Command pattern is used to encapsulate/wrap a command in an object so that the object can be sent as a parameter and can be used in other places.
This article demonstrates Command pattern implementations in Java. Check the following examples.
Examples
Here are a few examples of command pattern in Java-
Example #1: UI Elements
Let’s take the example of printing UI elements. Let’s take a look at the class diagram first.
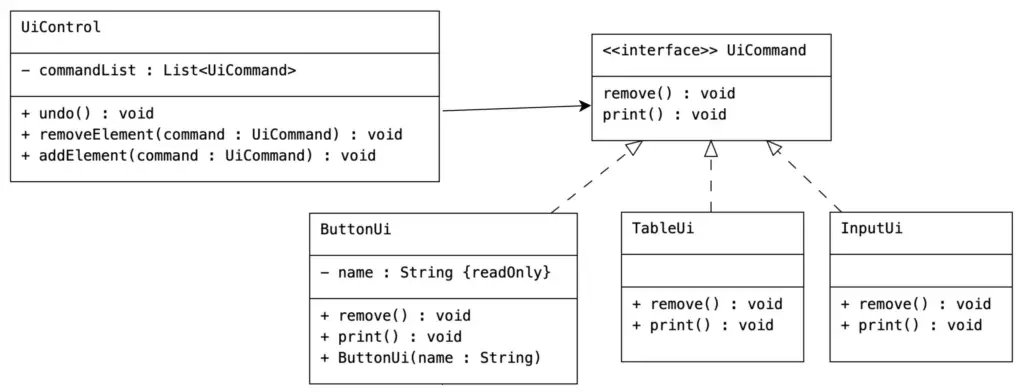
Command Interface
// UiCommand.java
package com.bigboxcode.designpattern.command.uielement;
public interface UiCommand {
void print();
void remove();
}
Button Concrete Class
// ButtonUi.java
package com.bigboxcode.designpattern.command.uielement;
public class ButtonUi implements UiCommand {
private final String name;
public ButtonUi(String name) {
this.name = name;
}
@Override
public void print() {
System.out.println("Printing " + this.name + " Button");
}
@Override
public void remove() {
System.out.println("Removing " + this.name + " Button");
}
}
Input Element Concrete Class
// UiCommand.java
package com.bigboxcode.designpattern.command.uielement;
public class InputUi implements UiCommand {
@Override
public void print() {
System.out.println("Printing Input");
}
@Override
public void remove() {
System.out.println("Removing Input");
}
}
Table Element Concrete Class
// TableUi.java
package com.bigboxcode.designpattern.command.uielement;
public class TableUi implements UiCommand {
@Override
public void print() {
System.out.println("Printing Table");
}
@Override
public void remove() {
System.out.println("Removing Table");
}
}
UI Element Control Class
// UiControl.java
package com.bigboxcode.designpattern.command.uielement;
import java.util.ArrayList;
import java.util.List;
public class UiControl {
private List<UiCommand> commandList = new ArrayList<>();
public void addElement(UiCommand command) {
// Execute command
command.print();
// Store command in list to have a history
commandList.add(command);
}
public void removeElement(UiCommand command) {
// Remove element
command.remove();
// Store command in list to have a history
commandList.remove(command);
}
public void undo() {
UiCommand lastCommand = commandList.get(commandList.size() - 1);
removeElement(lastCommand);
}
}
Demo
// Demo.java
package com.bigboxcode.designpattern.command.uielement;
public class Demo {
public static void main(String[] args) {
UiControl uiControl = new UiControl();
UiCommand inputUi = new InputUi();
UiCommand tableUi = new TableUi();
UiCommand buttonUi = new ButtonUi("Submit");
uiControl.addElement(inputUi);
uiControl.addElement(tableUi);
uiControl.addElement(buttonUi);
// Remove specific element
uiControl.removeElement(tableUi);
// Add some new elements
uiControl.addElement(new ButtonUi("Cancel"));
uiControl.addElement(new TableUi());
uiControl.addElement(new InputUi());
uiControl.addElement(new ButtonUi("Wrong button"));
// Undo last two command
uiControl.undo();
uiControl.undo();
}
}
Output
Printing Input
Printing Table
Printing Submit Button
Removing Table
Printing Cancel Button
Printing Table
Printing Input
Printing Wrong button Button
Removing Wrong button Button
Removing Input
Source Code
Use the following link to get the source code:
Example | Source Code Link |
---|---|
Example #1: UI Elements | ![]() |
Other Code Implementations
Use the following links to check Command pattern implementation in other programming languages.