6).Result()
if err != nil {
fmt.Println("Command: ltrim bigboxlist 0 6 | Error: " + err.Error())
}
fmt.Println("Command: ltrim bigboxlist 0 6 | Result: " + ltrimResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Try to trim by keeping items from index 3 to 100
// Max index in existing list is 6. So it will use 6 instead of 100
// Command: ltrim bigboxlist 3 100
// Result: OK
ltrimResult, err = rdb.LTrim(ctx, "bigboxlist", 3, 100).Result()
if err != nil {
fmt.Println("Command: ltrim bigboxlist 3 100 | Error: " + err.Error())
}
fmt.Println("Command: ltrim bigboxlist 3 100 | Result: " + ltrimResult)
// Check list
// Command: lrange bigboxlist 0 -1
// Result:
// 1) "C" 2) "O" 3) "D" 4) "E"
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Provide ltrim indexes where start index is larger
// This will empty the list
// Command: ltrim bigboxlist 2 1
// Result: OK
ltrimResult, err = rdb.LTrim(ctx, "bigboxlist", 2, 1).Result()
if err != nil {
fmt.Println("Command: ltrim bigboxlist 2 1 | Error: " + err.Error())
}
fmt.Println("Command: ltrim bigboxlist 2 1 | Result: " + ltrimResult)
// Check list, the list is empty now
// Command: lrange bigboxlist 0 -1
// Result: (empty array)
lrangeResult, err = rdb.LRange(ctx, "bigboxlist", 0, -1).Result()
if err != nil {
fmt.Println("Command: lrange bigboxlist 0 -1 | Error: " + err.Error())
}
fmt.Println("Command: lrange bigboxlist 0 -1 | Result: ", lrangeResult)
// Try to trim a list that does not exist
// It will return OK
// Command: ltrim nonexistinglist 0 1
// Result: OK
ltrimResult, err = rdb.LTrim(ctx, "bigboxlist", 0, 1).Result()
if err != nil {
fmt.Println("Command: ltrim nonexistinglist 0 1 | Error: " + err.Error())
}
fmt.Println("Command: ltrim nonexistinglist 0 1 | Result: " + ltrimResult)
// Set a string
// Command: set bigboxstr "Some string for test"
// Result: OK
setResult, err := rdb.Set(ctx, "bigboxstr", "Some string for test", 0).Result()
if err != nil {
fmt.Println("Command: set bigboxstr "Some string for test" | Error: " + err.Error())
}
fmt.Println("Command: set bigboxstr "Some string for test" | Result: " + setResult)
// Try to use LTRIM on a string
// we get an error
// Command: ltrim bigboxstr 0 1
// Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
ltrimResult, err = rdb.LTrim(ctx, "bigboxstr", 0, 1).Result()
if err != nil {
fmt.Println("Command: ltrim bigboxstr 0 1 | Error: " + err.Error())
}
fmt.Println("Command: ltrim bigboxstr 0 1 | Result: " + ltrimResult)
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [B I G B O X C O D E B I O]
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [B O X C O D E B I O]
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [B O X C O D E]
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [C O D E]
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Command: ltrim bigboxstr 0 1 | Result:
Notes
- Use “LTrim” method from redis-go module.
- Signature of the method is-
func (c cmdable) LTrim(ctx context.Context, key string, start, stop int64) *StatusCmd
// Redis LTRIM command example in JavaScript(NodeJS)
import { createClient } from "redis";
// Create redis client
const redisClient = createClient({
url: "redis://default:@localhost:6379",
});
await redisClient.on("error", (err) =>
console.log("Error while connecting to Redis", err)
);
// Connect Redis client
await redisClient.connect();
/**
* Push items and create list
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
let commandResult = await redisClient.rPush("bigboxlist", [
"B",
"I",
"G",
"B",
"O",
"X",
"C",
"O",
"D",
"E",
"B",
"I",
"O",
]);
console.log(
"Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " +
commandResult
);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Trim items outside of index 3 to the end
* Command: ltrim bigboxlist 3 -1
* Result: OK
*/
commandResult = await redisClient.lTrim("bigboxlist", 3, -1);
console.log("Command: ltrim bigboxlist 3 -1 | Result: " + commandResult);
/**
* Check list. Initial 3 items are deleted
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Keep items from index 0 to 6 and delete others
* Command: ltrim bigboxlist 0 6
* Result: OK
*/
commandResult = await redisClient.lTrim("bigboxlist", 0, 6);
console.log("Command: ltrim bigboxlist 0 6 | Result: " + commandResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Try to trim by keeping items from index 3 to 100
* Max index in existing list is 6. So it will use 6 instead of 100
* Command: ltrim bigboxlist 3 100
* Result: OK
*/
commandResult = await redisClient.lTrim("bigboxlist", 3, 100);
console.log("Command: ltrim bigboxlist 3 100 | Result: " + commandResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "C" 2) "O" 3) "D" 4) "E"
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Provide ltrim indexes where start index is larger
* This will empty the list
* Command: ltrim bigboxlist 2 1
* Result: OK
*/
commandResult = await redisClient.lTrim("bigboxlist", 2, 1);
console.log("Command: ltrim bigboxlist 2 1 | Result: " + commandResult);
/**
* Check list, the list is empty now
* Command: lrange bigboxlist 0 -1
* Result: (empty array)
*/
commandResult = await redisClient.lRange("bigboxlist", 0, -1);
console.log("Command: lrange bigboxlist 0 -1 | Result: ", commandResult);
/**
* Try to trim a list that does not exist
* It will return OK
* Command: ltrim nonexistinglist 0 1
* Result: OK
*/
commandResult = await redisClient.lTrim("bigboxlist", 0, 1);
console.log("Command: ltrim nonexistinglist 0 1 | Result: " + commandResult);
/**
* Set a string
* Command: set bigboxstr "Some string for test"
* Result: OK
*/
commandResult = await redisClient.set("bigboxstr", "Some string for test");
console.log(
'Command: set bigboxstr "Some string for test" | Result: ' + commandResult
);
/**
* Try to use LTRIM on a string
* we get an error
* Command: ltrim bigboxstr 0 1
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
commandResult = await redisClient.lTrim("bigboxstr", 0, 1);
console.log("Command: ltrim bigboxstr 0 1 | Result: " + commandResult);
} catch (err) {
console.log("Command: ltrim bigboxstr 0 1 | Error: ", err);
}
process.exit(0);
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [
'B', 'I', 'G', 'B',
'O', 'X', 'C', 'O',
'D', 'E', 'B', 'I',
'O'
]
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [
'B', 'O', 'X', 'C',
'O', 'D', 'E', 'B',
'I', 'O'
]
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [
'B', 'O', 'X',
'C', 'O', 'D',
'E'
]
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [ 'C', 'O', 'D', 'E' ]
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: [ErrorReply: WRONGTYPE Operation against a key holding the wrong kind of value]
Notes
- Use the function “lTrim” from the package node-redis.
- Signature of the method is-
lTrim(key: RedisCommandArgument, start: number, stop: number)
// Redis LTRIM Command example in Java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import java.util.List;
public class Ltrim {
public static void main(String[] args) {
// Create connection pool
JedisPool jedisPool = new JedisPool("localhost", 6379);
try (Jedis jedis = jedisPool.getResource()) {
/**
* Push items and create list
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
long rpushResult = jedis.rpush("bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O");
System.out.println("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " + rpushResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
*/
List<String> lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Trim items outside of index 3 to the end
* Command: ltrim bigboxlist 3 -1
* Result: OK
*/
String ltrimResult = jedis.ltrim("bigboxlist", 3, -1);
System.out.println("Command: ltrim bigboxlist 3 -1 | Result: " + ltrimResult);
/**
* Check list. Initial 3 items are deleted
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Keep items from index 0 to 6 and delete others
* Command: ltrim bigboxlist 0 6
* Result: OK
*/
ltrimResult = jedis.ltrim("bigboxlist", 0, 6);
System.out.println("Command: ltrim bigboxlist 0 6 | Result: " + ltrimResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Try to trim by keeping items from index 3 to 100
* Max index in existing list is 6. So it will use 6 instead of 100
* Command: ltrim bigboxlist 3 100
* Result: OK
*/
ltrimResult = jedis.ltrim("bigboxlist", 3, 100);
System.out.println("Command: ltrim bigboxlist 3 100 | Result: " + ltrimResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "C" 2) "O" 3) "D" 4) "E"
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Provide ltrim indexes where start index is larger
* This will empty the list
* Command: ltrim bigboxlist 2 1
* Result: OK
*/
ltrimResult = jedis.ltrim("bigboxlist", 2, 1);
System.out.println("Command: ltrim bigboxlist 2 1 | Result: " + ltrimResult);
/**
* Check list, the list is empty now
* Command: lrange bigboxlist 0 -1
* Result: (empty array)
*/
lrangeResult = jedis.lrange("bigboxlist", 0, -1);
System.out.println("Command: lrange bigboxlist 0 -1 | Result: " + lrangeResult.toString());
/**
* Try to trim a list that does not exist
* It will return OK
* Command: ltrim nonexistinglist 0 1
* Result: OK
*/
ltrimResult = jedis.ltrim("bigboxlist", 0, 1);
System.out.println("Command: ltrim nonexistinglist 0 1 | Result: " + ltrimResult);
/**
* Set a string
* Command: set bigboxstr "Some string for test"
* Result: OK
*/
String setResult = jedis.set("bigboxstr", "Some string for test");
System.out.println("Command: set bigboxstr "Some string for test" | Result: " + setResult);
/**
* Try to use LTRIM on a string
* we get an error
* Command: ltrim bigboxstr 0 1
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
ltrimResult = jedis.ltrim("bigboxstr", 0, 1);
System.out.println("Command: ltrim bigboxstr 0 1 | Result: " + ltrimResult);
} catch(Exception e) {
System.out.println("Command: ltrim bigboxstr 0 1 | Error: " + e.getMessage());
}
}
jedisPool.close();
}
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: [B, I, G, B, O, X, C, O, D, E, B, I, O]
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [B, O, X, C, O, D, E, B, I, O]
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [B, O, X, C, O, D, E]
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: [C, O, D, E]
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “ltrim” from Jedis package.
- Signature of the method is-
public String ltrim(final String key, final long start, final long stop)
// Redis LTRIM command examples in C#
using StackExchange.Redis;
namespace Ltrim
{
internal class Program
{
static void Main(string[] args)
{
ConnectionMultiplexer redis = ConnectionMultiplexer.Connect("localhost");
IDatabase rdb = redis.GetDatabase();
/**
* Push items and create list
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
long rpushResult = rdb.ListRightPush("bigboxlist", new RedisValue[] { "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O" });
Console.WriteLine("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " + rpushResult);
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
*/
RedisValue[] lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Trim items outside of index 3 to the end
* Command: ltrim bigboxlist 3 -1
* Result: OK
*/
rdb.ListTrim("bigboxlist", 3, -1);
Console.WriteLine("Command: ltrim bigboxlist 3 -1");
/**
* Check list. Initial 3 items are deleted
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Keep items from index 0 to 6 and delete others
* Command: ltrim bigboxlist 0 6
* Result: OK
*/
rdb.ListTrim("bigboxlist", 0, 6);
Console.WriteLine("Command: ltrim bigboxlist 0 6");
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Try to trim by keeping items from index 3 to 100
* Max index in existing list is 6. So it will use 6 instead of 100
* Command: ltrim bigboxlist 3 100
* Result: OK
*/
rdb.ListTrim("bigboxlist", 3, 100);
Console.WriteLine("Command: ltrim bigboxlist 3 100");
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "C" 2) "O" 3) "D" 4) "E"
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Provide ltrim indexes where start index is larger
* This will empty the list
* Command: ltrim bigboxlist 2 1
* Result: OK
*/
rdb.ListTrim("bigboxlist", 2, 1);
Console.WriteLine("Command: ltrim bigboxlist 2 1");
/**
* Check list, the list is empty now
* Command: lrange bigboxlist 0 -1
* Result: (empty array)
*/
lrangeResult = rdb.ListRange("bigboxlist", 0, -1);
Console.WriteLine("Command: lrange bigboxlist 0 -1 | Result: " + string.Join(", ", lrangeResult));
/**
* Try to trim a list that does not exist
* It will return OK
* Command: ltrim nonexistinglist 0 1
* Result: OK
*/
rdb.ListTrim("bigboxlist", 0, 1);
Console.WriteLine("Command: ltrim nonexistinglist 0 1");
/**
* Set a string
* Command: set bigboxstr "Some string for test"
* Result: OK
*/
bool setResult = rdb.StringSet("bigboxstr", "Some string for test");
Console.WriteLine("Command: set bigboxstr "Some string for test" | Result: " + setResult);
/**
* Try to use LTRIM on a string
* we get an error
* Command: ltrim bigboxstr 0 1
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try
{
rdb.ListTrim("bigboxstr", 0, 1);
Console.WriteLine("Command: ltrim bigboxstr 0 1");
}
catch (Exception e)
{
Console.WriteLine("Command: ltrim bigboxstr 0 1 | Error: " + e.Message);
}
}
}
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: B, I, G, B, O, X, C, O, D, E, B, I, O
Command: ltrim bigboxlist 3 -1
Command: lrange bigboxlist 0 -1 | Result: B, O, X, C, O, D, E, B, I, O
Command: ltrim bigboxlist 0 6
Command: lrange bigboxlist 0 -1 | Result: B, O, X, C, O, D, E
Command: ltrim bigboxlist 3 100
Command: lrange bigboxlist 0 -1 | Result: C, O, D, E
Command: ltrim bigboxlist 2 1
Command: lrange bigboxlist 0 -1 | Result:
Command: ltrim nonexistinglist 0 1
Command: set bigboxstr "Some string for test" | Result: True
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ListTrim” from StackExchange.Redis.
- Signature of the method is-
void ListTrim(RedisKey key, long start, long stop, CommandFlags flags = CommandFlags.None)
<?php
// Redis LTRIM command example in PHP
require 'vendor/autoload.php';
// Connect to Redis
$redisClient = new PredisClient([
'scheme' => 'tcp',
'host' => 'localhost',
'port' => 6379,
]);
/**
* Push items and create list
* Command: rpush bigboxlist B I G B O X C O D E B I O
* Result: (integer) 13
*/
$commandResult = $redisClient->rpush("bigboxlist", ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]);
echo "Command: rpush bigboxlist B I G B O X C O D E B I O | Result: " . $commandResult . "n";
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Trim items outside of index 3 to the end
* Command: ltrim bigboxlist 3 -1
* Result: OK
*/
$commandResult = $redisClient->ltrim("bigboxlist", 3, -1);
echo "Command: ltrim bigboxlist 3 -1 | Result: " . $commandResult . "n";
/**
* Check list. Initial 3 items are deleted
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Keep items from index 0 to 6 and delete others
* Command: ltrim bigboxlist 0 6
* Result: OK
*/
$commandResult = $redisClient->ltrim("bigboxlist", 0, 6);
echo "Command: ltrim bigboxlist 0 6 | Result: " . $commandResult . "n";
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Try to trim by keeping items from index 3 to 100
* Max index in existing list is 6. So it will use 6 instead of 100
* Command: ltrim bigboxlist 3 100
* Result: OK
*/
$commandResult = $redisClient->ltrim("bigboxlist", 3, 100);
echo "Command: ltrim bigboxlist 3 100 | Result: " . $commandResult . "n";
/**
* Check list
* Command: lrange bigboxlist 0 -1
* Result:
* 1) "C" 2) "O" 3) "D" 4) "E"
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Provide ltrim indexes where start index is larger
* This will empty the list
* Command: ltrim bigboxlist 2 1
* Result: OK
*/
$commandResult = $redisClient->ltrim("bigboxlist", 2, 1);
echo "Command: ltrim bigboxlist 2 1 | Result: " . $commandResult . "n";
/**
* Check list, the list is empty now
* Command: lrange bigboxlist 0 -1
* Result: (empty array)
*/
$commandResult = $redisClient->lrange("bigboxlist", 0, -1);
echo "Command: lrange bigboxlist 0 -1 | Result: ";
print_r($commandResult);
/**
* Try to trim a list that does not exist
* It will return OK
* Command: ltrim nonexistinglist 0 1
* Result: OK
*/
$commandResult = $redisClient->ltrim("bigboxlist", 0, 1);
echo "Command: ltrim nonexistinglist 0 1 | Result: " . $commandResult . "n";
/**
* Set a string
* Command: set bigboxstr "Some string for test"
* Result: OK
*/
$commandResult = $redisClient->set("bigboxstr", "Some string for test");
echo "Command: set bigboxstr "Some string for test" | Result: " . $commandResult . "n";
/**
* Try to use LTRIM on a string
* we get an error
* Command: ltrim bigboxstr 0 1
* Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
*/
try {
$commandResult = $redisClient->ltrim("bigboxstr", 0, 1);
echo "Command: ltrim bigboxstr 0 1 | Result: " . $commandResult . "n";
} catch(Exception $e) {
echo "Command: ltrim bigboxstr 0 1 | Error: " . $e->getMessage() . "n";
}
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => I
[2] => G
[3] => B
[4] => O
[5] => X
[6] => C
[7] => O
[8] => D
[9] => E
[10] => B
[11] => I
[12] => O
)
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => O
[2] => X
[3] => C
[4] => O
[5] => D
[6] => E
[7] => B
[8] => I
[9] => O
)
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => B
[1] => O
[2] => X
[3] => C
[4] => O
[5] => D
[6] => E
)
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
[0] => C
[1] => O
[2] => D
[3] => E
)
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: Array
(
)
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use the method “ltrim” of predis.
- Signature of the method is-
ltrim(string $key, int $start, int $stop): mixed
# Redis LTRIM command example in Python
import redis
import time
# Create Redis client
redisClient = redis.Redis(host='localhost', port=6379,
username='default', password='',
decode_responses=True)
# Push items and create list
# Command: rpush bigboxlist B I G B O X C O D E B I O
# Result: (integer) 13
commandResult = redisClient.rpush("bigboxlist", "B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O")
print("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Trim items outside of index 3 to the end
# Command: ltrim bigboxlist 3 -1
# Result: OK
commandResult = redisClient.ltrim("bigboxlist", 3, -1)
print("Command: ltrim bigboxlist 3 -1 | Result: {}".format(commandResult))
# Check list. Initial 3 items are deleted
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Keep items from index 0 to 6 and delete others
# Command: ltrim bigboxlist 0 6
# Result: OK
commandResult = redisClient.ltrim("bigboxlist", 0, 6)
print("Command: ltrim bigboxlist 0 6 | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Try to trim by keeping items from index 3 to 100
# Max index in existing list is 6. So it will use 6 instead of 100
# Command: ltrim bigboxlist 3 100
# Result: OK
commandResult = redisClient.ltrim("bigboxlist", 3, 100)
print("Command: ltrim bigboxlist 3 100 | Result: {}".format(commandResult))
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "C" 2) "O" 3) "D" 4) "E"
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Provide ltrim indexes where start index is larger
# This will empty the list
# Command: ltrim bigboxlist 2 1
# Result: OK
commandResult = redisClient.ltrim("bigboxlist", 2, 1)
print("Command: ltrim bigboxlist 2 1 | Result: {}".format(commandResult))
# Check list, the list is empty now
# Command: lrange bigboxlist 0 -1
# Result: (empty array)
commandResult = redisClient.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: {}".format(commandResult))
# Try to trim a list that does not exist
# It will return OK
# Command: ltrim nonexistinglist 0 1
# Result: OK
commandResult = redisClient.ltrim("bigboxlist", 0, 1)
print("Command: ltrim nonexistinglist 0 1 | Result: {}".format(commandResult))
# Set a string
# Command: set bigboxstr "Some string for test"
# Result: OK
commandResult = redisClient.set("bigboxstr", "Some string for test")
print("Command: set bigboxstr "Some string for test" | Result: {}".format(commandResult))
# Try to use LTRIM on a string
# we get an error
# Command: ltrim bigboxstr 0 1
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
try:
commandResult = redisClient.ltrim("bigboxstr", 0, 1)
print("Command: ltrim bigboxstr 0 1 | Result: {}".format(commandResult))
except Exception as error:
print("Command: ltrim bigboxstr 0 1 | Error: ", error)
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ['B', 'I', 'G', 'B', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: ltrim bigboxlist 3 -1 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['B', 'O', 'X', 'C', 'O', 'D', 'E', 'B', 'I', 'O']
Command: ltrim bigboxlist 0 6 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['B', 'O', 'X', 'C', 'O', 'D', 'E']
Command: ltrim bigboxlist 3 100 | Result: True
Command: lrange bigboxlist 0 -1 | Result: ['C', 'O', 'D', 'E']
Command: ltrim bigboxlist 2 1 | Result: True
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: True
Command: set bigboxstr "Some string for test" | Result: True
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “ltrim” from redis-py.
- Signature of the method is –
def ltrim(self, name: str, start: int, end: int) -> Union[Awaitable[str], str]
# Redis LTRIM command example in Ruby
require 'redis'
redis = Redis.new(host: "localhost", port: 6379)
# Push items and create list
# Command: rpush bigboxlist B I G B O X C O D E B I O
# Result: (integer) 13
commandResult = redis.rpush("bigboxlist", ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"])
print("Command: rpush bigboxlist B I G B O X C O D E B I O | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "I" 3) "G" 4) "B" 5) "O" 6) "X" 7) "C" 8) "O" 9) "D" 10) "E" 11) "B" 12) "I" 13) "O"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Trim items outside of index 3 to the end
# Command: ltrim bigboxlist 3 -1
# Result: OK
commandResult = redis.ltrim("bigboxlist", 3, -1)
print("Command: ltrim bigboxlist 3 -1 | Result: ", commandResult, "n")
# Check list. Initial 3 items are deleted
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E" 8) "B" 9) "I" 10) "O"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Keep items from index 0 to 6 and delete others
# Command: ltrim bigboxlist 0 6
# Result: OK
commandResult = redis.ltrim("bigboxlist", 0, 6)
print("Command: ltrim bigboxlist 0 6 | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "B" 2) "O" 3) "X" 4) "C" 5) "O" 6) "D" 7) "E"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Try to trim by keeping items from index 3 to 100
# Max index in existing list is 6. So it will use 6 instead of 100
# Command: ltrim bigboxlist 3 100
# Result: OK
commandResult = redis.ltrim("bigboxlist", 3, 100)
print("Command: ltrim bigboxlist 3 100 | Result: ", commandResult, "n")
# Check list
# Command: lrange bigboxlist 0 -1
# Result:
# 1) "C" 2) "O" 3) "D" 4) "E"
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Provide ltrim indexes where start index is larger
# This will empty the list
# Command: ltrim bigboxlist 2 1
# Result: OK
commandResult = redis.ltrim("bigboxlist", 2, 1)
print("Command: ltrim bigboxlist 2 1 | Result: ", commandResult, "n")
# Check list, the list is empty now
# Command: lrange bigboxlist 0 -1
# Result: (empty array)
commandResult = redis.lrange("bigboxlist", 0, -1)
print("Command: lrange bigboxlist 0 -1 | Result: ", commandResult, "n")
# Try to trim a list that does not exist
# It will return OK
# Command: ltrim nonexistinglist 0 1
# Result: OK
commandResult = redis.ltrim("bigboxlist", 0, 1)
print("Command: ltrim nonexistinglist 0 1 | Result: ", commandResult, "n")
# Set a string
# Command: set bigboxstr "Some string for test"
# Result: OK
commandResult = redis.set("bigboxstr", "Some string for test")
print("Command: set bigboxstr "Some string for test" | Result: ", commandResult, "n")
# Try to use LTRIM on a string
# we get an error
# Command: ltrim bigboxstr 0 1
# Result: (error) WRONGTYPE Operation against a key holding the wrong kind of value
begin
commandResult = redis.ltrim("bigboxstr", 0, 1)
print("Command: ltrim bigboxstr 0 1 | Result: ", commandResult, "n")
rescue => e
print("Command: ltrim bigboxstr 0 1 | Error: ", e, "n")
end
Output:
Command: rpush bigboxlist B I G B O X C O D E B I O | Result: 13
Command: lrange bigboxlist 0 -1 | Result: ["B", "I", "G", "B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: ltrim bigboxlist 3 -1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["B", "O", "X", "C", "O", "D", "E", "B", "I", "O"]
Command: ltrim bigboxlist 0 6 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["B", "O", "X", "C", "O", "D", "E"]
Command: ltrim bigboxlist 3 100 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: ["C", "O", "D", "E"]
Command: ltrim bigboxlist 2 1 | Result: OK
Command: lrange bigboxlist 0 -1 | Result: []
Command: ltrim nonexistinglist 0 1 | Result: OK
Command: set bigboxstr "Some string for test" | Result: OK
Command: ltrim bigboxstr 0 1 | Error: WRONGTYPE Operation against a key holding the wrong kind of value
Notes
- Use method “ltrim” from the redis-rb.
- Signature of the method is-
# @param [String] key
# @param [Integer] start start index
# @param [Integer] stop stop index
# @return [String] `OK`
def ltrim(key, start, stop)
Source Code
Use the following links to get the source code used in this article-
Source Code of | Source Code Link |
---|---|
Command Examples | ![]() |
Golang Implementation | ![]() |
NodeJS Implementation | ![]() |
Java Implementation | ![]() |
C# Implementation | ![]() |
PHP Implementation | ![]() |
Python Implementation | ![]() |
Ruby Implementation | ![]() |
Related Commands
Command | Details |
---|---|
LPUSH | ![]() |
LRANGE | ![]() |